This JavaScript code facilitates checking multiple checkboxes by holding Shift. When a user clicks a checkbox, holds Shift, and clicks another checkbox further down, it automatically checks all the checkboxes in between.
The script employs event listeners to detect checkbox clicks and uses the Shift key to determine the range for multiple selections, enhancing the user experience in selecting items quickly and efficiently.
How to Create “Check Multiple Checkboxes” Functionality In JavaScript
1. First, let’s set up our HTML structure, which includes a list of checkboxes that users can select.
- <h1>Check Multiple Checkboxes</h1>
- <h2>Check one item, hold down SHIFT, check another item</h2>
- <div class="inbox post-it-note">
- <div class="item">
- <input type="checkbox">
- <p>Message</p>
- </div>
- <div class="item">
- <input type="checkbox">
- <p>Message</p>
- </div>
- <div class="item">
- <input type="checkbox">
- <p>Message</p>
- </div>
- <div class="item">
- <input type="checkbox">
- <p>Message</p>
- </div>
- <div class="item">
- <input type="checkbox">
- <p>Message</p>
- </div>
- </div>
- </div>
2. Use the following CSS code to style the checkboxes. (Optional)
- @import url("https://fonts.googleapis.com/css?family=Exo");
- html {
- background-color: #00acc1;
- font-family: 'Exo', sans-serif;
- }
- h1 {
- text-align: center;
- font-size: 32px;
- }
- h2{
- text-align: center;
- font-size: 18px;
- font-weight: 400;
- }
- .inbox {
- max-width:400px;
- margin:50px auto;
- background:white;
- border-radius:5px;
- box-shadow:10px 10px 0 rgba(0,0,0,0.1);
- }
- .post-it-note {
- padding: 2em;
- background: #ffd707;
- position: relative;
- min-height: 10em;
- }
- .post-it-note:after {
- content: "";
- position: absolute;
- bottom: -2em;
- left: 0;
- right: 2em;
- border-width: 1em;
- border-style: solid;
- border-color: #ffd707;
- }
- .post-it-note:before {
- content: "";
- position: absolute;
- bottom: -2em;
- right: 0;
- border-width: 2em 2em 0 0;
- border-style: solid;
- border-color: #d3b100 transparent;
- }
- .item {
- display:flex;
- align-items:center;
- border-bottom: 1px solid #F1F1F1;
- }
- .item:last-child {
- border-bottom:0;
- }
- input:checked + p {
- background: #ffd707;
- text-decoration: line-through;
- }
- input[type="checkbox"] {
- margin:20px;
- }
- p {
- margin:0;
- padding:20px;
- transition:background 0.2s;
- flex:1;
- font-family:'helvetica neue';
- font-size: 20px;
- font-weight: 200;
- border-left: 1px solid #D1E2FF;
- }
3. Finally, add the following JavaScript code to your project. It adds the functionality to check multiple checkboxes using the Shift key. When a user clicks a checkbox, holds Shift, and clicks another checkbox further down, all checkboxes in between are automatically checked.
- const checkboxes = document.querySelectorAll('.inbox input[type="checkbox"]');
- let lastChecked;
- function handleCheck(e) {
- // Check if they had the shift key down
- // AND check that they are checking it
- let inBetween = false;
- if (e.shiftKey && this.checked) {
- // go ahead and do what we please
- // loop over every single checkbox
- checkboxes.forEach(checkbox => {
- console.log(checkbox);
- if (checkbox === this || checkbox === lastChecked) {
- inBetween = !inBetween;
- console.log('Starting to check them inbetween!');
- }
- if (inBetween) {
- checkbox.checked = true;
- }
- });
- }
- lastChecked = this;
- }
- checkboxes.forEach(checkbox => checkbox.addEventListener('click', handleCheck));
That’s all! hopefully, you have successfully created a functionality to check multiple checkboxes using JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
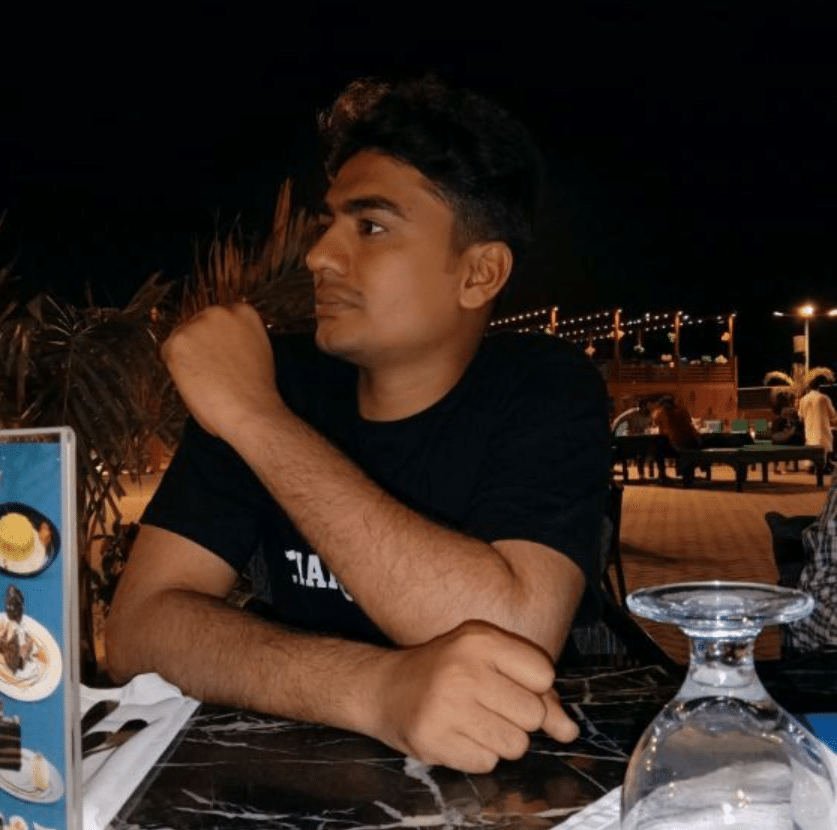
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.