This code creates a Sparkle Motion effect using SVG and Vanilla JS. It generates colorful sparks on user interaction. The sparks follow mouse clicks, movements, or touch gestures. It adds visual appeal to web elements.
You can use this code on your website to add interactive visual effects. It enhances user experience by adding sparkle animations to elements. It’s versatile, and suitable for various web projects, from personal blogs to business websites.
How to Create Sparkle Motion Effect Using SVG and Vanilla JS
1. Begin by adding the necessary HTML structure to your document. Insert an SVG element with an ID to serve as the container for the sparkle animations. You can customize the viewBox attribute to adjust the SVG’s dimensions.
- <svg id="motionSimulation" viewBox="0 0 200 100" preserveAspectRatio="none">
- <circle id="getMe" r="0" fill="none">
- <animateMotion dur="10s" repeatCount="indefinite" path="M20,50 C20,-50 180,150 180,50 C180-50 20,150 20,50 z" />
- </circle>
- </svg>
2. Apply CSS styles to position the SVG element and ensure it covers the desired area of your webpage. Utilize CSS properties like position, top, left, and transform to achieve the desired positioning for the sparkle effect.
- body{
- background: #111;
- overflow: hidden;
- min-height: 720px;
- }
- #motionSimulation {
- position: absolute;
- top: 50%;
- left: 50%;
- transform: translate(-50%, -50%);
- width: 100%;
- height: 100%;
- }
3. Implement the JavaScript code provided to enable the sparkle animation. This code handles user interactions such as mouse clicks, movements, or touch gestures. It dynamically creates SVG elements with randomized colors and positions to generate the sparkle effect.
- let sparkInterval;
- function spark(e, opt_properties) {
- let mouseX, mouseY;
- let event = e;
- if (!e) {
- event = window.event;
- }
- if (event && (event.pageX || event.pageY)) {
- mouseX = event.pageX;
- mouseY = event.pageY;
- }
- else if (event && (event.clientX || event.clientY)) {
- mouseX = event.clientX + document.body.scrollLeft
- + document.documentElement.scrollLeft;
- mouseY = event.clientY + document.body.scrollTop
- + document.documentElement.scrollTop;
- }
- const defaultProperties = {color: `random`, mouseX: mouseX, mouseY: mouseY, hw: 30, sparks: 8, sw: 8, time: 400};
- const randInt = (min, max) => {return Math.floor(Math.random() * (max - min + 1)) + min;}
- const c = Object.assign(defaultProperties, opt_properties);
- const col = c.color === 'random' ? `rgb(${randInt(0,255)}, ${randInt(0,255)}, ${randInt(0,255)})` : c.color;
- const svg = document.createElementNS("http://www.w3.org/2000/svg", "svg");
- svg.setAttribute("viewBox", "0 0 100 100");
- svg.setAttribute("style", `width: 100%; height: 100%; position: absolute; height: ${c.hw}px; width: ${c.hw}px; transform: translate(-50%,-50%); left: ${c.mouseX}px; top: ${c.mouseY}px; z-index: 99999`);
- for (let i = 0; i < c.sparks; i++) {
- svg.insertAdjacentHTML('afterbegin', `<path d="M50 50 50 ${50 - c.sw/2}" stroke="${col}" stroke-linecap="round" stroke-width="${c.sw}" fill="none" transform="rotate(${((360 / c.sparks) * i) - (180 / c.sparks)} 50 50)"><animate attributeName="d" values="M50 50 50 ${50 - c.sw/2}; M50 ${50 - c.sw} 50 ${c.sw/2}; M50 ${c.sw/2} 50 ${c.sw/2}" dur="${c.time}ms" begin="0s" repeatCount="0" fill="freeze" /></path>`);
- }
- document.body.appendChild(svg);
- setTimeout(() => {svg?.remove();}, c.time);
- }
- document.addEventListener("click", (event) => {spark(event, {color: 'random', hw: 60}); clearInterval(sparkInterval);});
- document.addEventListener("mousemove", (event) => {spark(event, {color: 'random'}); clearInterval(sparkInterval);});
- document.addEventListener("touchmove", (event) => {const touch = event.touches[0]; // Get the first touch point
- const x = touch.clientX; // Get the x coordinate relative to the viewport
- const y = touch.clientY; // Get the y coordinate relative to the viewport
- spark({x, y}, {color: 'random'}); clearInterval(sparkInterval);});
- function infiniteSparkle() {
- sparkInterval = setInterval(()=> {
- const boundingBox = document.getElementById('getMe').getBoundingClientRect();
- spark(undefined, {color: 'random', mouseX: boundingBox.left + window.scrollX, mouseY: boundingBox.top + window.scrollY});
- }, 50);
- }
- infiniteSparkle();
That’s all! hopefully, you have successfully created the Sparkle Motion Effect on your web/app project. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
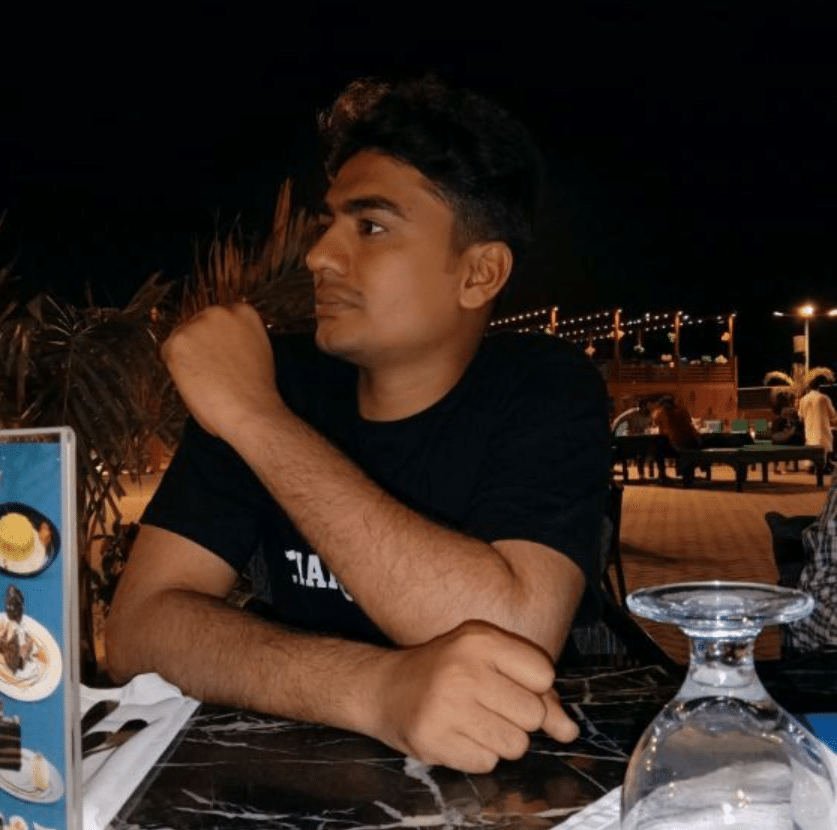
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.