This CSS and JavaScript code snippet helps you to create an animated text. It comes with calculated animations, and letters gracefully dance, forming a spellbinding visual experience. Perfect for injecting life into your web projects, this code seamlessly blends the artistry of design with the precision of code, creating an engaging display that is both eye-catching and innovative.
How to Create Animated Text Using CSS and JavaScript
1. Begin by creating a container <div>
with an id of “c1.” Inside this container, nest another <div>
with an id of “c2.” Finally, add a <p>
element within “c2” to hold the animated text. Each letter of the text is enclosed in a <span>
element with a custom style property “–t” to control the animation timing.
- <div id="c1">
- <div id="c2">
- <p>
- <span style="--t: 0.05">M</span>
- <span style="--t: 0.15">O</span>
- <span style="--t: 0.25">V</span>
- <span style="--t: 0.35">E</span>
- <span style="--t: 0.45">A</span>
- <span style="--t: 0.55">R</span>
- <span style="--t: 0.65">O</span>
- <span style="--t: 0.75">U</span>
- <span style="--t: 0.85">N</span>
- <span style="--t: 0.95">D</span>
- </p>
- </div>
- </div>
2. In CSS, import the necessary font and define the keyframe animation. The root styles set up the main animation, while individual styles for “c1” and “c2” control their specific animations. The “p” and “span” styles handle the positioning, size, and color of the animated text.
- @import url('https://fonts.googleapis.com/css2?family=Palanquin+Dark:wght@700&display=swap');
- @property --a {
- syntax: "<angle>";
- inherits: true;
- initial-value: 0deg;
- }
- @keyframes round {
- from {--a: 0deg}
- to {--a: 360deg}
- }
- :root {
- animation: round 10s linear infinite;
- --x0: 0;
- --y0: calc(cos(var(--a)) * 50 + 50);
- --x3: 100;
- --y3: calc(cos(var(--a) + 100deg) * 50 + 50);
- }
- #c1 {
- animation: round 8s linear infinite;
- --x1: calc(50 + sin(var(--a)) * 40);
- --y1: calc(50 + cos(var(--a)) * 50);
- }
- #c2 {
- animation: round 6s linear infinite;
- --x2: calc(50 + sin(var(--a)) * 40);
- --y2: calc(50 + cos(var(--a)) * 50);
- }
- body{
- background: #333 !important;
- margin: 0;
- width: 100vw;
- height: 100vh;
- overflow: hidden;
- }
- p {
- position: relative;
- width: 100%;
- margin: 0;
- height: 100%;
- font-family: 'Palanquin Dark', sans-serif;
- font-size: 6vw;
- }
- span {
- position: absolute;
- --mt: calc(1 - var(--t));
- --x: calc(var(--mt) * var(--mt) * var(--mt) * var(--x0) + 3 * var(--t) * var(--mt) * var(--mt) * var(--x1) + 3 * var(--t) * var(--t) * var(--mt) * var(--x2) + var(--t) * var(--t) * var(--t) * var(--x3));
- --y: calc(var(--mt) * var(--mt) * var(--mt) * var(--y0) + 3 * var(--t) * var(--mt) * var(--mt) * var(--y1) + 3 * var(--t) * var(--t) * var(--mt) * var(--y2) + var(--t) * var(--t) * var(--t) * var(--y3));
- left: calc(1vw * var(--x) - 2vw);
- top: calc(1vh * var(--y) - 4vh);
- color: rgb(calc((var(--y) - var(--x)) * 0.5% + 50%) calc((var(--x) - var(--y)) * 0.5% + 50%) calc(var(--x) * 1%));
- text-shadow: calc(0.2px * var(--x)) calc(0.5px * var(--y)) calc(0.1px * (var(--x) + var(--y))) #aaa;
- }
3. To ensure cross-browser compatibility, a JavaScript fallback is provided for browsers lacking support for the @property
rule. This script dynamically adjusts the animation based on the current timestamp, creating a smooth and continuous animation effect.
- /* Fallback for missing @property support in Firefox */
- if (!CSS.registerProperty) {
- const root = document.querySelector(':root');
- const c1 = root.querySelector('#c1');
- const c2 = root.querySelector('#c1');
- const zero = document.timeline.currentTime;
- function step (timeStamp) {
- const value = timeStamp - zero;
- root.style.setProperty('--a', `${(value / 10000 *360) % 360}deg`);
- c1.style.setProperty('--a', `${(value / 8000 *360) % 360}deg`);
- c2.style.setProperty('--a', `${(value / 6000 *360) % 360}deg`);
- requestAnimationFrame(step);
- }
- requestAnimationFrame(step);
- }
Feel free to customize the text within the <p>
element and experiment with the animation duration by adjusting the keyframe durations in the CSS code. You can also modify the font and colors to suit your design preferences.
That’s all! hopefully, you have successfully created Animated Text Using CSS and Javascript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
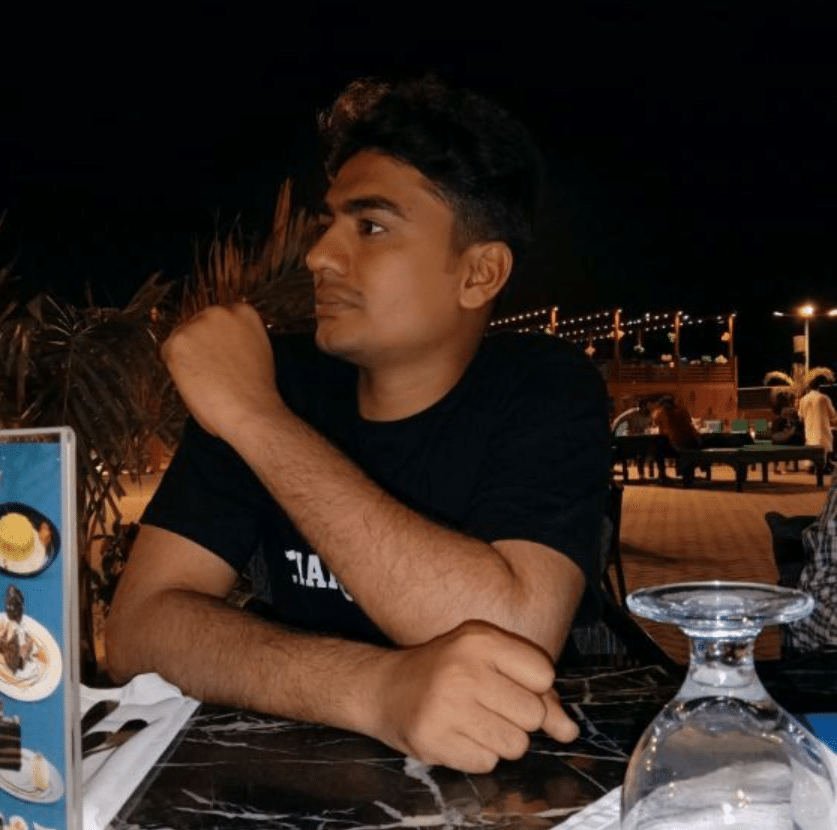
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
oewjlf