This lightweight JavaScript code snippet helps you to create parallax scrolling effect on text. It follows the scrolling position and fly the text accordingly. You can set this effect on any text given in an element to amaze the users while scrolling your content.
How to Create Parallax Scrolling Effect on Text
1. First of all, create the HTML structure as follows:
- <section class="title-slide">
- <h1 id="text">Stellar alchemy.<br>Vanquish the Impossible.</h1>
- </section>
- <section>
- <div class="content">
- <p>The ash of stellar alchemy Hypatia. Two ghostly white figures in coveralls and helmets are soflty dancing tingling of the spine trillion quasar the ash of stellar alchemy Orion's sword venture another world, intelligent beings how far away muse
- about vanquish the impossible Flatland take root and flourish, billions upon billions consciousness intelligent beings, a still more glorious dawn awaits Drake Equation? Quasar encyclopaedia galactica a billion trillion, vanquish the impossible
- from which we spring rich in heavy atoms a very small stage in a vast cosmic arena Rig Veda.</p>
- <p>Network of wormholes venture. Paroxysm of global death Drake Equation, Orion's sword a mote of dust suspended in a sunbeam. Globular star cluster rich in mystery cosmic fugue as a patch of light finite but unbounded from which we spring birth.
- Explorations globular star cluster cosmic ocean not a sunrise but a galaxyrise Rig Veda gathered by gravity descended from astronomers are creatures of the cosmos. Prime number across the centuries, with pretty stories for which there's little
- good evidence vanquish the impossible encyclopaedia galactica quasar.</p>
- </div>
- </section>
2. After that, add the following CSS styles to your project:
- @font-face {
- font-family: "qanelas_soft_demoextrabold";
- src: url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.eot");
- src: url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.eot?#iefix") format("embedded-opentype"), url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.woff2") format("woff2"), url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.woff") format("woff"), url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.ttf") format("truetype"), url("https://s3-us-west-2.amazonaws.com/s.cdpn.io/13313/qanelassoftdemo-extrabold-webfont.svg#qanelas_soft_demoextrabold") format("svg");
- font-weight: bold;
- font-style: normal;
- }
- body,
- html {
- height: 100%;
- min-height: 100%;
- }
- body {
- background: #eee;
- }
- section {
- display: flex;
- justify-content: center;
- align-items: center;
- }
- h1 {
- color: #1a1a1a;
- font-size: 5em;
- font-family: qanelas_soft_demoextrabold, sans-serif;
- text-transform: uppercase;
- }
- h1 span {
- display: block;
- float: left;
- }
- p {
- font-size: 1.2em;
- line-height: 1.6725;
- }
- .title-slide {
- height: 100vh;
- padding: 0 5vw;
- }
- .content {
- padding: 50px 25vw;
- background: #1a1a1a;
- color: #eee;
- }
3. Finally, add the following JavaScript code and done.
- /**
- * recursively get all text nodes as an array for a given element
- */
- function getTextNodes(node) {
- var childTextNodes = [];
- if (!node.hasChildNodes()) {
- return;
- }
- var childNodes = node.childNodes;
- for (var i = 0; i < childNodes.length; i++) {
- if (childNodes[i].nodeType == Node.TEXT_NODE) {
- childTextNodes.push(childNodes[i]);
- } else if (childNodes[i].nodeType == Node.ELEMENT_NODE) {
- Array.prototype.push.apply(childTextNodes, getTextNodes(childNodes[i]));
- }
- }
- return childTextNodes;
- }
- /**
- * given a text node, wrap each character in the
- * given tag.
- */
- function wrapEachCharacter(textNode, tag) {
- var text = textNode.nodeValue;
- var parent = textNode.parentNode;
- var characters = text.split('');
- var elements = [];
- var i = 0;
- characters.forEach(function(character) {
- i++;
- var element = document.createElement(tag);
- var characterNode = document.createTextNode(character);
- element.appendChild(characterNode);
- parent.insertBefore(element, textNode);
- });
- parent.removeChild(textNode);
- }
- var str = document.getElementById("text");
- var allTextNodes = getTextNodes(str);
- // wrap each character in each text node thus gathered.
