This JavaScript code snippet helps you to dynamically change button text onClick event. The `changeText`
function, triggered by the button click, intelligently toggles between a default and substitute text.
The code also ensures compatibility across browsers and seamlessly updates the button’s text, providing a simple yet effective way to engage your audience.
You can integrate this interactive button that dynamically changes its text upon a click. This feature is particularly useful in forms, or any scenario where you want to guide users through different stages or actions.
How to Change Button Text Onclick in JavaScript
1. Start by adding a button element to your HTML code. For example:
- <p>Click the button to change it's text.</p>
- <button class="btn" type="button">Change</button>
2. You can customize the button’s appearance using CSS. The provided code includes some basic styling; feel free to modify it to match your website’s design.
- body {
- background: #ACC6E0;
- }
- p {
- margin-top: 2em;
- text-align: center;
- }
- p,
- .btn {
- font-family: Roboto;
- }
- .btn {
- position: relative;
- width: 6em;
- height: 2em;
- padding: 0.2em ;
- margin: 3% 0;
- left: 50%;
- transform: translateX(-50%);
- font-size: 1.2em;
- color: #eee;
- background-color: #1B6CBD;
- border: 1px solid #0E3A59;
- border-radius: 0.2em;
- box-shadow: 0 1px 0 #0E3A59,
- 0 0 2px rgba(#1B6CBD, 0.8);
- text-shadow: 0.05em 0.05em 0 #555;
- }
- .btn,
- .btn:hover {
- transition: background 150ms;
- }
- .btn:hover,
- .btn:active {
- color: #eee;
- background: #114C8F;
- border-color: #0E3A59;
- }
3. Finally, copy and paste the JavaScript code into your script file or inline script tag. Ensure the script is placed after your HTML content or inside the <body>
tag.
- /**
- * Change the text of a button
- * @param {el} object HTMLElement: button to change text of
- * @param {dText} string: default text
- * @param {nText} string: new text
- **/
- function changeText(el, dText, nText) {
- var content = '',
- context = '';
- /**
- * Set the text of a button
- * - dependant upon current text
- **/
- function setText() {
- return (content === dText) ? nText : dText;
- }
- /* Check to see if the browser accepts textContent */
- if ('textContent' in document.body) {
- context = 'textContent';
- /* Get the current button text */
- content = el[context];
- /* Browser does NOT use textContent */
- } else {
- /* Get the button text with fallback option */
- content = el.firstChild.nodeValue;
- }
- /* Set button text */
- if (context === 'textContent') {
- el.textContent = setText();
- } else {
- el.firstChild.nodeValue = setText();
- }
- }
- var defaultText,
- substituteText,
- btn;
- /* Default text of the button */
- defaultText = 'Change';
- /* Alternate text for button */
- substituteText = 'Revert';
- /* Grab our button */
- btn = document.querySelector('.btn');
- /* Add a listener to the button instance so we can manipulate it */
- btn.addEventListener('click', function() {
- changeText(this, defaultText, substituteText);
- }, false);
Adjust the defaultText
and substituteText
variables in the JavaScript code according to your needs. These represent the initial and alternate text for the button.
That’s all! hopefully, you have successfully created a functionality to update Button Text dynamically. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
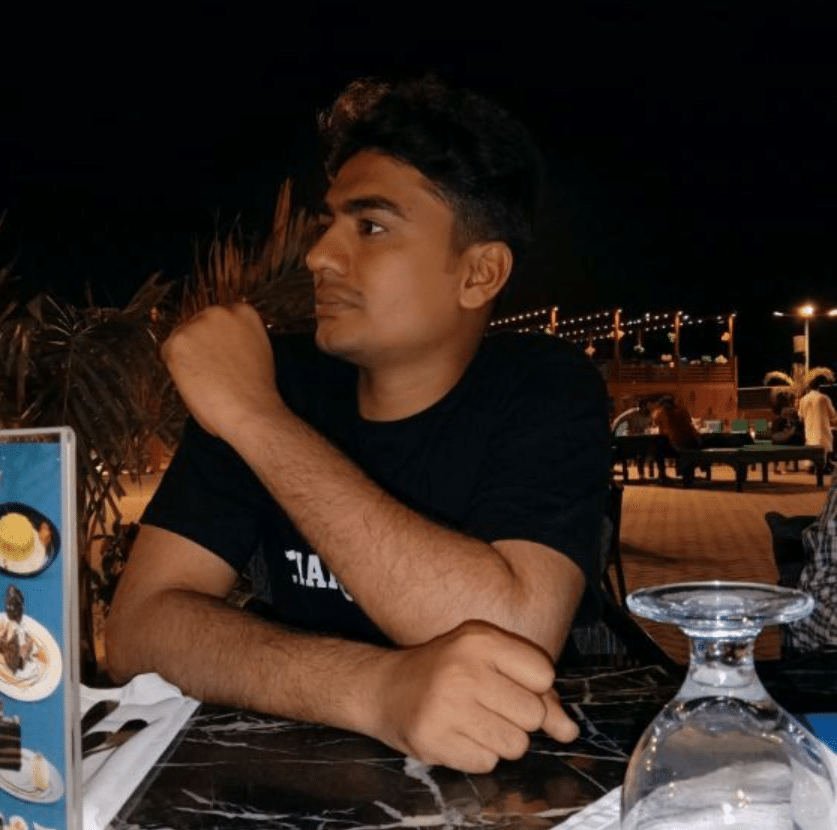
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.