The Poppa is a lightweight, easy to use and powerful jQuery form validation plugin. It lets you to validate existing HTML forms or required field validation on jQuery submit event. The main purpose of this plugin is to validate client-side input fields on submit or before submit (live validation). It comes with multiple configuration options to validate various type of required fields.
Moreover, the plugin can be customize with additional CSS to fully fit on your needs.
How to Use jQuery Required Field Validation
1. First of all load jQuery JavaScript library and validation Poppa plugin’s CSS & JavaScript files into your HTML document.
<!-- jQuery --> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <!-- Poppa Validation JS --> <script src="js/validation_new.js"></script> <!-- Poppa Validation CSS --> <link rel="stylesheet" type="text/css" href="css/validation.css">
2. After that, create your HTML form with different input fields and define validation methods.
<form action="" class="user-registration mb-3"> <h6 class="title mb-2 mt-0">User registration</h6> <div class="form-group"> <label>Login *</label> <input name="login" type="text" data-validation-length="min:3" class="form-control" required> </div> <div class="form-group"> <label>Email *</label> <input name="email" type="email" class="form-control" required> </div> <div class="form-group"> <label>Password *</label> <input name="password" type="password" class="form-control" required> </div> <div class="form-group"> <label>Website</label> <input name="website" data-validation-type="url" class="form-control"> </div> <div class="form-group"> <label>Bio</label> <textarea name="bio" data-validation-length="min:10,max:150" data-validation-hint="Describe yourself" class="form-control"></textarea> </div> <div class="form-group"> <label class="mb-0">I agree to the terms of service *</label> <input type="checkbox" data-validation-message="You have to agree to terms of service" required> </div> <div class="mb-2"><small>* required fields</small></div> <button type="submit" class="btn btn-primary">Submit</button> </form>
3. And finally, initialize the plugin (with all default settings) in jQuery document ready function.
$(document).ready(function () { $('.user-registration').validation(); });
4. You can also validate required input filed of your existing HTML form. You just need to call plugin with your form. Like:
$('#your-form').validation();
Advance Configuration Options for Required Field Validation Plugin
The following are some advance configuration options to create / customize “jQuery required field validation on submit”.
Option | Description, Default, Type |
---|---|
preventDefault |
Enable / disable prevent default form from submitting. Default: true, Type: Boolean.
$('#your-form').validation({ preventDefault: true, }); |
sendForm |
Enable / disable prevents form from submitting even if provided data is valid. Default: true, Type: Boolean.
$('#your-form').validation({ sendForm: true, }); |
autocomplete |
Enable / diable auto complete functionality of input fields. Default: ‘on’, Type: String.
Available Option: ‘on’ | ‘off’ $('#your-form').validation({ autocomplete: 'on', }); |
noValidate |
This option is useful to disable HTML5 default form validation. Default: true, Type: Boolean.
$('#your-form').validation({ noValidate: true, }); |
liveValidation |
If you want to validate your form’s inputs before submit, then this option might be useful for you. Default: true, Type: Boolean.
$('#your-form').validation({ liveValidation: true, }); |
Similar Code Snippets:
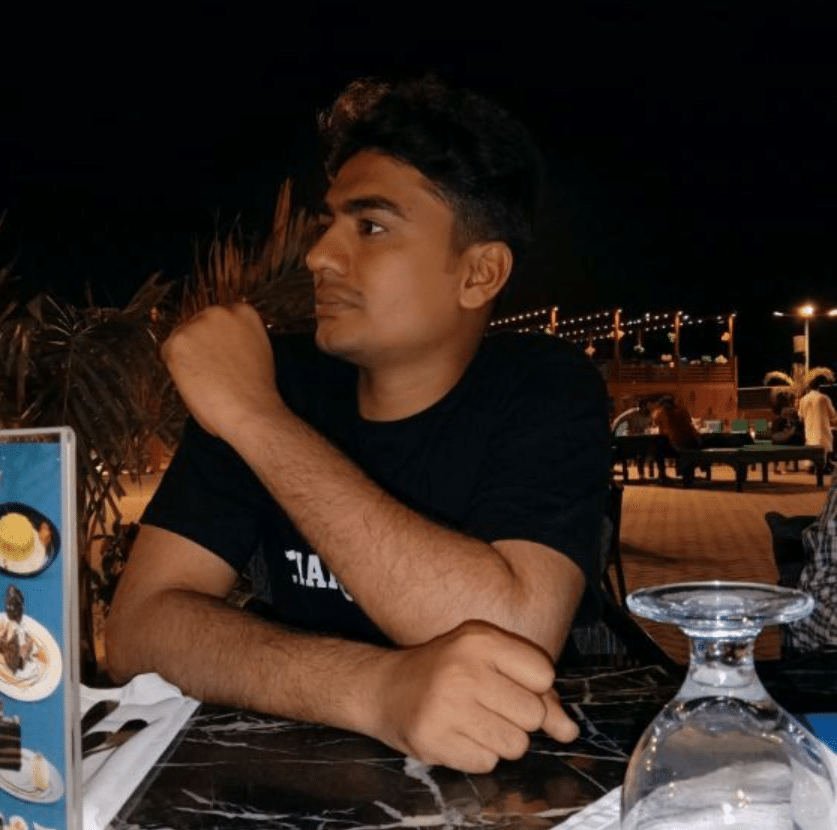
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.