This code creates CSS cards with a grid layout. It displays images, titles, and actions. The grid layout adjusts to different screen sizes. It helps in arranging content neatly in a responsive grid format.
You can use this code on websites to showcase products or articles. It enhances visual appeal with organized card layouts. It ensures content looks good on various devices, improving user experience.
How to Create CSS Cards With Grid Layout
1. Begin by setting up the HTML structure. Inside the <body>
tag, create a <div>
element with a class of “cards” to contain your cards. Each card will be represented by a <div>
with a class of “card”. Include elements such as <img>
, <h3>
, and <div>
for actions within each card.
Replace the placeholder content (e.g., image URLs, titles, prices) with your own content. Update the <img>
tag with the URLs of your images, <h3>
tags with your titles, and adjust prices accordingly.
- <h1>Subgrid and card layouts</h1>
- <div class="cards">
- <div class="card"><img src="https://picsum.photos/200/300" alt="random image from picusm"/>
- <h3>Title 1</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$25</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/200/400" alt="random image from picusm"/>
- <h3>Title 2</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$50</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/300/400" alt="random image from picusm"/>
- <h3>Long Title 3: forcing a multi line</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$15</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/200/500" alt="random image from picusm"/>
- <h3>Title 4</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$46</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/200/200" alt="random image from picusm"/>
- <h3>Title 5</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$50</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/300/200" alt="random image from picusm"/>
- <h3>Title 6</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$45</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/400/400" alt="random image from picusm"/>
- <h3>Title 7</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$60</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/200/400" alt="random image from picusm"/>
- <h3>Title 8</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$75</div>
- </div>
- </div>
- <div class="card"><img src="https://picsum.photos/200/500" alt="random image from picusm"/>
- <h3>Title 9</h3>
- <div class="actions">
- <button>Buy</button>
- <div class="prce">$100</div>
- </div>
- </div>
- <div class="card big">
- <h3>Notes</h3>
- <div class="notes">This is a simple demo of using subgrids for cards, this highlights how we can overcome the issues of having objects of multiple sizes, and aligning them, along with the adjoining cards. In this case the images are of different widths and heights, and to also throw in 1 card header that overflows a single line.
- <p> <code>Subgrid </code>is making this rather simpler to implement.</p>
- </div>
- </div>
- </div>
2. Copy the following CSS code into your stylesheet or <style>
tags within your HTML document. This CSS code defines the styling for the cards, including grid layout, card appearance, and responsive design
- @import url("https://fonts.googleapis.com/css2?family=Roboto:wght@100;400;700&display=swap");
- :root {
- font-family: "Roboto", sans-serif;
- }
- body{
- background-color: #EBEBE3;
- margin: 0;
- font-size: 1.2rem;
- }
- code {
- color: #7ea5b9;
- }
- h1 {
- font-weight: 100;
- text-align: center;
- }
- .cards {
- display: grid;
- grid-auto-flow: dense;
- grid-auto-rows: 200px auto auto;
- grid-template-columns: repeat(auto-fit, minmax(min(100%, 20ch), 1fr));
- gap: 1em;
- width: min(100ch, calc(100% - 2rem));
- margin: 0 auto;
- }
- .card {
- background-color: #F9F6EF;
- display: grid;
- grid-row: span 3;
- grid-template-rows: subgrid;
- border: 1px solid #0002;
- box-shadow: 0.1em 0.1em 0.5em #0003;
- border-radius: 0.5em;
- overflow: hidden;
- }
- .card img {
- height: 100%;
- width: 100%;
- object-fit: cover;
- object-position: 50% 80%;
- }
- .card h3, .card .actions {
- padding-inline: 0.5em;
- margin: 0;
- }
- .card .actions {
- display: flex;
- justify-content: space-between;
- padding-bottom: 1em;
- color: #1784ba;
- }
- .card .actions button {
- all: unset;
- animation: 0.3s ease;
- padding: 0.1em 0.5em;
- border: 1px solid transparent;
- border-radius: 0.3em;
- cursor: pointer;
- }
- .card .actions button:hover {
- border: 1px solid currentcolor;
- }
- .card .actions button:active {
- background-color: #1784ba;
- color: #F9F3EE;
- border: 1px solid #000;
- }
- .card .actions > * {
- padding: 0.1em 0.5em;
- }
- .big {
- grid-column: 1/-1;
- grid-row: 4/7;
- grid-template-rows: auto 1fr;
- }
- .big .notes {
- padding: 0.5em;
- }
- .big h3 {
- padding-top: 0.5em;
- }
Preview your webpage in a browser to see how the cards appear. Make adjustments to the HTML or CSS as needed to achieve your desired layout and styling. Test the responsiveness by resizing the browser window or viewing on different devices.
That’s all! hopefully, you have successfully created CSS cards on your website. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
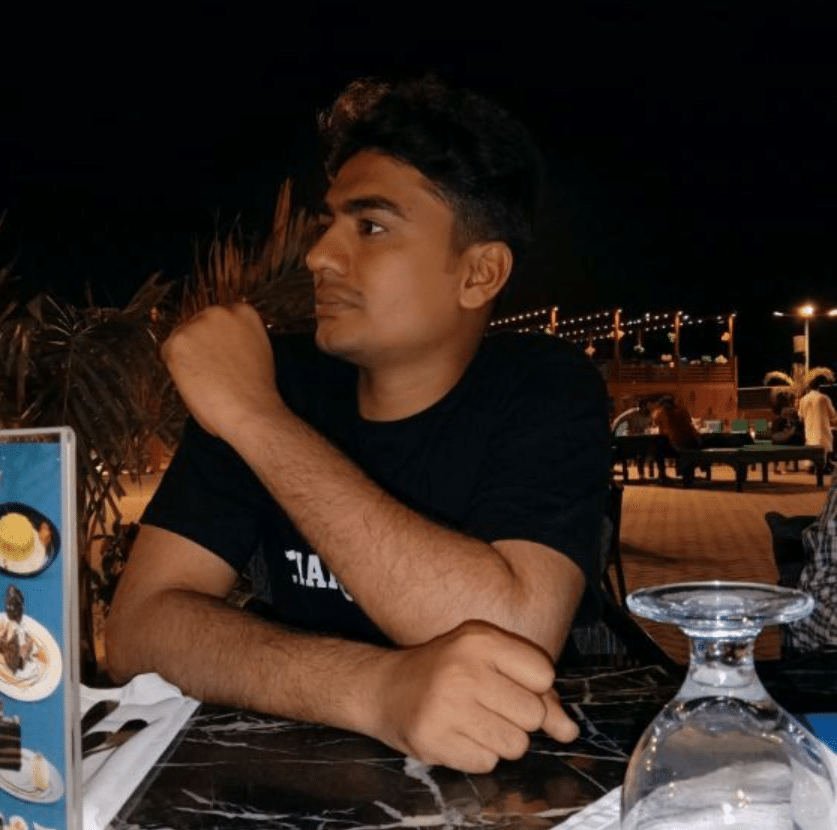
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.