This JavaScript code provides a way to resize images using the HTML canvas element. It allows you to specify a new width and/or height for an image, and it will create a resized version of the image on the canvas. This functionality is helpful for adjusting image dimensions without altering the original image file.
You can use this code in web applications to dynamically resize images. It’s beneficial for creating responsive websites, optimizing image sizes for faster loading, and providing users with downloadable resized images.
How to Create Resize Image Functionality in Javascript Using Canvas
1. In the first step, load the Normalize CSS and Google Fonts by adding the following CDN links into the head tag of your webpage. (Optional)
- <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css">
- <link rel='stylesheet' href='https://fonts.googleapis.com/css?family=Dosis'>
2. Create the HTML structure to resize the image dynamically. Include an <h2>
element for a title, an <a>
tag for image credit, a <canvas>
element to display the resized image, and a button with the class “download” to allow users to download the resized image. Update the imagePath
variable with the actual path of your image that you want to resize.
- <h2>Resizing Images in JavaScript</h2>
- <p>Image Credit: <a href="https://pixabay.com/photos/tulips-flowers-field-sky-6897351/">Pixabay</a></p>
- <div><canvas id="canvas"></canvas></div>
- <div><button class="download">Download Image</button></div>
- <script>
- imagePath = "path/to/your-image.jpg";
- </script>
3. The following CSS code ensures proper styling for your HTML elements. It defines basic styles for various elements like body, canvas, input, and buttons. You can modify the CSS code according to your project requirements.
- * {
- padding: 0;
- margin: 0;
- boxz-sizing: border-box;
- }
- body {
- margin: 20px auto;
- font-family: 'Dosis';
- font-weight: 300;
- text-align: center;
- font-size: 1rem;
- }
- canvas {
- margin: 20px auto;
- }
- input {
- border: none;
- border-bottom: 1px solid black;
- font-family: 'Dosis';
- margin: 1rem;
- text-align: center;
- outline: none;
- width: 200px;
- }
- button {
- background: yellowgreen;
- border: 2px solid black;
- color: black;
- border-radius: 5px;
- padding: 1rem 2rem;
- cursor: pointer;
- font-family: 'Dosis';
- }
- h2 {
- margin: 1rem 0;
- }
- div.wrapper {
- display: flex;
- flex-direction: column;
- }
- form {
- display: flex;
- justify-content: center;
- }
- label {
- margin: 0 1rem;
- color: gray;
- }
4. Finally, use the following JavaScript code to resize images dynamically. The resizeImage
function takes three parameters: imagePath
(the path to your image), newWidth
, and newHeight
. It resizes and displays the image on the canvas element. Make sure to call this function with the desired parameters to resize your image.
- //resize and draw the image on first load
- resizeImage(imagePath, 360, 240);
- //resize the image and draw it to the canvas
- function resizeImage(imagePath, newWidth, newHeight) {
- //create an image object from the path
- const originalImage = new Image();
- originalImage.src = imagePath;
- //get a reference to the canvas
- const canvas = document.getElementById('canvas');
- const ctx = canvas.getContext('2d');
- //wait for the image to load
- originalImage.addEventListener('load', function() {
- //get the original image size and aspect ratio
- const originalWidth = originalImage.naturalWidth;
- const originalHeight = originalImage.naturalHeight;
- const aspectRatio = originalWidth/originalHeight;
- //if the new height wasn't specified, use the width and the original aspect ratio
- if (newHeight === undefined) {
- //calculate the new height
- newHeight = newWidth/aspectRatio;
- newHeight = Math.floor(newHeight);
- //update the input element with the new height
- hInput.placeholder = `Height (${newHeight})`;
- hInput.value = newHeight;
- }
- //set the canvas size
- canvas.width = newWidth;
- canvas.height = newHeight;
- //render the image
- ctx.drawImage(originalImage, 0, 0, newWidth, newHeight);
- });
- }
- const downloadBtn = document.querySelector("button.download");
- //a click event handler for the download button
- //download the resized image to the client computer
- downloadBtn.addEventListener('click', function() {
- //create a temporary link for the download item
- let tempLink = document.createElement('a');
- //generate a new filename
- let fileName = `image-resized.jpg`;
- //configure the link to download the resized image
- tempLink.download = fileName;
- tempLink.href = document.getElementById('canvas').toDataURL("image/jpeg", 0.9);
- //trigger a click on the link to start the download
- tempLink.click();
- });
That’s all! hopefully, you have successfully integrated this JavaScript code to resize image using Canvas element. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
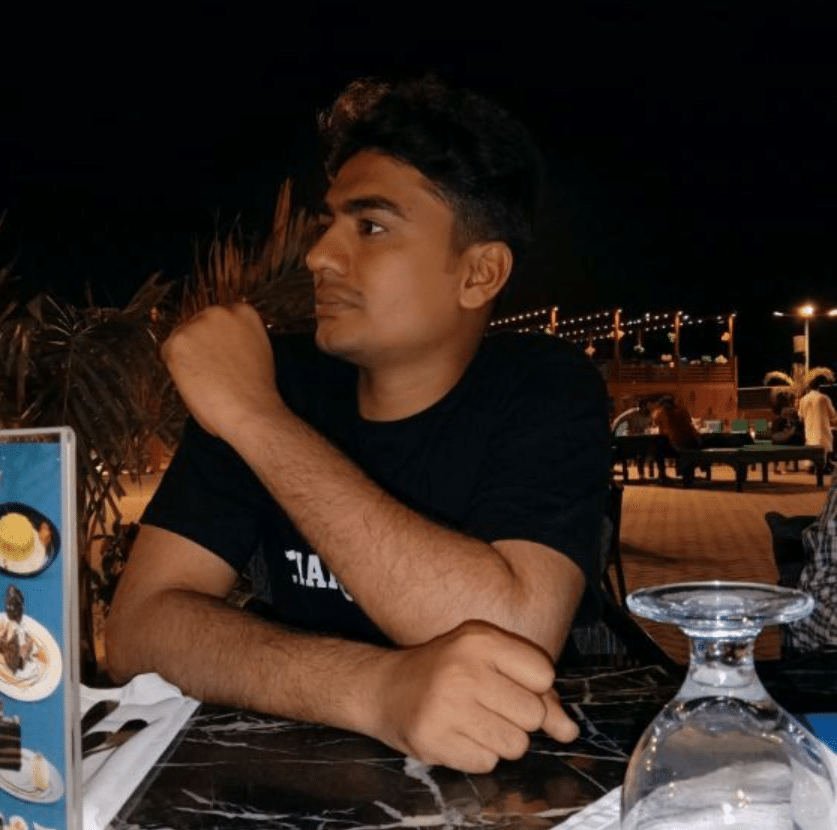
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.