This code snippet helps you to create a simple grocery list app in JavaScript. It comes with a user-friendly interface to allow users to enter grocery items with quantity to the shopping list. Similarly, users can remove the added items from the list or mark the items that have been purchased.
You can easily integrate this grocery app into your shopping website project/template to allow users to make their shopping lists. On the other hand, it can also be used as a separate grocery list web app that may help users to make digital shopping items lists.
Basically, this grocery app project depends on jQuery and Backbone JS to add, remove and mark items in an efficient way. So, it can be highly customized with additional functions and features according to your needs.
How to Create Simple Grocery List App in JavaScript
1. First of all, create the HTML structure for the grocery app as follows. You can place the following HTML code anywhere in the webpage where you want to create the grocery app.
- <header>
- Simple grocery list
- </header>
- <main>
- <form>
- <label for="item-input">Item</label>
- <input type="text" id="item-input">
- <label for="quantity-input">Quantity</label>
- <input type="number" id="quantity-input">
- <button type="submit" id="submit-btn">Add</button>
- </form>
- <div id="lists">
- <p class="list__label">Still need to grab <span class="quantity" id="shopping-num"></span></p>
- <ul id="shopping-list">
- </ul>
- <p class="list__label">In cart <span class="quantity" id="bought-num"></span></p>
- <ul id="bought-list">
- </ul>
- </div>
- </main>
- <div class="push"></div>
- </div>
2. After that, style the grocery app with the following CSS styles. You can also use your own CSS or change styles in order to customize the app interface.
- header,
- footer {
- color: white;
- background-color: #004A55;
- width: 100%;
- text-align: center;
- padding: 0.5em;
- position: relative;
- }
- footer,
- .push {
- height: 2em;
- }
- .wrapper {
- margin: 0 auto -2em;
- min-height: 100%;
- }
- form {
- width: inherit;
- }
- label {
- display: block;
- line-height: 1.5em;
- }
- input {
- width: inherit;
- padding: 1em;
- }
- .delete, button {
- color: white;
- background-color: #F87D09;
- }
- button {
- border: 0;
- padding: 1em;
- width: inherit;
- margin: 0.75em 0;
- }
- .icon {
- display: inline-block;
- width: 0.9em;
- height: 0.9em;
- margin-right: 0.25em;
- fill: #004A55;
- }
- #bought-list li:before, #shopping-list li:before {
- content: "";
- display: inline-block;
- background-size: 100%;
- height: 0.9em;
- width: 1em;
- margin-right: 0.3em;
- }
- #shopping-list li:before {
- background-image: url("https://res.cloudinary.com/ddy54k4ks/image/upload/v1454106412/svg/checkmark2.svg");
- }
- #bought-list li:before {
- background-image: url("https://res.cloudinary.com/ddy54k4ks/image/upload/v1454106412/svg/checkmark.svg");
- }
- ul {
- list-style: none;
- margin: 0 auto;
- padding: 0;
- }
- li {
- color: #004A55;
- padding: 0.75em;
- cursor: pointer;
- margin: 0.5em 0;
- border: 1px solid #004A55;
- position: relative;
- transition: background-color 0.2s;
- }
- li:hover {
- background-color: #A7CDCC;
- }
- .quantity {
- color: #F87D09;
- }
- .quantity:before {
- content: "(";
- }
- .quantity:after {
- content: ")";
- }
- .delete {
- float: right;
- padding: inherit;
- position: absolute;
- right: 0;
- top: 0;
- }
- .delete:after {
- clear: both;
- }
- #bought-list li {
- background-color: #F6F6F6;
- }
- #bought-list li .quantity {
- color: #666666;
- }
- .list__label {
- border-bottom: 2px solid #F87D09;
- font-size: 0.75em;
- color: #004A55;
- padding: 0;
- }
3. After creating HTML and CSS styles for the grocery app, load the following dependencies just before closing of the body tag.
- <!-- jQuery -->
- <script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.0.0-alpha1/jquery.min.js'></script>
- <!-- Lodash JS -->
- <script src='https://cdnjs.cloudflare.com/ajax/libs/lodash.js/3.10.1/lodash.min.js'></script>
- <!-- Backbone JS -->
- <script src='https://cdnjs.cloudflare.com/ajax/libs/backbone.js/1.2.3/backbone-min.js'></script>
4. Finally, functionalize and initialize the app with the following JavaScript function and done. You can place the following code between <script> tag just after the dependencies files. Or create a separate JS file and include it into your project.
- const classNames = {
- DELETE: "delete" };
- const logger = {
- logging: false,
- log(msg) {
- if (this.logging) console.log(msg);
- } };
- const itemProto = {
- bought: false,
- toggle() {
- this.bought = !this.bought;
- this.trigger("toggled", this);
- } };
- const items = {
- list: [],
- add(item) {
- let newItem = Object.create(itemProto);
- Object.assign(newItem, item, Backbone.Events);
- newItem.on("toggled", function (item) {
- logger.log("toggled");
- view.addToList(item);
- });
- newItem.id = _.uniqueId();
- this.list.push(newItem);
- this.trigger("itemAdded", newItem);
- this.trigger("updated");
- },
- delete(id) {
- logger.log("delete: " + id);
- let item = _.find(items.list, {
- "id": id });
- view.remove(item.$el);
- this.list = _.pull(this.list, item);
- this.trigger("updated");
- },
- toggle(id) {
- _.find(items.list, {
- "id": id }).
