This JavaScript code snippet helps you to create table pagination with a search box. Basically, it arranges data in a table look-like unordered list. It appends a search box and pagination with that list to filter and navigate table data.
How to Create Table Pagination with Search
1. First of all, create the HTML structure as follows:
- <div class="page">
- <div class="page-header cf">
- <h2>Students</h2>
- </div>
- <ul class="student-list">
- <li class="student-item cf">
- <div class="student-details">
- <img class="avatar" src="https://randomuser.me/api/portraits/thumb/women/67.jpg">
- <h3>iboya vat</h3>
- <span class="email">iboya.vat@example.com</span>
- </div>
- <div class="joined-details">
- <span class="date">Joined 07/15/15</span>
- </div>
- </li>
- .
- .
- .
- <li class="student-item cf">
- <div class="student-details">
- <img class="avatar" src="https://randomuser.me/api/portraits/thumb/men/89.jpg">
- <h3>henri kruse</h3>
- <span class="email">henri.kruse@example.com</span>
- </div>
- <div class="joined-details">
- <span class="date">Joined 05/14/13</span>
- </div>
- </li>
- </ul>
- </div>
2. After that, style the basic page layout and table element using the following CSS code.
- .page{
- margin: 50px auto;
- width: 70%;
- background-color: #fff;
- border-radius: 5px;
- padding: 50px;
- }
- .page-header{
- margin-bottom: 20px;
- }
- .page-header h2{
- float: left;
- font-size: 22px;
- text-transform: uppercase;
- font-weight: bold;
- color: #555;
- }
- .page-header .student-search{
- float: right;
- }
- .page-header .student-search input{
- border-radius: 5px;
- border: 1px solid #eaeaea;
- padding: 8px 15px;
- font-size: 14px;
- }
- .page-header .student-search button{
- border-radius: 5px;
- border: 1px solid #eaeaea;
- padding: 8px 15px;
- font-size: 14px;
- background-color: #4ba6c3;
- color: #fff
- }
- .student-list{}
- .student-item{
- margin: 0 0 20px 0;
- padding: 0 0 20px 0;
- border-bottom: 1px solid #eaeaea;
- }
- .student-details{
- width: 50%;
- float: left;
- }
- .student-details .avatar{
- width: 40px;
- height: auto;
- border-radius: 20px;
- float: left;
- margin-right: 14px
- }
- .student-details h3{
- margin: 4px 0 2px 0;
- font-weight: bold;
- color: #4ba6c3;
- }
- .student-details .email{
- color: #888;
- font-size: 14px;
- }
- .joined-details{
- width: 50%;
- float: left;
- text-align: right;
- }
- .joined-details .date{
- margin-top: 15px;
- display: block;
- font-size: 14px;
- color: #999;
- }
- .student-item:last-child{
- margin: 0;
- padding: 0;
- border-bottom: none;
- }
- .pagination{
- margin: 40px 0 0 0;
- text-align: center;
- }
- .pagination li{
- display: inline;
- }
- .pagination li a{
- border: 1px solid #eaeaea;
- border-radius: 5px;
- padding: 3px 8px;
- text-decoration: none;
- color: #4ba6c3;
- }
- .pagination li a.active,
- .pagination li a:hover{
- background-color: #4ba6c3;
- color: #fff;
- }
- /* https://meyerweb.com/eric/tools/css/reset/
- v2.0 | 20110126
- License: none (public domain)
- */
- html, body, div, span, applet, object, iframe,
- h1, h2, h3, h4, h5, h6, p, blockquote, pre,
- a, abbr, acronym, address, big, cite, code,
- del, dfn, em, img, ins, kbd, q, s, samp,
- small, strike, strong, sub, sup, tt, var,
- b, u, i, center,
- dl, dt, dd, ol, ul, li,
- fieldset, form, label, legend,
- table, caption, tbody, tfoot, thead, tr, th, td,
- article, aside, canvas, details, embed,
- figure, figcaption, footer, header, hgroup,
- menu, nav, output, ruby, section, summary,
- time, mark, audio, video {
- margin: 0;
- padding: 0;
- border: 0;
- font-size: 100%;
- font: inherit;
- vertical-align: baseline;
- }
- /* HTML5 display-role reset for older browsers */
- article, aside, details, figcaption, figure,
- footer, header, hgroup, menu, nav, section {
- display: block;
- }
- body {
- line-height: 1;
- }
- ol, ul {
- list-style: none;
- }
- blockquote, q {
- quotes: none;
- }
- blockquote:before, blockquote:after,
- q:before, q:after {
- content: '';
- content: none;
- }
- table {
- border-collapse: collapse;
- border-spacing: 0;
- }
- .cf:after {
- content: ".";
- visibility: hidden;
- display: block;
- height: 0;
- clear: both;
- }
- .navlink {
- float: left;
