If you’re looking for a simple Vanilla JS carousel solution, this code snippet might be helpful for you to build an image carousel with fading/sliding effect. It allows users to slide the carousel images with next and previous button.
How to Create Image Carousel using Vanilla JS
1. In the first step, create a div element with a class name “carousel” and place your image inside it. Similarly, add your all images by following the same method.
Create the button element with next and prev IDs at the end of images. Wrap all elements into a div element and define its ID “item”. So, the complete HTML structure for a simple image carousel looks like below:
- <div id="item">
- <div class="carousel">
- <img src="https://unsplash.it/600/300" alt="" />
- </div>
- <div class="carousel">
- <img src="https://unsplash.it/600/302" alt="" />
- </div>
- <div class="carousel">
- <img src="https://unsplash.it/600/303" alt="" />
- </div>
- <div class="carousel">
- <img src="https://unsplash.it/600/304" alt="" />
- </div><br>
- <i id="prev" class="fa fa-chevron-left"></i>
- <i id="next" class="fa fa-chevron-right"></i>
- </div>
2. Now, use the following CSS to style the image carousel. You can set the custom width and height for the #item selector in order to customize the size of carousel.
- body {
- background: #333;
- }
- #item {
- width: 800px;
- height: 500px;
- padding: 30px;
- padding-left: 170px;
- margin: 0 auto;
- margin-top: 100px;
- position: relative;
- }
- .carousel {
- width: 600px;
- height: 300px;
- overflow: hidden;
- border: #fff solid 5px;
- }
- #prev, #next {
- font-size: 40px;
- color: #fff;
- position: absolute;
- top: 0;
- left: 0;
- }
- #prev {
- top: 150px;
- left: 90px;
- cursor: pointer;
- }
- #next {
- top: 150px;
- left: 830px;
- cursor: pointer;
- }
3. Finally, add the following JavaScript carousel function to activate the slider.
- /*
- @name:Pure JS carousel
- @creator: endy
- @ref: simply-coded
- */
- //carousel object
- function Carousel(containerID) {
- this.container = document.getElementById(containerID) || document.body;
- this.slides = this.container.querySelectorAll('.carousel');
- this.total = this.slides.length - 1;
- this.width = this.container.offsetWidth;
- this.height = this.container.offsetHeight;
- this.current = 0;
- //start on slide 1
- this.slide(this.current);
- //animation effect
- this._animate = function (direction, effect, interval) {
- var dRate = 1.2;
- if (effect == "slide") {
- var width = this.width;
- var left = 0,opacity = 1;
- var rate, playing;
- if (direction == 'forward') {
- if (interval === false || interval >= dRate * 1000 + 200) {
- // Default animation speed. rate = 10; is for 1 second.
- rate = dRate * 10;
- } else if (interval < dRate * 1000 + 200 && interval > 400) {
- // Adjusts the animation duration to complete within the interval time period. +200 millisecond pause.
- rate = (interval - 200) / 2 / 50;
- } else {
- // Make faster than 400 millisecond animations continuous without any pause.
- rate = interval / 2 / 50;
- }
- playing = setInterval(slideOut, rate);
- var elemOut = this.slides[this.current];
- this.current === this.total ? this.current = 0 : this.current += 1;
- var elemIn = this.slides[this.current];
- function slideOut() {
- left -= width / 100;
- elemOut.style.left = left + "px";
- if (opacity > 0) {
- opacity -= 1 / (width / 2) * (width / 100);
- }
- elemOut.style.opacity = opacity;
- if (left <= -width / 2) {
- clearInterval(playing);
- elemOut.style.display = "none";
- elemOut.style.left = "0px";
- elemOut.style.opacity = "1";
- elemIn.style.left = width / 2 + "px";
- elemIn.style.opacity = "0";
- elemIn.style.display = "inline-block";
- left = width / 2;
- playing = setInterval(slideIn, rate);
- }
- }
- function slideIn() {
- left -= width / 100;
- elemIn.style.left = left + "px";
- if (opacity < 1) {
- opacity += 1 / (width / 2) * (width / 100);
- }
- elemIn.style.opacity = opacity;
- if (left <= 0) {
- clearInterval(playing);
- elemIn.style.left = "0px";
- }
- }
- } else
- if (direction == 'backward') {
- if (interval === false || interval >= dRate * 1000 + 200) {
- // Default animation speed. rate = 10; is for 1 second.
- rate = dRate * 10;
- } else if (interval < dRate * 1000 + 200 && interval > 400) {
- // Adjusts the animation duration to complete within the interval time period. +200 millisecond pause.
- rate = (interval - 200) / 2 / 50;
- } else {
- // Make faster than 400 millisecond animations continuous without any pause.