- toggle();
- this.trigger("updated");
- } };
- const app = {
- init() {
- view.init();
- Object.assign(items, Backbone.Events);
- items.on("itemAdded", function (item) {
- logger.log("item added");
- view.addToList(item);
- });
- items.on("updated", function () {
- logger.log("updated");
- view.updateQuantities();
- });
- } };
- const view = {
- init() {
- this.$shoppingList = $("#shopping-list");
- this.$boughtList = $("#bought-list");
- this.$form = $("form");
- const handleSubmit = function (e) {
- e.preventDefault();
- let name = $("#item-input"),
- quantity = $("#quantity-input");
- if (name.val()) {
- items.add({
- name: name.val(),
- quantity: quantity.val() || 1 });
- }
- name.val("");
- quantity.val("");
- };
- const handleClick = function (e) {
- e.preventDefault();
- logger.log("Clicked");
- if (e.target.nodeName === "LI") {
- let id = $(e.target).data("id").toString();
- items.toggle(id);
- } else if (e.target.className === classNames.DELETE) {
- let id = $(e.target).parent().data("id").toString();
- items.delete(id);
- }
- };
- const handleDelete = function (e) {
- e.preventDefault();
- logger.log("Delete: " + item);
- let id = $(e.target).data("id").toString(),
- item = _.find(items.list, {
- "id": id });
- };
- $("#lists").on("click", handleClick);
- this.$form.on("submit", handleSubmit);
- $("." + classNames.DELETE).on("click", handleDelete);
- },
- addToList(item, list) {
- let $item = item.$el || this.createListItem(item);
- if (item.bought) {
- $item.prependTo(this.$boughtList);
- } else {
- $item.appendTo(this.$shoppingList);
- }
- },
- updateQuantities() {
- logger.log("updateQuantities");
- $("#shopping-num").html(this.$shoppingList.children().length);
- $("#bought-num").html(this.$boughtList.children().length);
- },
- remove($el) {
- $el.remove();
- },
- createListItem(item) {
- item.$el = $(`<li data-id=${item.id}>${item.name} <span class="quantity">${item.quantity}</span><span class="delete">X</span></li>`);
- return item.$el;
- },
- getListItem(id) {
- let $el = $("li[data-id='" + id + "']");
- return $el.length ? $el : null;
- },
- render(items) {
- logger.log("render");
- this.$shoppingList.empty();
- this.$boughtList.empty();
- items.forEach(item => {
- let $item = $(`<li data-id=${item.id}>${item.name}<span>${item.quantity}</span></li>`);
- if (item.bought) {
- this.$boughtList.append($item);
- $item.addClass("bought");
- } else {
- this.$shoppingList.append($item);
- }
- });
- } };
- app.init();
- items.add({
- name: "Wine",
- quantity: 1 });
- items.add({
- name: "Cheese",
- quantity: 1 });
- items.add({
- name: "Dark chocolate",
- quantity: 1 });
That’s all! hopefully, you have successfully integrated this grocery app into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
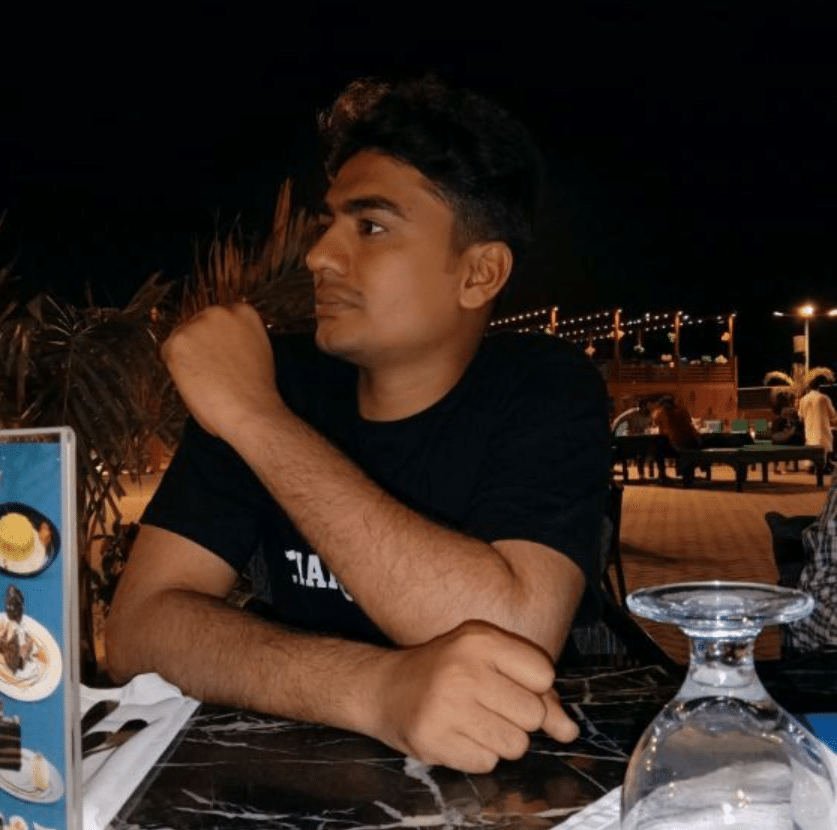
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.