This JavaScript code creates a captivating pixel background animation. The code generates a grid of squares that move and fade over time. It also allows you to interact by clicking, spawning new squares or resetting existing ones. The animation adapts to mouse movements, adding a dynamic touch to the pixelated display. Helpful for creating visually appealing backgrounds on web pages with an interactive twist.
How to Create Pixel Background Animation In JavaScript
1. Create the HTML structure for your webpage as follows:
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>Pixel Background Animation</title>
- </head>
- <body>
- <h2>Your Content Goes Here</h2>
- <script>
- // JavaScript code goes here
- </script>
- </body>
- </html>
2. Define the body and canvas styles in CSS to create a clean background and set the stage for the animation.
- body{
- min-height: 720px;
- margin: 0;
- padding: 0;
- background: url(https://picsum.photos/1920/1080) !important;
- background-position: center center;
- background-size: cover;
- position: relative;
- }
- canvas {
- top: 0;
- left: 0;
- width: 100%;
- height: 100%;
- }
3. Now, let’s delve into the JavaScript code. Copy and paste the following code into your HTML file, just before the closing </body>
tag.
This JavaScript code employs the HTML5 canvas element to create a grid of animated pixels. It initializes parameters such as the number of columns, rows, colors, and animation speed. The code handles mouse interactions, allowing users to click and interact with the pixel animation.
- (function() {
- var squares = [];
- var canvas = null;
- var lastFrame = new Date().getTime();
- var delta = 0;
- var mousePos = null;
- var debug = true;
- var click = false;
- var properties = {
- cols: 64,
- rows: 32,
- color: "#ffffff",
- altColor: "#1564a1",
- sLength: 0,
- activeAmt: 0.25,
- easeIn: 0.25
- };
- var init = function() {
- canvas = document.createElement('canvas');
- document.body.appendChild(canvas);
- canvas.width = canvas.offsetWidth;
- canvas.height = (canvas.offsetWidth / properties.cols * properties.rows);
- properties.sLength = canvas.offsetWidth / properties.cols;
- // Generate the Grid
- for (var r = 0; r < properties.rows; r++) {
- squares[r] = [];
- for (var c = 0; c < properties.cols; c++) {
- squares[r][c] = null;
- }
- }
- canvas.addEventListener('mousemove', function(evt) {
- mousePos = getMousePos(evt);
- });
- canvas.addEventListener('mouseout', function(evt) {
- mousePos = null;
- });
- canvas.addEventListener('mousedown', function(evt){
- mousePos = getMousePos(evt);
- click = true;
- });
- }
- var step = function() {
- delta = (new Date().getTime() - lastFrame) / 1000;
- lastFrame = new Date().getTime();
- // Spawn new Squares
- for (var i = 0; i < Math.ceil(properties.cols * delta); i++) {
- var c = Math.round(Math.random() * (properties.cols - 1));
- var r = Math.round(Math.random() * (properties.rows - 1));
- if (squares[r][c] == null) {
- var duration = Math.round(5 * (Math.random() + 0.5));
- squares[r][c] = new Square(c, r, duration);
- }
- }
- // Spawn squares under the mouse
- if (mousePos != null) {
- var col = Math.round((mousePos.x - properties.sLength / 2) / canvas.width * properties.cols);
