This code creates custom select dropdowns in a webpage. It uses ul and li elements styled with CSS to mimic dropdown functionality. The JavaScript manages the opening and closing of the dropdowns when clicked, allowing selection of items within them. This is helpful for creating custom-styled select dropdown menus without relying on the default browser behavior.
How to Create Custom Select Dropdown Using Ul Li In Javascript
1. Start by setting up the HTML structure for your custom dropdowns. Use unordered lists (ul
) and list items (li
) to create the dropdown options. For example:
- <div class="block-wrapper">
- <ul class="select">
- <li>
- <input class="select-field" value="" placeholder="Select an element" readonly />
- <span></span>
- <ul class="select-list">
- <li>Item I</li>
- <li>Item II</li>
- <li>Item III</li>
- </ul>
- </li>
- </ul>
- <ul class="select">
- <li>
- <input class="select-field" value="" placeholder="Select an element" readonly />
- <span></span>
- <ul class="select-list">
- <li>Item A</li>
- <li>Item B</li>
- <li>Item C</li>
- </ul>
- </li>
- </ul>
- </div>
Modify the dropdown options by updating the content within the <ul class="select-list">
for each select element. Customize the items to fit the specific choices you want to offer.
2. Style the dropdown elements using the following CSS code. Customize the CSS properties as per your design preferences.
- @import url("https://fonts.googleapis.com/css2?family=Montserrat:wght@300;400;500&display=swap");
- :root {
- --font: "Montserrat", sans-serif;
- --fontWeightLight: 300;
- --fontWeightRegular: 400;
- --fontWeightMedium: 500;
- --textColor: #000;
- --textColorLight: #444;
- }
- * {
- box-sizing: border-box;
- margin: 0;
- padding: 0;
- }
- body {
- padding: 20px;
- font-family: var(--font);
- font-weight: var(--fontWeightRegular);
- color: var(--textColor);
- }
- ul {
- list-style: none;
- }
- .block-wrapper {
- display: flex;
- gap: 30px;
- padding: 20px 0 40px;
- }
- .select {
- position: relative;
- width: 100%;
- max-width: 350px;
- background: #fff;
- }
- .select-field {
- padding: 12px 32px 12px 12px;
- width: 100%;
- font-family: inherit;
- font-weight: var(--fontWeightMedium);
- font-size: 1em;
- color: #444;
- background-color: unset;
- border: 2px solid #7e9bbd;
- border-radius: 4px;
- transition: 0.2s;
- z-index: 1;
- cursor: pointer;
- }
- .select-field:focus {
- outline: transparent;
- box-shadow: 0 0 3px #7e9bbd;
- }
- .select-field::placeholder {
- font-weight: var(--fontWeightLight);
- color: #444;
- }
- .select-field + span {
- display: flex;
- align-items: center;
- justify-content: center;
- position: absolute;
- top: 0;
- right: 0;
- bottom: 0;
- width: 32px;
- }
- .select-field + span::after {
- content: "";
- width: 0;
- height: 0;
- border-style: solid;
- border-width: 8px 8px 0 8px;
- border-color: #008095 transparent transparent transparent;
- pointer-events: none;
- transition: 0.5s;
- z-index: 1;
- }
- .select-field.turn + span::after {
- border-width: 0 8px 8px 8px;
- border-color: transparent transparent #008095 transparent;
- }
- .select-list {
- display: none;
- position: absolute;
- top: 45px;
- left: 0;
- width: 100%;
- background-color: #fff;
- border: 2px solid #7e9bbd;
- animation: fadeIn 0.4s;
- z-index: 10;
- }
- .select-list.open {
- display: block;
- }
- .select-list li {
- display: block;
- color: var(--textColor);
- font-weight: var(--textWeightLight);
- padding: 12px 12px 12px 12px;
- transition: all 0.2s ease-out;
- cursor: pointer;
- }
- .select-list li:not(:last-child) {
- border-bottom: 1px solid #7e9bbd;
- }
- .select-list li:hover {
- background-color: #008095;
- color: #fff;
- }
- @keyframes fadeIn {
- from {
- opacity: 0;
- }
- to {
- opacity: 1;
- }
- }
3. Finally, add the JavaScript code to enable the dropdown functionality. The SelectManager
class manages the opening and closing of the dropdowns when clicked, allowing the selection of items within them.
