This HTML source code helps you to create a simple website visitor counter. Basically, it counts the visits for a single user using the local storage API. It checks if there is a page_view
entry in localStorage. If there is, the code increments the counter by 1 and updates the localStorage entry.
If you are working on a tracking website visit statistics project, this code snippet might be helpful for you.
How to Create a Simple HTML Visitor Counter
1. Begin by including the necessary CSS libraries in the <head>
section of your HTML file. Add the provided CDN links for the Meyer Reset and Tailwind CSS to ensure proper styling.
- <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
- <link rel='stylesheet' href='https://cdn.tailwindcss.com'>
2. Create the HTML structure for your visitor counter. Place it where you want the counter to appear. You can customize the layout and positioning according to your project’s design.
- <div>Website visit count:</div>
- <div class="website-counter"></div>
- <button class="button" id="reset">Reset</button>
3. Copy and paste the following CSS code into your project’s stylesheet. This will ensure the counter and reset button are styled appropriately.
- /* Center child elements */
- .website-counter {
- display: flex;
- justify-content: center;
- align-items: center;
- flex-direction: column;
- }
- /* Styles for website counter container */
- .website-counter {
- background-color: #ff4957;
- height: 50px;
- width: 80px;
- color: white;
- border-radius: 30px;
- font-weight: 700;
- font-size: 25px;
- margin-top: 10px;
- }
- /* Styles for reset button */
- #reset {
- margin-top: 20px;
- background-color: #008cba;
- cursor: pointer;
- font-size: 18px;
- padding: 8px 20px;
- color: white;
- border: 0;
- }
4. Finally, insert the JavaScript code at the end of your HTML file, just before the closing </body>
tag. This script handles the counter functionality and the reset button.
- var counterContainer = document.querySelector(".website-counter");
- var resetButton = document.querySelector("#reset");
- var visitCount = localStorage.getItem("page_view");
- // Check if page_view entry is present
- if (visitCount) {
- visitCount = Number(visitCount) + 1;
- localStorage.setItem("page_view", visitCount);
- } else {
- visitCount = 1;
- localStorage.setItem("page_view", 1);
- }
- counterContainer.innerHTML = visitCount;
- // Adding onClick event listener
- resetButton.addEventListener("click", () => {
- visitCount = 1;
- localStorage.setItem("page_view", 1);
- counterContainer.innerHTML = visitCount;
- });
That’s all! hopefully, you have successfully integrated this HTML Visitor Counter source code on your website. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
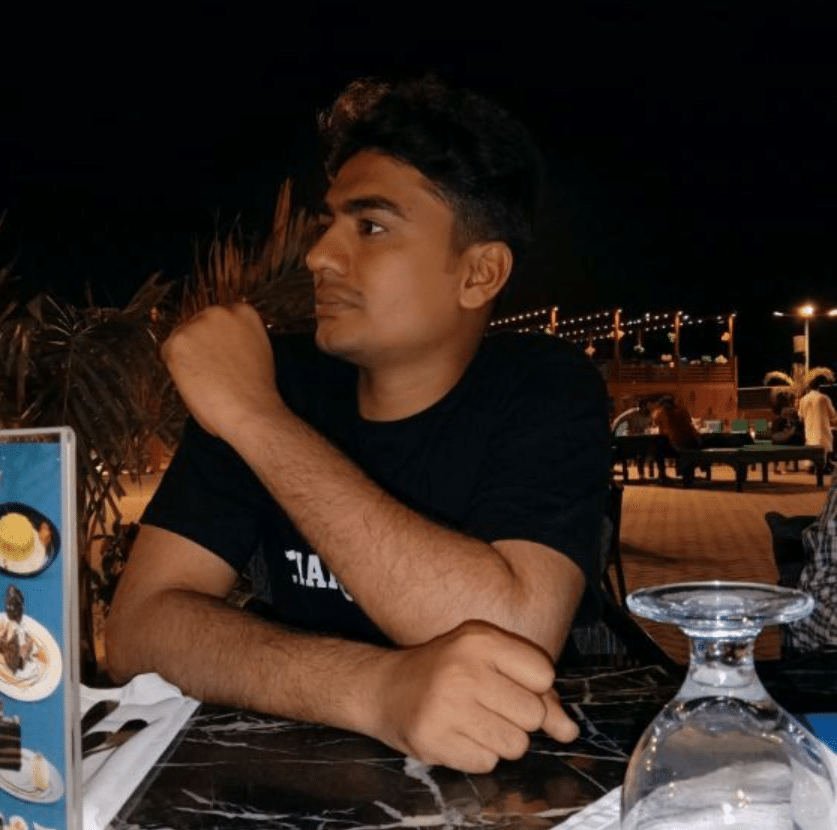
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.