This Vanilla JavaScript code snippet helps you to create autocomplete suggestion dropdown. It uses JavaScript regular expression pattern to match the entered value on keyup event. After matching, it appends the result to the suggestions list and highlights both the first letter and all matches in the suggested keywords.
You just need to update your keywords in the array according to your select menu. Then this plugin will search and load the relevant result in the suggestions list. Besides this, you can customize/style the select dropdown with additional CSS according to your needs.
How to Create JavaScript Autocomplete Dropdown
1. First of all, create a div element with the class name “search-container” and place an input element for the search box. Likewise, create a div element just after the input and define its class name “suggestions”. Place an unordered list (ul element) inside the suggestions dropdown, it will contain the search items. So, the complete HTML structure for the autocomplete dropdown is as follows:
- <div class="search-container">
- <input type="text" name="fruit" id="fruit" placeholder="Search fruit 🍎">
- <div class="suggestions">
- <ul></ul>
- </div>
- </div>
2. After creating the HTML, we need to style the input box and suggestions dropdown list. So, add the following CSS styles to your project. You can also use your own CSS styles in order to customize the autocomplete dropdown according to your existing website template.
input { border: 0 none; } .search-container { width: 400px; position: relative; margin: 15px auto; } .search-container input, .search-container .suggestions { width: 100%; background: #fff; text-align: left; } .search-container input { background: rgba(255, 255, 255, 0.2); height: 60px; padding: 0 10px; } .search-container .suggestions { position: absolute; top: 60px; } ul { display: none; list-style-type: none; padding: 0; margin: 0; box-shadow: 0 1px 2px 0 rgba(0, 0, 0, 0.2); max-height: 200px; overflow-y: auto; } ul.has-suggestions { display: block; } ul li { padding: 10px; cursor: pointer; background: rgba(255, 255, 255, 0.2); } ul li:hover { background-color: #e65c00; } input { border-bottom: 2px solid #e65c00; }
3. Finally, add the following autocomplete dropdown function between the <script> before closing the body tag. In the below function, you just need to update fruit array values. You can read more about JavaScript arrays that may help in regards to defining your own values.
- const input = document.querySelector('#fruit');
- const suggestions = document.querySelector('.suggestions ul');
- const fruit = [ 'Apple', 'Apricot', 'Avocado 🥑', 'Banana', 'Bilberry', 'Blackberry', 'Blackcurrant', 'Blueberry', 'Boysenberry', 'Currant', 'Cherry', 'Coconut', 'Cranberry', 'Cucumber', 'Custard apple', 'Damson', 'Date', 'Dragonfruit', 'Durian', 'Elderberry', 'Feijoa', 'Fig', 'Gooseberry', 'Grape', 'Raisin', 'Grapefruit', 'Guava', 'Honeyberry', 'Huckleberry', 'Jabuticaba', 'Jackfruit', 'Jambul', 'Juniper berry', 'Kiwifruit', 'Kumquat', 'Lemon', 'Lime', 'Loquat', 'Longan', 'Lychee', 'Mango', 'Mangosteen', 'Marionberry', 'Melon', 'Cantaloupe', 'Honeydew', 'Watermelon', 'Miracle fruit', 'Mulberry', 'Nectarine', 'Nance', 'Olive', 'Orange', 'Clementine', 'Mandarine', 'Tangerine', 'Papaya', 'Passionfruit', 'Peach', 'Pear', 'Persimmon', 'Plantain', 'Plum', 'Pineapple', 'Pomegranate', 'Pomelo', 'Quince', 'Raspberry', 'Salmonberry', 'Rambutan', 'Redcurrant', 'Salak', 'Satsuma', 'Soursop', 'Star fruit', 'Strawberry', 'Tamarillo', 'Tamarind', 'Yuzu'];
- function search(str) {
- let results = [];
- const val = str.toLowerCase();
- for (i = 0; i < fruit.length; i++) {
- if (fruit[i].toLowerCase().indexOf(val) > -1) {
- results.push(fruit[i]);
- }
- }
- return results;
- }
- function searchHandler(e) {
- const inputVal = e.currentTarget.value;
- let results = [];
- if (inputVal.length > 0) {
- results = search(inputVal);
- }
- showSuggestions(results, inputVal);
- }
- function showSuggestions(results, inputVal) {
- suggestions.innerHTML = '';
- if (results.length > 0) {
- for (i = 0; i < results.length; i++) {
- let item = results[i];
- // Highlights only the first match
- // TODO: highlight all matches
- const match = item.match(new RegExp(inputVal, 'i'));
- item = item.replace(match[0], `<strong>${match[0]}</strong>`);
- suggestions.innerHTML += `<li>${item}</li>`;
- }
- suggestions.classList.add('has-suggestions');
- } else {
- results = [];
- suggestions.innerHTML = '';
- suggestions.classList.remove('has-suggestions');
- }
- }
- function useSuggestion(e) {
- input.value = e.target.innerText;
- input.focus();
- suggestions.innerHTML = '';
- suggestions.classList.remove('has-suggestions');
- }
- input.addEventListener('keyup', searchHandler);
- suggestions.addEventListener('click', useSuggestion);
That’s all! hopefully, you have successfully integrated this code snippet into your project to create autocomplete dropdown. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
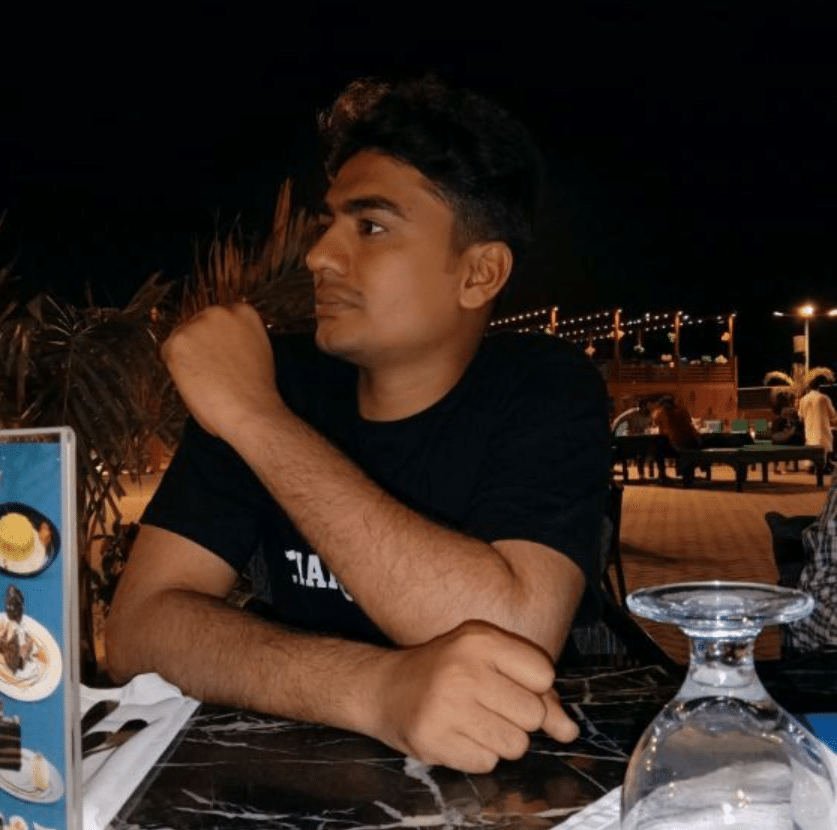
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.