This code creates a Header Image Parallax Scrolling Effect with CSS. It allows an image to move at a different speed while scrolling, creating an eye-catching visual effect. The code positions and manipulates the images based on scroll position for a simple, responsive design. It’s helpful for creating engaging headers with parallax effects using HTML and CSS.
You can use this code on your website’s header to add a captivating parallax scrolling effect. It’s easy to implement, requiring only HTML and CSS, and it offers a responsive design. Furthermore, it ensures working well on various screen sizes.
How to Create Header Image Parallax Scrolling Effect with CSS
1. First, create an HTML file and set up the basic structure for your website. Inside the <header>
element, you’ll need to add two sets of image elements: one for the background and one for the foreground. These images will create the parallax effect. Here’s how to structure each image element:
- <header class="hero center-bottom">
- <div class="hero-image hero-image-background">
- <picture>
- <source srcset="https://source.unsplash.com/1280x720?natural">
- <img src="https://source.unsplash.com/1280x720?natural" alt="background" width="1920" height="1280">
- </picture>
- </div>
- <div class="hero-image hero-image-foreground">
- <picture>
- <source srcset="https://source.unsplash.com/1280x720?natural">
- <img src="https://source.unsplash.com/1280x720?natural" alt="foreground" width="1920" height="1280">
- </picture>
- </div>
- <div class="hero-darken"></div>
- </header>
- <!-- only for demonstration -->
- <main>
- <!-- Your content goes here -->
- </main>
- <nav>
- <ul>
- <li><a href="#what">What</a></li>
- <li><a href="#why">Why</a></li>
- <li><a href="#align">Align</a></li>
- <li><a href="#extend">Extend</a></li>
- </ul>
- </nav>
2. Copy and paste the provided CSS code into your stylesheet. This CSS code defines the parallax scrolling effect, positioning, and responsiveness of the images. It’s important to keep the CSS classes and IDs consistent with your HTML structure.
- /* fonts */
- @import url('https://fonts.googleapis.com/css2?family=Sofia+Sans+Condensed:wght@400;700&family=Sofia+Sans:ital@0;1&display=swap');
- /* resets */
- *,
- *:before,
- *:after {
- margin: 0;
- padding: 0;
- box-sizing: border-box;
- }
- /* styles – required */
- html,
- body {
- height: 100%;
- scroll-behavior: smooth;
- }
- .hero {
- position: relative;
- height: 100vh !important;
- overflow: hidden;
- width: 100%;
- }
- .hero .hero-image {
- position: absolute;
- top: 0;
- right: 0;
- bottom: 0;
- left: 0;
- }
- .hero .hero-image img {
- position: absolute;
- width: 100%;
- height: 100%;
- object-fit: cover;
- }
- .hero.left-top .hero-image img {
- object-position: left top;
- }
- .hero.center-top .hero-image img {
- object-position: center top;
- }
- .hero.right-top .hero-image img {
- object-position: right top;
- }
- .hero.left-bottom .hero-image img {
- object-position: left bottom;
- }
- .hero.center-bottom .hero-image img {
- object-position: center bottom;
- }
- .hero.right-bottom .hero-image img {
- object-position: right bottom;
- }
- .hero .hero-darken {
- position: absolute;
- top: 0;
- right: 0;
- bottom: 0;
- left: 0;
- background-color: rgba(0,0,0,.1);
- }
- /* styles – only for demonstration */
- html {
- font-family: 'Sofia Sans', sans-serif;
- font-size: calc(1rem + 0.2 * ((100vw - 20rem) / 50));
- line-height: 1.4rem;
- }
- h1,
- h2 {
- font-family: 'Sofia Sans Condensed', sans-serif;
- font-weight: 700;
- line-height: 2.4rem;
- margin-bottom: 1.4rem;
- }
- p {
- margin-bottom: 1.4rem;
- }
- a {
- color: black;
- }
- a.anchor {
- display: block;
- position: relative;
- top: -100px;
- visibility: hidden;
- }
- em {
- opacity: .5;
- }
- main {
- width: 90%;
- max-width: 720px;
- margin: 3em auto 6em auto;
- }
- nav {
- position: absolute;
- z-index: 2;
- top: 30px;
- left: 50%;
- transform: translate(-50%, 0);
- width: 80%;
- max-width: 540px;
- background-color: black;
- border-radius: 12px;
- text-align: center;
- }
- nav ul {
- list-style: none;
- }
- nav ul li {
- display: inline;
- }
- nav ul li a {
- display: inline-block;
- font-family: 'Sofia Sans Condensed', sans-serif;
- font-weight: 400;
- letter-spacing: .1em;
- padding: .5em;
- color: white;
- text-decoration: none;
- text-transform: uppercase;
- }
- @media screen and (min-width: 30em) {
- nav ul li a {
- padding: .5em 1em;
- letter-spacing: .15em;
- opacity: .75;
- }
- nav ul li a:hover {
- opacity: 1;
- }
- }
- /* scroll animation */
- .hero .hero-darken:before,
- .hero .hero-darken:after {
- content: '';
- position: absolute;
- right: 0;
- left: 0;
- margin-left: auto;
- margin-right: auto;
- text-align: center;
- }
- .hero .hero-darken:before {
- top: 104px;
- width: 30px;
- height: 50px;
- border: 2px solid black;
- border-radius: 12px;
- }
- .hero .hero-darken:after {
- top: 112px;
- width: 2px;
- height: 12px;
- background-color: black;
- border-radius: 1px;
- animation: scroll 2s infinite linear;
- }
- @keyframes scroll {
- from {
- top: 112px;
- }
- to {
- top: 122px;
- }
- }
You can customize the parallax effect further by adjusting the CSS properties, such as object position and image size, to achieve your desired look. Experiment with different images to find what works best for your website.
