Yet another text typing and erasing animation created in CSS and JavaScript. It gets text strings stored in an array and animates with a specific time period with typing effect. You can easily integrate this code snippet to create typing effect with a custom interval.
How to Create Typing and Erasing Animation in CSS and JavaScript
1. First of all, create the HTML structure as follows:
- <h1>Our recipes are
- <span
- class="txt-rotate"
- data-period="2000"
- data-rotate='[ "Healthy.", "Nutritious.", "Delicious.", "fun!" ]'></span>
- </h1>
- <h2>A single recipe is all you need.</h2>
- <!-- partial -->
- <script src="./js/script.js"></script>
2. After that, add the following CSS styles to your project:
- html,body {
- font-family: 'Raleway', sans-serif;
- font-size: 18px;
- color: #aaa
- }
- h1,h2 {
- font-weight: 200;
- margin: 0.4em 0;
- }
- h1 { font-size: 3.5em; }
- h2 {
- color: #888;
- font-size: 2em;
- }
3. Finally, add the following JavaScript code and done.
- var TxtRotate = function(el, toRotate, period) {
- this.toRotate = toRotate;
- this.el = el;
- this.loopNum = 0;
- this.period = parseInt(period, 10) || 2000;
- this.txt = '';
- this.tick();
- this.isDeleting = false;
- };
- TxtRotate.prototype.tick = function() {
- var i = this.loopNum % this.toRotate.length;
- var fullTxt = this.toRotate[i];
- if (this.isDeleting) {
- this.txt = fullTxt.substring(0, this.txt.length - 1);
- } else {
- this.txt = fullTxt.substring(0, this.txt.length + 1);
- }
- this.el.innerHTML = '<span class="wrap">'+this.txt+'</span>';
- var that = this;
- var delta = 300 - Math.random() * 100;
- if (this.isDeleting) { delta /= 2; }
- if (!this.isDeleting && this.txt === fullTxt) {
- delta = this.period;
- this.isDeleting = true;
- } else if (this.isDeleting && this.txt === '') {
- this.isDeleting = false;
- this.loopNum++;
- delta = 500;
- }
- setTimeout(function() {
- that.tick();
- }, delta);
- };
- window.onload = function() {
- var elements = document.getElementsByClassName('txt-rotate');
- for (var i=0; i<elements.length; i++) {
- var toRotate = elements[i].getAttribute('data-rotate');
- var period = elements[i].getAttribute('data-period');
- if (toRotate) {
- new TxtRotate(elements[i], JSON.parse(toRotate), period);
- }
- }
- // INJECT CSS
- var css = document.createElement("style");
- css.type = "text/css";
- css.innerHTML = ".txt-rotate > .wrap { border-right: 0.08em solid #666 }";
- document.body.appendChild(css);
- };
That’s all! hopefully, you have successfully integrated this typing and erasing animation code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
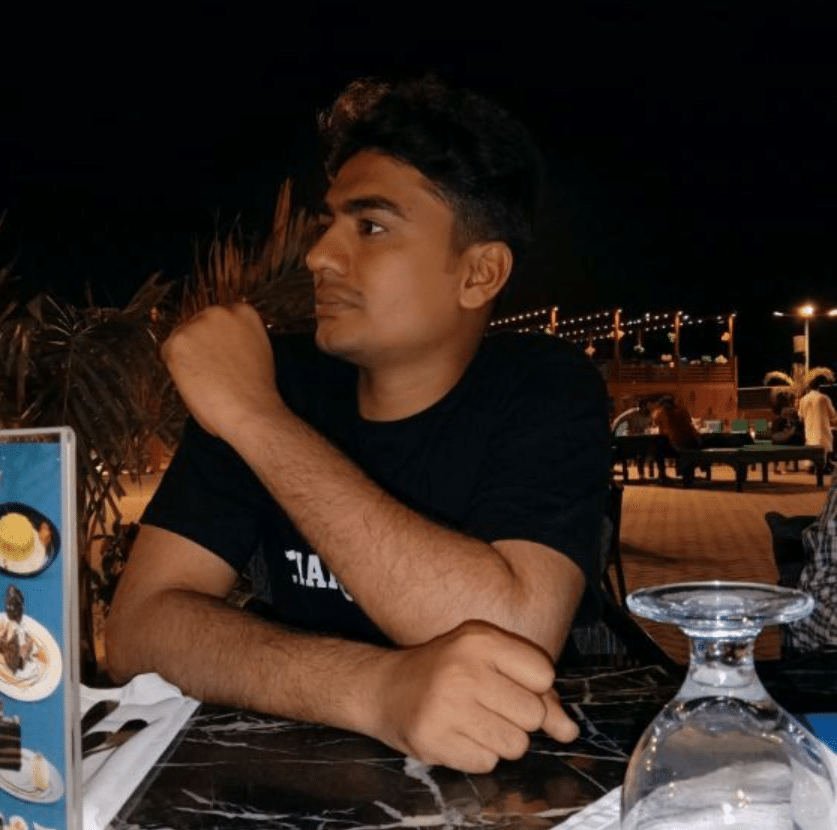
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
is their any way i can change the word on specific button click