- rate = interval / 2 / 50;
- }
- playing = setInterval(slideOut, rate);
- var elemOut = this.slides[this.current];
- this.current === this.total ? this.current = 0 : this.current += 1;
- var elemIn = this.slides[this.current];
- function slideOut() {
- left -= width / 100;
- elemOut.style.left = left + "px";
- if (opacity > 0) {
- opacity -= 1 / (width / 2) * (width / 100);
- }
- elemOut.style.opacity = opacity;
- if (left <= -width / 2) {
- clearInterval(playing);
- elemOut.style.display = "none";
- elemOut.style.left = "0px";
- elemOut.style.opacity = "1";
- elemIn.style.left = width / 2 + "px";
- elemIn.style.opacity = "0";
- elemIn.style.display = "inline-block";
- left = width / 2;
- playing = setInterval(slideIn, rate);
- }
- }
- function slideIn() {
- left -= width / 100;
- elemIn.style.left = left + "px";
- if (opacity < 1) {
- opacity += 1 / (width / 2) * (width / 100);
- }
- elemIn.style.opacity = opacity;
- if (left <= 0) {
- clearInterval(playing);
- elemIn.style.left = "0px";
- }
- }
- }
- } else
- {
- console.log(elimOut);
- this.slide(this.current);
- }
- };
- }
- //? is inline conditional : is else
- //next function
- Carousel.prototype.next = function (effect, interval) {
- effect = effect || false;
- interval = interval || false;
- this.stop();
- this._animate('forward', effect, interval);
- //check interval type to avoid any abuse XD
- if (typeof interval === 'number' && interval % 1 === 0) {
- var context = this;
- this.run = setTimeout(() => {
- context.next(interval);
- }, interval);
- }
- };
- //prev function
- Carousel.prototype.prev = function (effect, interval) {
- this.stop();
- this._animate('backward', effect, interval);
- //check interval type to avoid any abuse XD
- if (typeof interval === 'number' && interval % 1 === 0) {
- var context = this;
- this.run = setTimeout(() => {
- context.prev(interval);
- }, interval);
- }
- };
- //stop function
- Carousel.prototype.stop = function () {
- clearTimeout(this.run);
- };
- //select slide function logic
- Carousel.prototype.slide = function (index) {
- if (index >= 0 && index <= this.total) {
- this.stop();
- for (var i = 0; i <= this.total; i++) {
- i === index ? this.slides[i].style.display = 'block' : this.slides[i].style.display = 'none';
- }
- } else
- {
- alert('Index ' + index + "doesn't exist. Available: 0 -" + this.total);
- }
- };
- //using the plugin
- var slider = new Carousel('item');
- var nextbtn = document.getElementById('next');
- var prevbtn = document.getElementById('prev');
- nextbtn.onclick = function () {
- slider.next('slide');
- };
- prevbtn.onclick = function () {
- slider.prev('slide');
- };
That’s all! hopefully, you have successfully integrated this image carousel into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
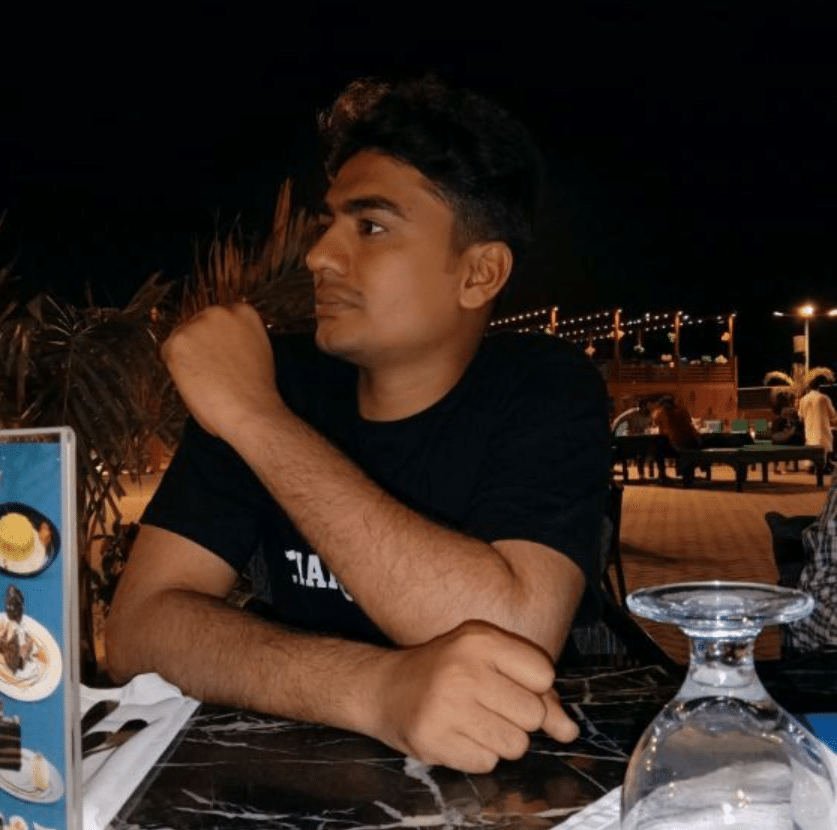
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.