This Vanilla JavaScript code snippet helps you to create a simple fullscreen hamburger menu. The menu interface comes with a background image and a hamburger menu toggle button. The toggle button animates to cross icon and a fullscreen menu displays.
How to Create Simple Fullscreen Hamburger Menu in Vanilla JavaScript
1. First of all, load the Google Fonts CSS into the head tag of your HTML document.
- <link href='https://fonts.googleapis.com/css?family=Montserrat' rel='stylesheet' type='text/css'><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
2. After that, create the HTML structure for the fullscreen hamburger menu as follows:
- <div class="full-menu">
- <nav id="menu" class="menu">
- <ul>
- <li class="home">Home</li>
- <li class="about">About</li>
- <li class="works">Works</li>
- <li class="contact">Contact</li>
- </ul>
- </nav>
- </div>
- <div class="hamburguer">
- <div class="lines line-top"></div>
- <div class="lines line-mid"></div>
- <div class="lines line-bottom"></div>
- </div>
- <main class="content">
- <h1 id="teste">Imagine all your content here</h1>
- </main>
Style using the following CSS styles:
- @-webkit-keyframes zoom {
- 0%, 100% {
- transform: scale(1);
- }
- 50% {
- transform: scale(1.1);
- }
- }
- @keyframes zoom {
- 0%, 100% {
- transform: scale(1);
- }
- 50% {
- transform: scale(1.1);
- }
- }
- html, body {
- line-height: 1.5;
- font-family: "Montserrat", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- }
- html, body, .full-menu, .content {
- width: 100%;
- height: 100%;
- }
- .full-menu, .content {
- display: flex;
- align-items: center;
- justify-content: center;
- }
- .content {
- background-image: url(https://s3.amazonaws.com/StartupStockPhotos/20140808_StartupStockPhotos/96.jpg);
- background-size: cover;
- font-size: 3em;
- min-height: 720px;
- }
- .full-menu {
- position: absolute;
- background-color: #1e88e5;
- opacity: 0.5;
- transform: translateX(100%);
- transition: all 400ms ease-in;
- }
- .full-menu li {
- font-size: 4em;
- color: #fff;
- opacity: 0;
- transform: translateY(2%);
- transition: all 300ms ease-in;
- cursor: pointer;
- }
- .full-menu li:after {
- content: "";
- position: absolute;
- height: 5px;
- width: 0%;
- left: 0;
- bottom: 0;
- background-color: #fff;
- transition: width 200ms ease-in;
- }
- .full-menu li:hover:after {
- width: 100%;
- }
- .full-menu ul {
- list-style: none;
- }
- .full-menu.active {
- transform: translateX(0%);
- opacity: 1;
- z-index: 0;
- }
- .full-menu.active .home {
- transition-delay: 0.3s;
- }
- .full-menu.active .about {
- transition-delay: 0.4s;
- }
- .full-menu.active .works {
- transition-delay: 0.5s;
- }
- .full-menu.active .contact {
- transition-delay: 0.6s;
- }
- .full-menu.active li {
- opacity: 1;
- transform: translateX(0%);
- }
- .full-menu.active li:hover {
- -webkit-animation: zoom 200ms ease-in;
- animation: zoom 200ms ease-in;
- }
- .hamburguer {
- position: absolute;
- width: 2em;
- height: 2em;
- margin: 3em;
- z-index: 1;
- cursor: pointer;
- }
- .hamburguer:hover {
- -webkit-animation: zoom 300ms ease-in;
- animation: zoom 300ms ease-in;
- }
- .lines {
- background-color: #fff;
- width: 100%;
- height: 5px;
- margin: 5px 0;
- transition: all 450ms ease-in;
- }
- .close-hamburguer .lines {
- cursor: pointer;
- }
- .close-hamburguer .line-top {
- transform: translateY(200%) rotate(45deg);
- }
- .close-hamburguer .line-mid {
- opacity: 0;
- }
- .close-hamburguer .line-bottom {
- transform: translateY(-200%) rotate(135deg);
- }
3. Finally, add the following JavaScript function into your project and done.
- //Using Vanilla JS
- document.querySelector(".hamburguer").addEventListener("click", function(){
- document.querySelector(".full-menu").classList.toggle("active");
- document.querySelector(".hamburguer").classList.toggle("close-hamburguer");
- });
That’s all! hopefully, you have successfully created a simple fullscreen hamburger menu. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
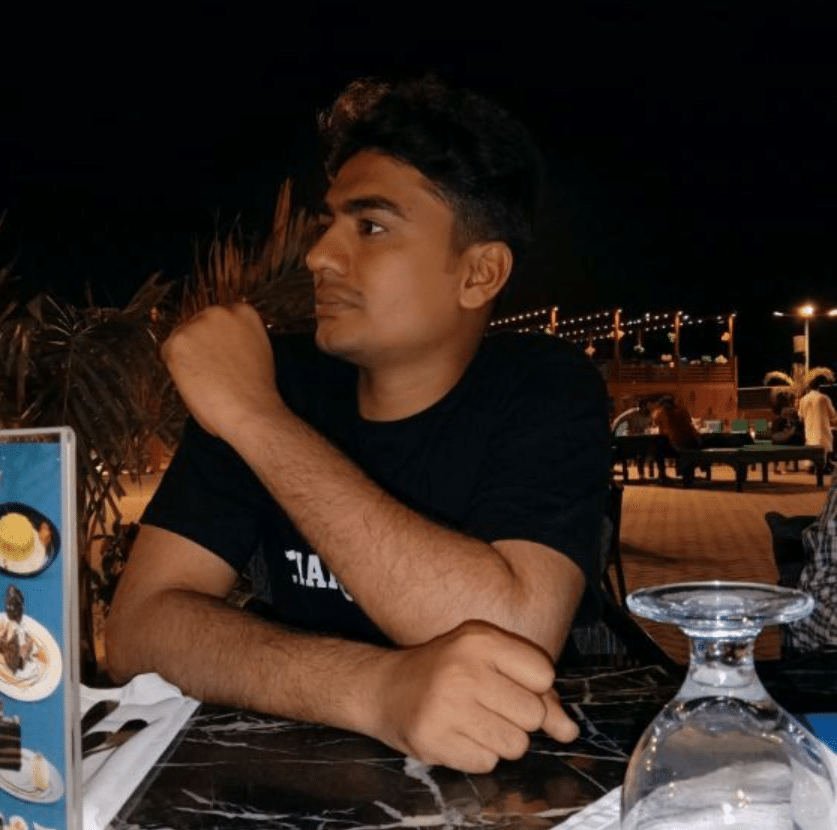
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.