- var row = Math.round((mousePos.y - properties.sLength / 2) / canvas.height * properties.rows);
- if(click){
- squares[row][col] = new Square(col, row, 5, false, "#f00");
- click = false;
- } else if(squares[row][col]) {
- squares[row][col].reset();
- } else {
- squares[row][col] = new Square(col, row, 10, false)
- }
- }
- // Remove old squares
- for (var r = 0; r < properties.rows; r++) {
- for (var c = 0; c < properties.cols; c++) {
- if (squares[r][c] != null && squares[r][c].life <= 0) {
- squares[r][c] = null;
- }
- }
- }
- render();
- requestAnimationFrame(step);
- }
- var render = function() {
- var ctx = canvas.getContext("2d");
- ctx.clearRect(0, 0, canvas.width, canvas.height);
- for (var r = 0; r < properties.rows; r++) {
- for (var c = 0; c < properties.cols; c++) {
- if (squares[r][c] != null) {
- squares[r][c].step(ctx);
- }
- }
- }
- }
- function getMousePos(evt) {
- var rect = canvas.getBoundingClientRect();
- return {
- x: evt.clientX - rect.left,
- y: evt.clientY - rect.top
- };
- }
- init();
- requestAnimationFrame(step);
- // Helper Function
- var getActiveNum = function() {
- var i = 0;
- for (var r = 0; r < properties.rows; r++) {
- for (var c = 0; c < properties.cols; c++) {
- if (squares[r][c] != null) {
- i++;
- }
- }
- }
- return i;
- }
- var Square = function(c, r, life, animIn, color) {
- if(typeof(animIn) == "undefined"){
- animIn = true;
- opacity = 0;
- } else {
- animIn = false;
- opacity = 1;
- }
- this.col = c;
- this.row = r;
- this.life = life;
- this.color = color ? color : properties.color;
- this.initialLife = life;
- this.animIn = animIn;
- this.opacity = opacity;
- this.step = function(ctx) {
- this.render(ctx);
- if (this.animIn) { //this.animIn){
- this.opacity = this.opacity + (1 / properties.easeIn * delta);
- if (this.opacity >= 1) {
- this.opacity = 1;
- this.animIn = false;
- }
- } else {
- this.life = this.life - delta;
- if (this.life < 0) {
- this.life = 0;
- }
- this.opacity = Math.round(1 * (this.life / this.initialLife) * 1000) / 1000;
- }
- };
- this.render = function(ctx) {
- var l = properties.sLength;
- ctx.globalAlpha = this.opacity;
- ctx.fillStyle = this.color;
- ctx.fillRect(this.col * l, this.row * l, l, l);
- if (debug == true) {
- ctx.globalAlpha = 1;
- ctx.fillStyle = "#000";
- ctx.font = "12px Arial";
- // ctx.fillText(this.col, this.col * l + 2, this.row * l + 12);
- // ctx.fillText(this.row, this.col * l + l - 14, this.row * l + 12);
- // ctx.fillText(Math.round(this.life), this.col * l + 2, this.row * l + (l / 2) + 10);
- // ctx.fillText(this.initialLife, this.col * l + 2, this.row * l + l - 4);
- // ctx.fillText(delta, this.col * l + l - 24, this.row * l + (l / 2) + 10);
- // ctx.fillText(this.opacity, this.col * l + l - 24, this.row * l + +l - 4);
- }
- };
- this.reset = function(){
- this.opacity = 1;
- this.life = this.initialLife;
- };
- }
- })();
Feel free to experiment with the code to customize the animation further. Adjust parameters like the number of columns, rows, colors, and animation speed to suit your preferences.
That’s all! hopefully, you have successfully created a Pixel Background Animation in JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
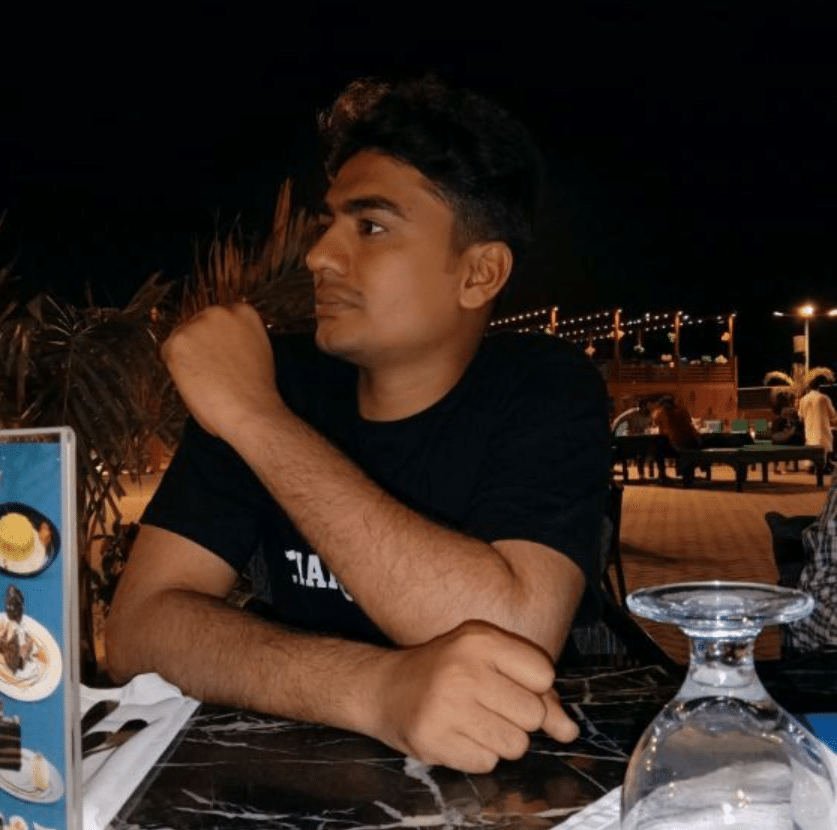
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.