This simple JavaScript code creates a text-to-speech converter. It takes text input and reads it aloud. The code sets up a user interface with a text area and a button to initiate the speech. When you enter text and press the button, the code converts the text into speech using the browser’s built-in speech synthesis capabilities.
You can use this code on websites to make your content accessible. It helps visually impaired users by converting text into speech, improving accessibility.
How to Create a Simple Text To Speech Converter in JavaScript
1. First of all, copy the HTML code into your file. It creates a container with a text area for input and a button to trigger the speech synthesis.
- <div class="container">
- <label for="text">Text to speak:</label>
- <textarea id="text"></textarea>
- <button id="speak">Speak Text</button>
- </div>
2. Insert the following CSS code for styling. It ensures a clean and responsive layout for your TTS converter.
- *{
- box-sizing: border-box;
- margin: 0;
- }
- body{
- display: flex;
- justify-content: center;
- align-items: center;
- min-height: 100vh;
- }
- .container{
- background-color: #4FBDBA;
- display: grid;
- gap: 20px;
- width: 500px;
- max-width: calc(100vw - 40px);
- padding: 30px;
- border-radius: 10px;
- font-size: 1.2rem;
- }
- #text{
- display: block;
- height: 100px;
- border-radius: .5rem;
- font-size: 1.5rem;
- border: none;
- resize: none;
- padding: 8px 10px;
- outline: 2px solid rgba(120,120,120,0.623);
- }
- button{
- padding: 10px;
- background: #072227;
- color: #fff;
- border-radius: .5rem;
- cursor: pointer;
- border: none;
- font-size: 1rem;
- font-weight: bold;
- }
3. Finally, add the following JavaScript code to enable the text-to-speech functionality. It listens for button clicks, converts the entered text into speech, and cancels any ongoing speech.
- const textEL = document.getElementById('text');
- const speakEL = document.getElementById('speak');
- speakEL.addEventListener('click', speakText);
- function speakText() {
- // stop any speaking in progress
- window.speechSynthesis.cancel();
- const text = textEL.value;
- const utterance = new SpeechSynthesisUtterance(text);
- window.speechSynthesis.speak(utterance);
- }
Open your HTML file in a browser, enter text, and click the “Speak Text” button. Experience the simplicity of adding text-to-speech functionality to your website!
That’s all! hopefully, you have successfully created a Simple Text-to-Speech Converter in JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
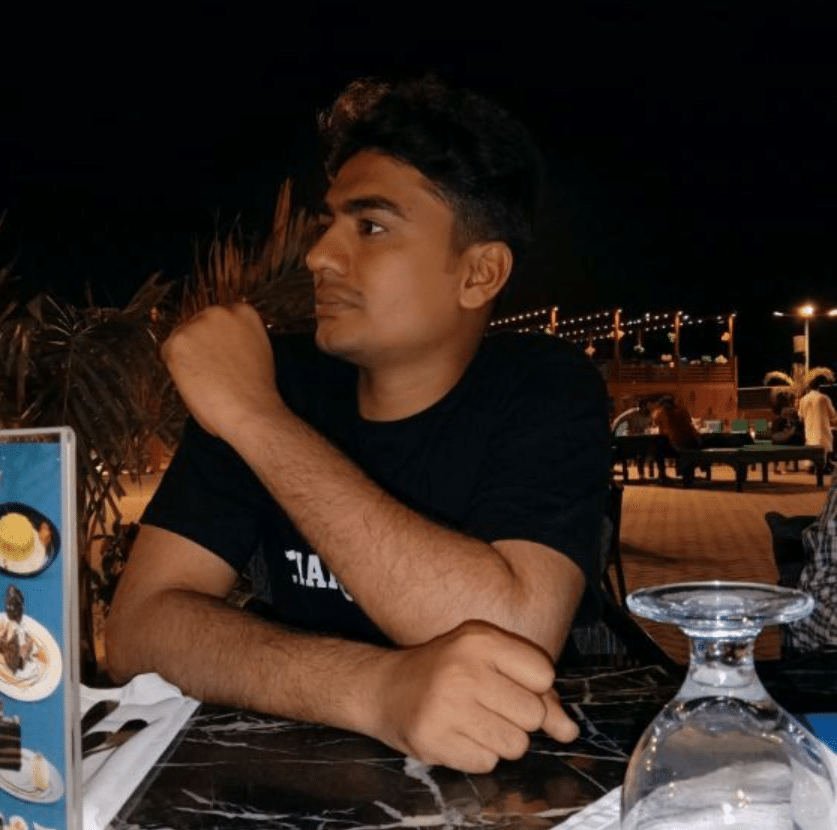
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.