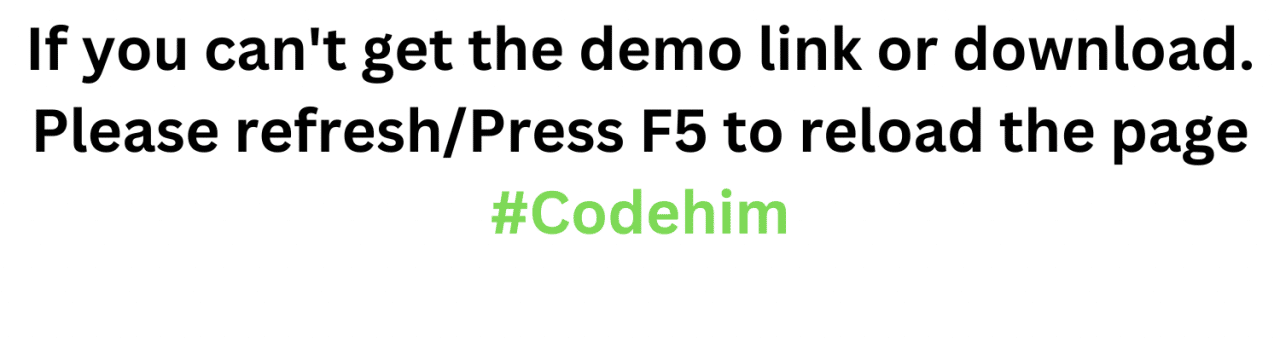
Tabs are a great way to organize and display content on your website in an organized and user-friendly manner. This JavaScript code enables tab navigation with an indicator. It allows switching content by clicking tabs. The method hides and displays content panels, applying active styling to the selected tab.
This code improves user experience by organizing and displaying information in an easily navigable way.
How to Create Tab Navigation With Indicator in JavaScript
1. First of all, load the Normalize CSS and Google Fonts by adding the following CDN links into the head tag of your HTML document.
<link href="https://fonts.googleapis.com/css?family=Roboto:400,700" rel="stylesheet"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css">
2. Create the HTML structure for your tabbed navigation system. The following code includes a container for tabs and content panels. You can customize the tab names and content as needed.
<div class="tabs"> <nav class="tabs-nav"> <ol class="nav-list"> <li class="nav-item"> <a href="#description" class="nav-link">Description</a> </li> <li class="nav-item"> <a href="#updates" class="nav-link">Updates</a> </li> <li class="nav-item"> <a href="#donations" class="nav-link">Donations</a> </li> </ol> </nav> <div class="tabs-panels"> <div class="tabs-panel" id="description" style="">Here you will find the description.</div> <div class="tabs-panel" id="updates" style="display: none;">Here you will find updates.</div> <div class="tabs-panel" id="donations" style="display: none;">Here you will find <a href="">donations</a>.</div> </div> </div>
3. Now, include the following CSS code in the <style>
section of your HTML document or in an external CSS file. This code defines the styling for your tabs and content panels. You can modify the styles to match your website’s design.
*, *::before, *::after { box-sizing: border-box; } body { padding: 0.5rem; background: #ddd; font-family: 'Roboto', 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif; } ul, ol { list-style: none; padding: 0; margin: 0; } .tabs { max-width: 40rem; margin: 2rem auto; } .nav-list { display: flex; background: #fff; border-bottom: 1px solid #ddd; } .nav-item { flex: 0 0 8rem; } .nav-link { display: block; padding: 1rem; border-top: 3px solid transparent; text-decoration: none; text-align: center; color: inherit; outline: none; } .nav-link:hover, .nav-link:focus, .nav-link:active, .nav-link--active { font-weight: bold; } .nav-link--active { border-top-color: sienna; } .tabs-panels { background: #fff; } .tabs-panel { padding: 2rem; }
4. Finally, copy the following JavaScript code and place it just before the closing </body>
tag in your HTML document. This code is responsible for handling tab navigation and indicator functionality.
const doc = document const tabsPanels = [...doc.querySelectorAll('.tabs-panel')] const navLinks = [...doc.querySelectorAll('.nav-link')] function hideTabsPanels() { tabsPanels.forEach(panel => { panel.style.display = 'none' }) } const activeClassName = 'nav-link--active' function removeActiveStyle() { navLinks.forEach(link => { link.classList.remove(activeClassName) }) } function addActiveStyle(el) { el.classList.add(activeClassName) } hideTabsPanels() const hash = window.location.hash if (hash) { const panelName = hash.slice(1) doc.getElementById(panelName).style.display = '' addActiveStyle(doc.querySelector('[href="#' + panelName + '"]')) } else { tabsPanels[0].style.display = '' addActiveStyle(doc.querySelector('.nav-link')) } navLinks.forEach(link => { link.addEventListener('click', function() { const panelName = this.href.slice(this.href.indexOf('#') + 1) hideTabsPanels() doc.getElementById(panelName).style.display = '' removeActiveStyle() addActiveStyle(this) }) }) window.addEventListener('hashchange', function() { hideTabsPanels() const panelName = window.location.hash.slice(1) doc.getElementById(panelName).style.display = '' removeActiveStyle() addActiveStyle(doc.querySelector('[href="#' + panelName + '"]')) })
That’s all! hopefully, you have successfully created Tab Navigation with Indicator using JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
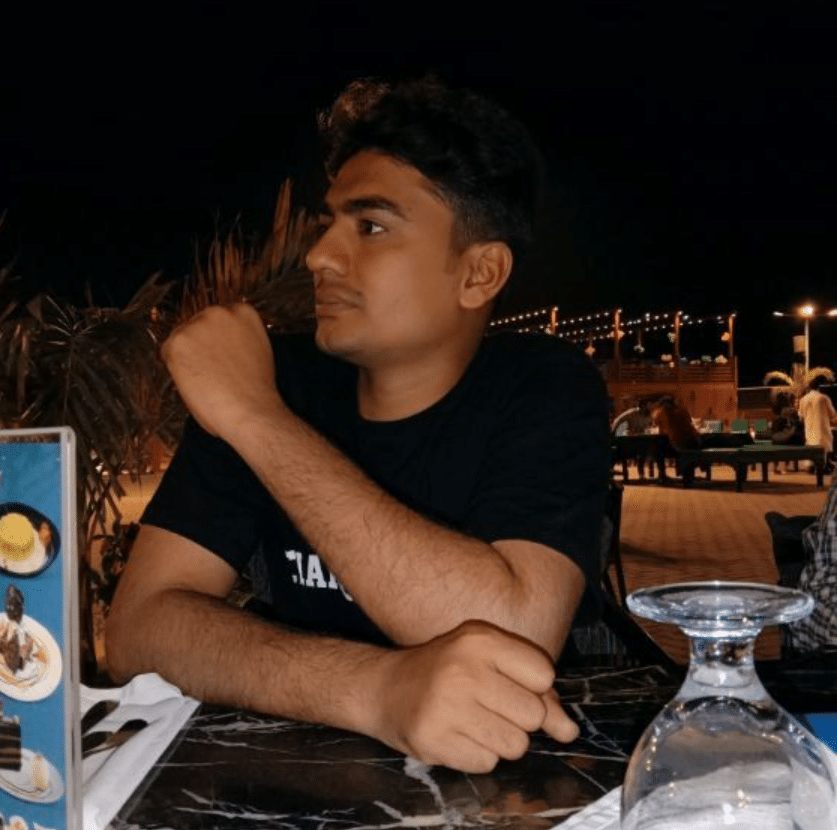
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.