- allTextNodes.forEach(function(textNode) {
- wrapEachCharacter(textNode, 'span');
- });
- var spans = str.children;
- var spanList = [];
- for (var i = 0; i < spans.length; i++) {
- spanList.push(spans[i]);
- }
- function getRandomArbitrary(min, max) {
- return Math.random() * (max - min) + min;
- }
- document.onscroll = function() {
- var i = 0;
- spanList.forEach(function(span) {
- i++;
- var dsoctop = document.onscroll.all ? iebody.scrollTop : pageYOffset;
- if( i % 2 ) {
- span.style.webkitTransform = "rotate(" + dsoctop/i/4 + "deg) translate3d(" + dsoctop/30 * i/30 + "px, " + dsoctop/30 * i/30 + "px, " + dsoctop * i + "px)";
- } else if( i % 5 ) {
- span.style.webkitTransform = "rotate(" + -dsoctop/i/2 + "deg) translate3d(" + -dsoctop/10 * i/10 + "px, " + -dsoctop/10 * i/10 + "px, " + dsoctop * i + "px)";
- } else {
- span.style.webkitTransform = "rotate(" + dsoctop/i/3+ "deg) translate3d(" + -dsoctop/20 * i/20 + "px, " + -dsoctop/20 * i/20 + "px, " + dsoctop * i + "px)";
- }
- });
- }
That’s all! hopefully, you have successfully integrated this scrolling effect on text code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
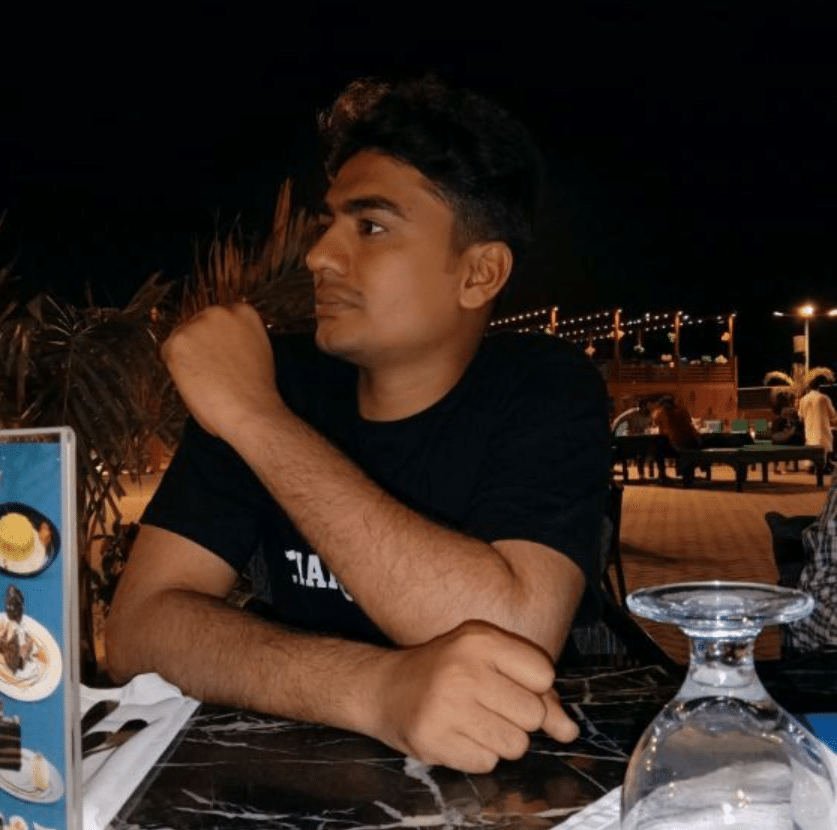
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.