3. Finally, include the following JavaScript code to make the parallax effect work. Place it just before the closing </body>
tag:
- document.addEventListener('DOMContentLoaded', function() {
- var backgroundElements = document.querySelectorAll('.hero-image-background');
- var foregroundElements = document.querySelectorAll('.hero-image-foreground');
- window.addEventListener('scroll', function() {
- var scrollPosWin = window.scrollY;
- var factorBackground = scrollPosWin * 0.4;
- var factorForeground = scrollPosWin * 0.2;
- backgroundElements.forEach(function(element) {
- element.style.top = factorBackground + 'px';
- element.style.bottom = -factorBackground + 'px';
- });
- foregroundElements.forEach(function(element) {
- element.style.top = factorForeground + 'px';
- element.style.bottom = -factorForeground + 'px';
- });
- });
- });
That’s all! hopefully, you have successfully created the header image parallax scrolling effect. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
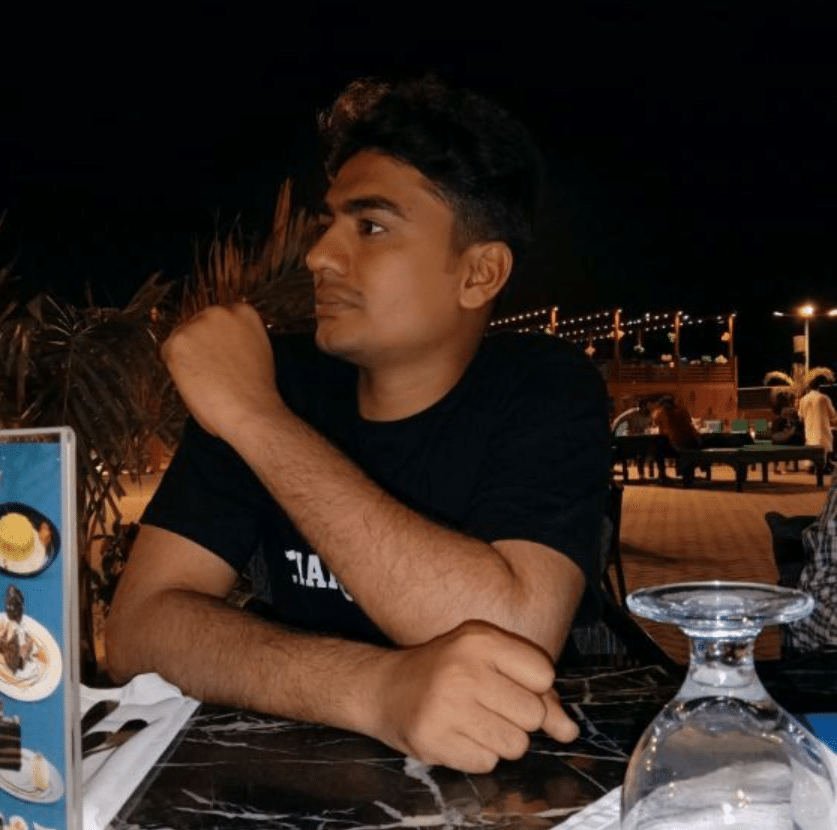
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.