This JavaScript code snippet helps you to create accessible vertical tabs for easy content navigation. It organizes content into tabs. It enables switching between content sections by clicking. The method ensures active tab visibility while hiding others, aiding content accessibility.
You can use this code in websites or web applications requiring organized content sections. It simplifies navigation by grouping related information into tabs. It enhances user experience by allowing easy access to specific content without overwhelming the interface.
How to Create Accessible Vertical Tabs in JavaScript
1. Start by structuring your HTML to define the content and tabs. Use <ul>
for indexes and tabs, each associated with specific content. Customize the content inside each tab.
- <main>
- <header>
- <h2><span>Table</span> of content</h2>
- </header>
- <section>
- <ul class='indexes'>
- <li data-index='0'>01</li>
- <li data-index='1'>02</li>
- <li data-index='2'>03</li>
- <li data-index='3'>04</li>
- </ul>
- <ul class='tabs'>
- <li class='tab'>
- <article class='tab-content'>
- <h3>Midnight Station</h3>
- <p>Lorem ipsum dolor, sit amet consectetur adipisicing elit.
- Voluptas nihil sequi doloribus obcaecati. Aut vel, recusandae ipsa
- voluptate blanditiis nemo magnam sit modi architecto officia
- maiores magni. Necessitatibus, iste aut.</p>
- <button>Read More</button>
- </article>
- <div class='tab-image'><img src='https://picsum.photos/id/345/1000/600'></div>
- </li>
- <li class='tab'>
- <article class='tab-content'>
- <h3>The Hitchhiker</h3>
- <p>Lorem ipsum dolor, sit amet consectetur adipisicing elit.
- Voluptas nihil sequi doloribus obcaecati. Aut vel, recusandae ipsa
- voluptate blanditiis nemo magnam sit modi architecto officia
- maiores magni. Necessitatibus, iste aut.</p>
- <button>Read More</button>
- </article>
- <div class='tab-image'><img src='https://picsum.photos/id/352/1000/600'></div>
- </li>
- <li class='tab'>
- <article class='tab-content'>
- <h3>Missing Pages</h3>
- <p>Lorem ipsum dolor, sit amet consectetur adipisicing elit.
- Voluptas nihil sequi doloribus obcaecati. Aut vel, recusandae ipsa
- voluptate blanditiis nemo magnam sit modi architecto officia
- maiores magni. Necessitatibus, iste aut.</p>
- <button>Read More</button>
- </article>
- <div class='tab-image'><img src='https://picsum.photos/id/444/1000/600'></div>
- </li>
- <li class='tab'>
- <article class='tab-content'>
- <h3>Uninvited Guests</h3>
- <p>Lorem ipsum dolor, sit amet consectetur adipisicing elit.
- Voluptas nihil sequi doloribus obcaecati. Aut vel, recusandae ipsa
- voluptate blanditiis nemo magnam sit modi architecto officia
- maiores magni. Necessitatibus, iste aut.</p>
- <button>Read More</button>
- </article>
- <div class='tab-image'><img src='https://picsum.photos/id/451/1000/600'></div>
- </li>
- </ul>
- </section>
- </main>
2. Now, apply CSS styles to make the tabs visually appealing and ensure they align properly. Customize the styling by adjusting CSS variables like --primary
for color and --overlay
for overlay transparency. Modify the CSS rules to match your overall website theme and branding.
- :root {
- --primary: #d32626;
- --overlay: rgba(211, 38, 38, 0.6);
- }
- * {
- margin: 0;
- padding: 0;
- box-sizing: border-box;
- }
- body {
- height: 100vh;
- display: grid;
- place-items: center;
- background-color: black !important;
