This JavaScript code snippet helps you to create and open dropdown on button click event. It uses JavaScript to toggle the menu’s visibility. The `myFunction` method adds or removes a class to show or hide the dropdown. Helpful for creating interactive menus on web pages.
You can use this code for website navigation menus. It helps create interactive dropdowns, enhancing user experience.
How to Create a Dropdown that Opens On Button Click
1. In your HTML file, set up the structure for the dropdown menu using <div>
, <button>
, and <a>
tags: Add more <a>
tags inside <div class="dropdown-content">
for additional dropdown options.
- <h2>Clickable Dropdown</h2>
- <p>Click on the button to open the dropdown menu.</p>
- <div class="dropdown">
- <button id="myBtn" class="dropbtn">Dropdown</button>
- <div id="myDropdown" class="dropdown-content">
- <a href="#home">Home</a>
- <a href="#about">About</a>
- <a href="#contact">Contact</a>
- </div>
- </div>
2. Style the button and dropdown menu using CSS to achieve the desired look and feel. Modify CSS styles for personalized appearance.
- .dropbtn {
- background-color: #4CAF50;
- color: white;
- padding: 16px;
- font-size: 16px;
- border: none;
- cursor: pointer;
- }
- .dropbtn:hover,
- .dropbtn:focus {
- background-color: #3e8e41;
- }
- .dropdown {
- position: relative;
- display: inline-block;
- }
- .dropdown-content {
- display: none;
- position: absolute;
- background-color: #f9f9f9;
- min-width: 160px;
- overflow: auto;
- box-shadow: 0px 8px 16px 0px rgba(0, 0, 0, 0.2);
- }
- .dropdown-content a {
- color: black;
- padding: 12px 16px;
- text-decoration: none;
- display: block;
- }
- .dropdown-content a:hover {
- background-color: #f1f1f1;
- }
- .show {
- display: block;
- }
3. Finally, use the following JavaScript code to toggle the display of the dropdown when the button is clicked and close it if clicked outside.
- // Get the button, and when the user clicks on it, execute myFunction
- document.getElementById("myBtn").onclick = function () {myFunction();};
- /* myFunction toggles between adding and removing the show class, which is used to hide and show the dropdown content */
- function myFunction() {
- document.getElementById("myDropdown").classList.toggle("show");
- }
- // Close the dropdown if the user clicks outside of it
- window.onclick = function (event) {
- if (!event.target.matches('.dropbtn')) {
- var dropdowns = document.getElementsByClassName("dropdown-content");
- for (let i = 0; i < dropdowns.length; i++) {
- var openDropdown = dropdowns[i];
- if (openDropdown.classList.contains('show')) {
- openDropdown.classList.remove('show');
- }
- }
- }
- };
That’s all! hopefully, you have successfully created Open Dropdown On Button Click Javascript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
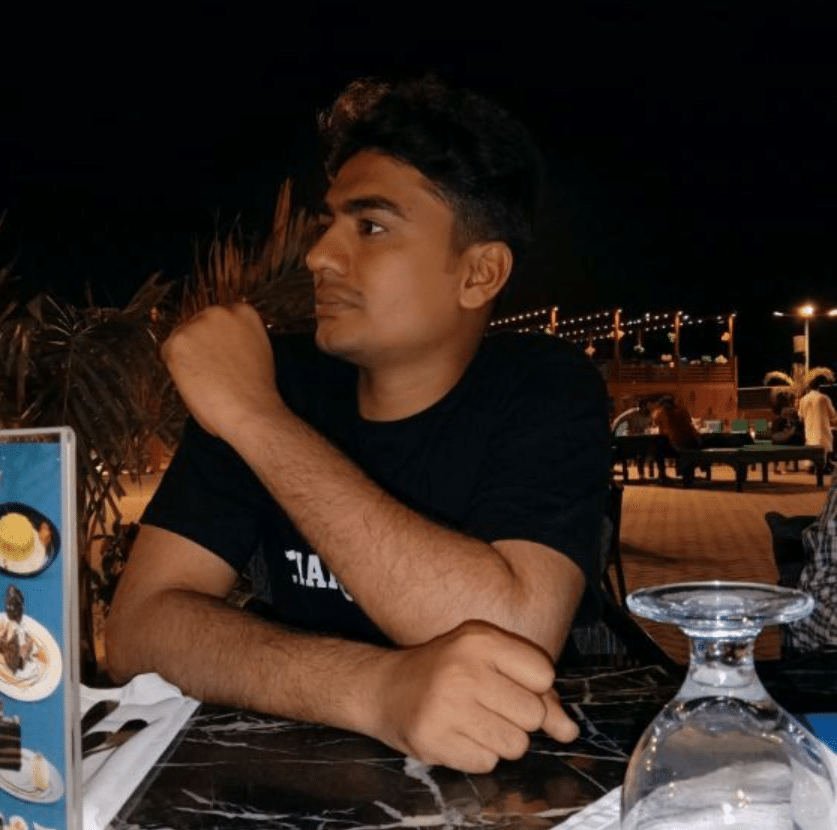
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.