- class SelectManager {
- constructor(containerSelector, selectSelector) {
- this.container = document.querySelector(containerSelector);
- this.selectSelector = selectSelector;
- this.init();
- }
- init() {
- this.container.addEventListener("click", (e) => {
- const targetSelect = e.target.closest(this.selectSelector);
- if (targetSelect) {
- this.handleSelectClick(targetSelect);
- } else {
- this.closeAllSelects();
- }
- });
- document.addEventListener("click", (e) => {
- if (
- !e.target.closest(this.selectSelector) &&
- !e.target.closest(".select-list")
- ) {
- this.closeAllSelects();
- }
- });
- }
- handleSelectClick(select) {
- const field = select.querySelector(".select-field");
- const list = select.querySelector(".select-list");
- this.closeOtherSelects(field);
- list.classList.toggle("open");
- field.classList.toggle("turn");
- list.addEventListener("click", (e) => {
- e.stopPropagation();
- });
- const selectItemClickHandler = (e) => {
- const selectedItem = e.target.closest("li");
- if (selectedItem) {
- const result = selectedItem.textContent.trim();
- field.value = result;
- list.classList.remove("open");
- field.classList.remove("turn");
- list.removeEventListener("click", selectItemClickHandler);
- }
- };
- list.addEventListener("click", selectItemClickHandler);
- }
- closeOtherSelects(clickedField) {
- var selects = this.container.querySelectorAll(this.selectSelector);
- for (var i = 0; i < selects.length; i++) {
- var select = selects[i];
- var field = select.querySelector(".select-field");
- var list = select.querySelector(".select-list");
- if (field && list && field !== clickedField) {
- if (list.classList.contains("open")) {
- list.classList.remove("open");
- }
- if (field.classList.contains("turn")) {
- field.classList.remove("turn");
- }
- }
- }
- }
- closeAllSelects() {
- let selects = this.container.querySelectorAll(this.selectSelector);
- for (let i = 0; i < selects.length; i++) {
- let select = selects[i];
- const field = select.querySelector(".select-field");
- const list = select.querySelector(".select-list");
- if (list && field) {
- list.classList.remove("open");
- field.classList.remove("turn");
- }
- }
- }
- }
- // Init
- const selectManager = new SelectManager("body", ".select");
That’s all! hopefully, you have successfully created a custom select dropdown using ul li elements in JavaScript. This solution provides a visually appealing alternative to the default browser select dropdowns. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
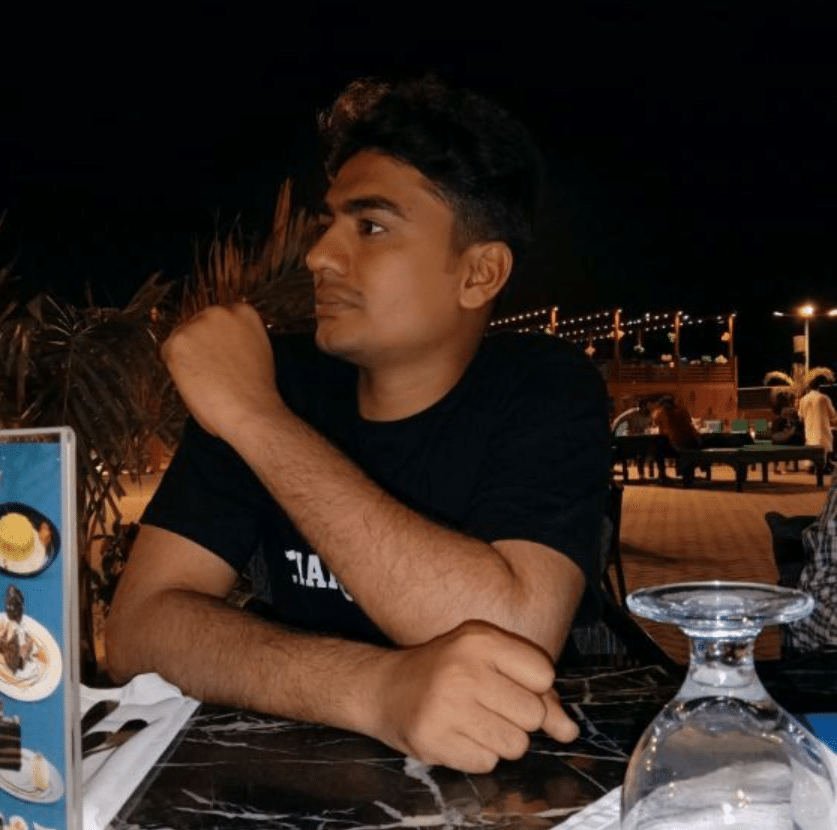
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.