- color: rgba(255, 255, 255, 0.85);
- }
- main {
- width: 600px;
- height: 300px;
- font: 0.7rem impact, sans-serif;
- }
- main header {
- font-size: 1.2rem;
- text-transform: uppercase;
- margin-bottom: 2.25rem;
- color: white;
- }
- main header span {
- color: var(--primary);
- }
- main section {
- display: flex;
- gap: 2rem;
- }
- .indexes,
- .tabs {
- list-style-type: none;
- }
- .indexes {
- font-size: 1rem;
- }
- .indexes li {
- padding: 1rem;
- border: 1px solid transparent;
- cursor: pointer;
- }
- .tabs {
- position: relative;
- }
- .tab {
- position: absolute;
- display: flex;
- width: 530px;
- height: 225px;
- opacity: 0;
- background-color: black;
- overflow: hidden;
- }
- .tab-content {
- position: relative;
- z-index: 5;
- width: 300px;
- display: flex;
- flex-direction: column;
- justify-content: space-between;
- gap: 1rem;
- opacity: 0;
- transform: translateY(-5rem);
- }
- .tab-content h3 {
- font-family: helvetica;
- font-weight: 900;
- font-size: 1rem;
- border-bottom: 1.5px solid white;
- padding-bottom: 1rem;
- }
- .tab-content p {
- font-family: helvetica;
- font-weight: 100;
- line-height: 2;
- color: rgba(255, 255, 255, 0.7);
- }
- .tab-content button {
- width: fit-content;
- background-color: transparent;
- color: white;
- border: 1px solid white;
- font-size: 0.7rem;
- padding: 0.75rem 1rem;
- cursor: pointer;
- }
- @keyframes content {
- 100% {
- opacity: 1;
- transform: translateY(0);
- }
- }
- .tab-image {
- position: absolute;
- right: 1rem;
- width: 200px;
- height: 200px;
- opacity: 0;
- transform: translateX(2rem);
- }
- .tab-image::after {
- content: '';
- position: absolute;
- inset: 0;
- background-color: var(--overlay);
- mix-blend-mode: multiply;
- }
- .tab-image img {
- width: inherit;
- height: inherit;
- object-fit: cover;
- filter: grayscale(100%);
- }
- @keyframes image {
- 100% {
- opacity: 1;
- width: 300px;
- transform: translateX(0);
- }
- }
- .active .tab {
- opacity: 1;
- z-index: 5;
- }
- .active .tab-content {
- animation: content 0.9s ease-out 0.4s forwards;
- }
- .active .tab-image {
- animation: image 1s ease-out forwards;
- }
3. Finally, include the following JavaScript code in your project, ensuring it’s loaded after the DOM has fully loaded. Use the indexes
and tabs
variables to reference your index list and tab elements.
- const indexes = document.querySelectorAll('.indexes li');
- const tabs = document.querySelectorAll('.tab');
- const contents = document.querySelectorAll('.tab-content');
- function reset() {
- for (let i = 0; i < tabs.length; i++) {
- indexes[i].style.borderColor = 'transparent';
- tabs[i].style.zIndex = 0;
- tabs[i].classList.remove('active');
- contents[i].classList.remove('active');
- }
- }
- function showTab(i) {
- indexes[i].style.borderColor = 'rgba(211,38,38,0.6)';
- tabs[i].style.opacity = 1;
- tabs[i].style.zIndex = 5;
- tabs[i].classList.add('active');
- contents[i].classList.add('active');
- }
- function activate(e) {
- if (!e.target.matches('.indexes li')) return;
- reset();
- showTab(e.target.dataset.index);
- }
- const init = () => showTab(0);
- window.addEventListener('load',init,false);
- window.addEventListener('click',activate,false);
That’s all! hopefully, you have successfully created accessible vertical tabs in JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
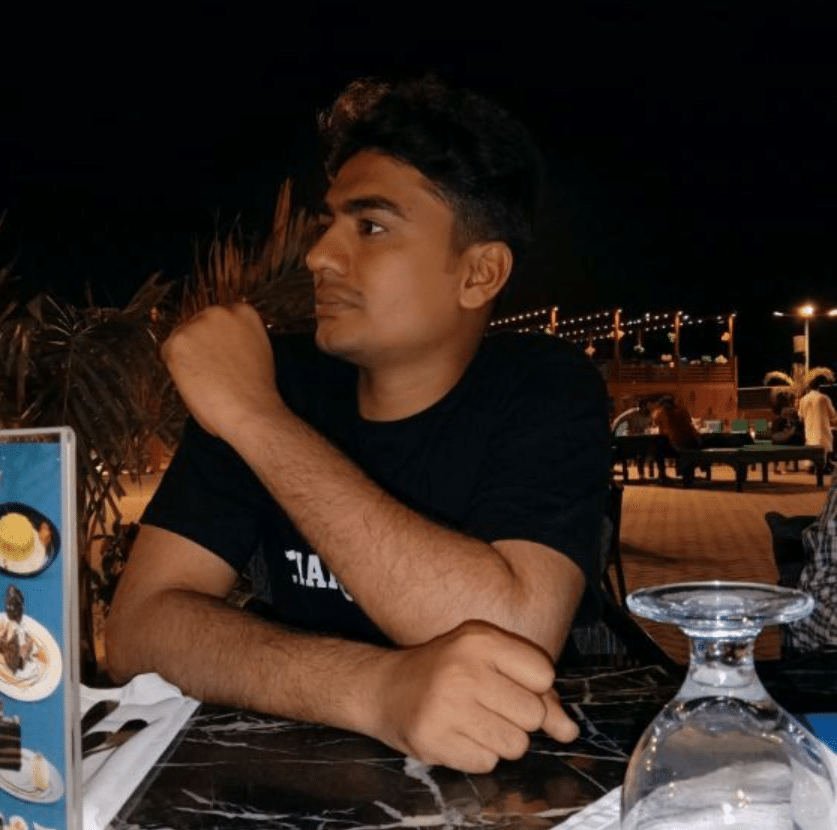
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.