This code creates an email subscription form with HTML, CSS, and JavaScript. When users click “Subscribe,” the form becomes visible, and they can enter their email. Clicking the send button triggers a thank-you message.
The code works by adding and removing CSS classes to control the form’s visibility and appearance. It’s helpful for capturing email subscriptions on your website.
How to Create Email Subscription Form Using HTML and CSS
1. First of all, load the Font Awesome CSS and Bootstrap CSS by adding the following CDN links into the head tag of your HTML document.
- <link rel='stylesheet' href='https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css'>
- <link rel='stylesheet' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.1.2/css/bootstrap.min.css'>
2. Copy and paste the HTML code into the section of your web page where you want the subscription form to appear. It is typically placed inside a <div>
element with a class of “subscribe-wrapper.” The form structure consists of a “Subscribe” button, an email input field, and a “Send” button.
- <main>
- <div class="subscribe-wrapper">
- <button class="btn subscribe" id="subscribe" type="button">Subscribe</button>
- <button class="btn sending" id="sending" type="button">Thank you!</button>
- <form id="form" action="">
- <label id="wrapper">
- <input class="form-control" type="email" placeholder="Email"/>
- <button class="btn send" id="send" type="submit"><i class="fa fa-envelope"></i></button>
- </label>
- </form>
- </div>
- </main>
3. Add the following CSS code to your project. This CSS code is essential for styling the form and ensuring it looks appealing. The styles define the appearance of buttons, input fields, and their animations.
- @import url("https://fonts.googleapis.com/css?family=Montserrat:300,400,500,600,700");
- html, body {
- width: 100%;
- height: 100%;
- font-family: Montserrat, sans-serif;
- font-size: 14px;
- }
- main{
- width: 100%;
- height: 100%;
- display: flex;
- justify-content: center;
- align-items: center;
- background: #333333 !important;
- overflow: hidden;
- }
- .subscribe-wrapper {
- position: relative;
- min-width: 400px;
- }
- .subscribe-wrapper label {
- display: flex;
- background-color: #494949;
- border-radius: 38px;
- margin-bottom: 0;
- }
- .subscribe-wrapper form {
- opacity: 0;
- visibility: hidden;
- transition: all 0.5s ease;
- }
- .subscribe-wrapper form.active {
- opacity: 1;
- visibility: inherit;
- }
- .form-control {
- height: 51px;
- color: #fff;
- background-color: #494949;
- border-radius: 38px;
- border: none;
- padding: 1rem 2rem;
- }
- .form-control:focus {
- color: #fff;
- background-color: #494949;
- box-shadow: none;
- }
- .btn {
- font-weight: 600;
- text-transform: uppercase;
- letter-spacing: 0.05em;
- color: #fff;
- background-color: #ef4d8a;
- border-radius: 38px;
- padding: 1rem 2rem;
- transform: scale(1);
- transition: all 0.5s ease;
- }
- .btn:hover {
- transform: scale(1.1);
- }
- .btn:focus {
- box-shadow: none;
- }
- .btn.subscribe, .btn.sending {
- position: absolute;
- top: 0;
- z-index: 10;
- }
- .btn.subscribe {
- left: 50%;
- opacity: 1;
- transform: scale(1) translateX(-50%);
- }
- .btn.subscribe.active {
- left: 0;
- opacity: 0;
- transform: scale(1) translateX(-100%);
- }
- .btn.subscribe:hover {
- transform: scale(1.1) translateX(-47%);
- }
- .btn.sending {
- right: 0;
- opacity: 0;
- transform: scale(1) translateX(100%);
- }
- .btn.sending.active {
- right: 50%;
- opacity: 1;
- transform: scale(1) translateX(50%);
- }
- .btn.sending:hover {
- transform: scale(1.1) translateX(47%);
- }
- .btn.send {
- min-width: 51px;
- height: 51px;
- display: flex;
- justify-content: center;
- align-items: center;
- padding: 1rem;
- }
4. Finally, add the following JavaScript code to your project. This code handles the functionality of the subscription form. It manages the form’s visibility and interaction with users. It also includes event listeners for buttons and form submission.
- const subscribeBtn = document.getElementById('subscribe');
- const sendingBtn = document.getElementById('sending');
- const subscribeWrapper = document.getElementById('wrapper');
- const btnSend = document.getElementById('send');
- const form = document.getElementById('form');
- const onClickSubscribeBtn = event => {
- event.stopPropagation();
- subscribeBtn.classList.add('active');
- form.classList.add('active');
- };
- const onClickDocument = event => {
- const clickTarget = event.target.parentNode;
- let botonContainClass = subscribeBtn.classList.contains('active');
- if(botonContainClass && clickTarget != subscribeWrapper) {
- subscribeBtn.classList.remove('active');
- form.classList.remove('active');
- form.reset()
- sendingBtn.classList.remove('active');
- }
- };
- const onClickSend = event => {
- event.preventDefault();
- event.stopPropagation();
- sendingBtn.classList.add('active');
- form.classList.remove('active');
- }
- const init = () => {
- subscribeBtn.addEventListener('click', onClickSubscribeBtn, false);
- btnSend.addEventListener('click', onClickSend, false);
- document.addEventListener('click', onClickDocument, false);
- };
- document.addEventListener('DOMContentLoaded', init);
That’s all! hopefully, you have successfully created an Email Subscription form using HTML, CSS, and JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
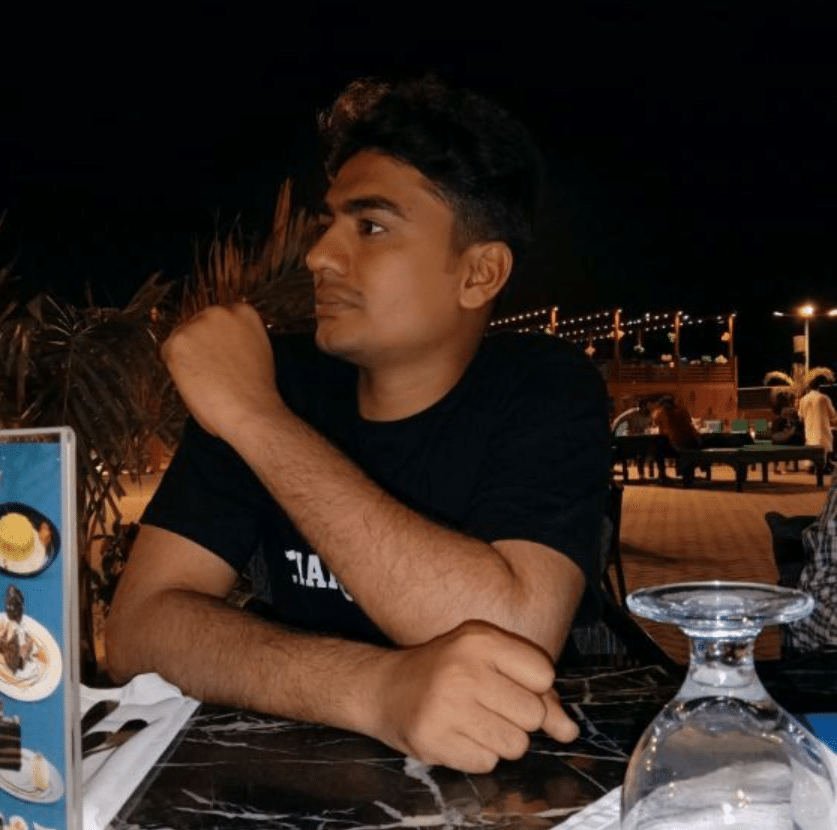
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.