This snippet helps you to create a tilt image effect on hover using CSS and JavaScript. It tracks the mouse’s position relative to the center of an image and applies a dynamic transform effect. When you hover over the image, it tilts in response to your mouse movement, creating a 3D-like effect.
This functionality is helpful for enhancing the user experience and adding interactive visual effects to images on your website.
How to Create Tilt Image On Hover Using CSS and JavaScript
1. We start by creating the HTML structure where our image will reside. You can place this code within your HTML document where you want to implement the tilt effect. Make sure to replace the src
attribute with the URL of the image you want to use.
- <!-- photo cred: @joelvodell on unsplash
- https://unsplash.com/photos/TApAkERW5pQ
- -->
- <div>
- <img class="tilt" src="https://images.unsplash.com/photo-1542856391-010fb87dcfed">
- </div>
2. Now, let’s add some CSS to style our image container and set the initial properties for our tilt effect. These styles are important for positioning and making the image look stylish.
- * {
- box-sizing: inherit;
- }
- body, html {
- margin: 0;
- background-color: #e6f3ee;
- }
- .tilt {
- position: absolute;
- top: 25%;
- left: 25%;
- width: 50%;
- height: auto;
- object-fit: cover;
- object-position: center center;
- opacity: 1;
- border-radius: 8px;
- cursor: pointer;
- }
3. The magic happens with JavaScript. We’ll apply the tilt effect to our image by tracking the mouse’s position relative to the image’s center and applying a dynamic transform:
- // basic idea is as follows:
- // measure the mouse's distance from center of the image and apply a transform rotateX and rotateY of between -5deg and 5deg accordingly
- const image = document.querySelector(".tilt");
- image.addEventListener("mousemove", event => {
- const { top, bottom, left, right } = event.target.getBoundingClientRect();
- const middleX = (right - left) / 2;
- const middleY = (bottom - top) / 2;
- const clientX = event.clientX;
- const clientY = event.clientY;
- const offsetX = (clientX - middleX) / middleX;
- const offsetY = (middleY - clientY) / middleY;
- event.target.style.transform = `perspective(1000px) rotateY(${offsetX *
- 5}deg) rotateX(${offsetY * 5}deg) scale3d(1, 1, 1)`;
- });
That’s all! hopefully, you have successfully created a tilt image effect on mouseover on your website. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
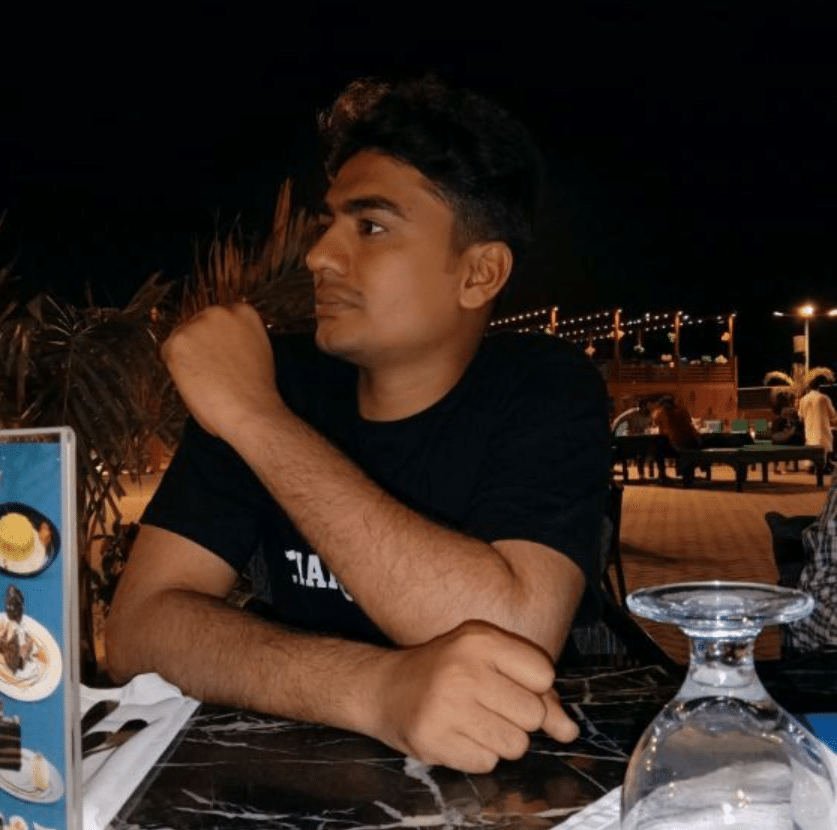
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.