- }
3. Finally, add the following JavaScript code to your project to functionalize the table search and pagination.
- //get all the main elements on the page
- var page = document.querySelector(".page");
- var pageHeader = document.querySelector(".page-header");
- var studentList = document.querySelector(".student-list");
- var eachStudent = document.querySelectorAll(".student-item");
- var studentDetails = document.querySelector(".student-details");
- var inputString = document.getElementById('inputSearch');
- //Set the pages variable
- var currentPage = 1;
- var numPages = 0;
- var studentsPerPage = 10;
- var index;
- //Recreate Search Element in Js
- var searchBar = function createBar (searchString) {
- //Creating the three elements that make up the search bar
- var studentSearch = document.createElement("div");
- var input = document.createElement("input");
- var searchButton = document.createElement("button");
- var txtNode = document.createTextNode("Search");
- //Define the type of the entry
- input.type="text";
- //Set the attributes fro the different elements to make it easier to select them
- studentSearch.setAttribute("class", "student-search");
- input.setAttribute("id", "inputSearch");
- searchButton.setAttribute("id", "search-button");
- //Append all these elements to the page
- searchButton.appendChild(txtNode);
- studentSearch.appendChild(input);
- studentSearch.appendChild(searchButton);
- //Setting the placeholder for the searchButton
- input.placeholder = "Type name here..";
- //Return the main element which contains all the elements
- return studentSearch;
- }
- //Create static container for pagination in Js
- var paginationFilter = function pageFilter (nbOfEntries) {
- //Create the static elements for the pagination
- var pagination = document.createElement('div');
- var ulList = document.createElement('ul');
- //Giving them attributes to select them easily
- pagination.setAttribute("class", "pagination");
- ulList.setAttribute("id", "pagelist");
- //Append the ulList to the main pagination div
- pagination.appendChild(ulList);
- //Return the main div that contains all the elements
- return pagination;
- };
- //Finding the number of students
- var numberOfStudents = function () {
- var numberOfStudents = eachStudent.length;
- //Return the number of students
- return (numberOfStudents);
- }
- //Finding the number of pages
- var numberOfPages = function () {
- //Divise the number of students by the number of students per pag
- var numberOfPages = parseInt(numberOfStudents() / studentsPerPage);
- //If there is a remaining to the above division then create an extra page
- if ( numberOfStudents() % studentsPerPage > 0 ){
- numPages += 1;
- }
- //Return the number of students
- return numberOfPages;
- };
- //Start by hiding all the students on the list
- var hideAll = function () {
- for (var i = 0; i < numberOfStudents(); i++) {
- eachStudent[i].style.display = "none";
- }
- };
- //Then display only 10 intems per page
- function showStudents (number) {
- for (var i = 0; i < studentsPerPage; i++) {
- index = number * studentsPerPage - studentsPerPage + i;
- eachStudent[index].style.display = "block";
- }
- };
- //Create the pagination links dynamically
- function createPages () {
- for (var i = 0; i <= numberOfPages(); i++) {
- //Create the dynamic elements in the pagination
- var liList = document.createElement('li');
- var pageLink = document.createElement('a');
- //Set attributes to the pagination links & the li list
- pageLink.setAttribute("href", "#");
- pageLink.setAttribute("class", "link");
- liList.setAttribute("class", "pageLi");
- //Append the different elements to the static ulList
- var pagelist = document.getElementById("pagelist");
- pagelist.appendChild(liList);
- liList.appendChild(pageLink);
- //Create and appending the page numbers for the pagination
- var pageNumbers = document.createTextNode(i + 1);
- pageLink.appendChild(pageNumbers);
- pageLink.addEventListener("click", function(){
- var pageNumClicked = (this.innerHTML || this.innerText);
- changePage(pageNumClicked);
- }, false);
- }
- return i;
- };
- //Create the seach feature
- var searchFunction = function searchFeature(searchString) {
- var eachStudentI;
- console.log("Is my search feature working?");
- //Get the value entered in the search box
- var inputString = document.getElementById('inputSearch');
- //Onkeyup we want to filter the content by the string entered in the search box dynamically
- inputString.onkeyup = function() {
- //toUpperCase to make it case insensitive
- var filter = inputString.value.toUpperCase();
- //loop through all the li's
- for (var i = 0; i < eachStudent.length; i++) {
- //Select the student name and retrieve the .innerText value
- var studentName = document.getElementsByTagName("h3");
- var studentInfo = studentName[i].innerText;
- //Display all the results where indexOf() does not return -1
- if (studentInfo.toUpperCase().indexOf(filter) != -1) {
- eachStudent[i].style.display = 'list-item';
- //numberOfStudents = eachStudent[i]
- console.log(eachStudent);
- } else {
- eachStudent[i].style.display = 'none';
- }
- }
- }
- }
- //Global function to add all the elements onLoad of the page
- function addElements() {
- pageHeader.appendChild(searchBar());
- page.appendChild(paginationFilter());
- var searchButton = document.getElementById("search-button");
- searchButton.addEventListener("click", searchFunction);
- //fadeIn();
- //noResults();
- hideAll()
- createPages();
- showStudents(1);
- searchFunction();
- }
- window.onload = addElements();
That’s all! hopefully, you have successfully integrated this JavaScript table search plugin. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
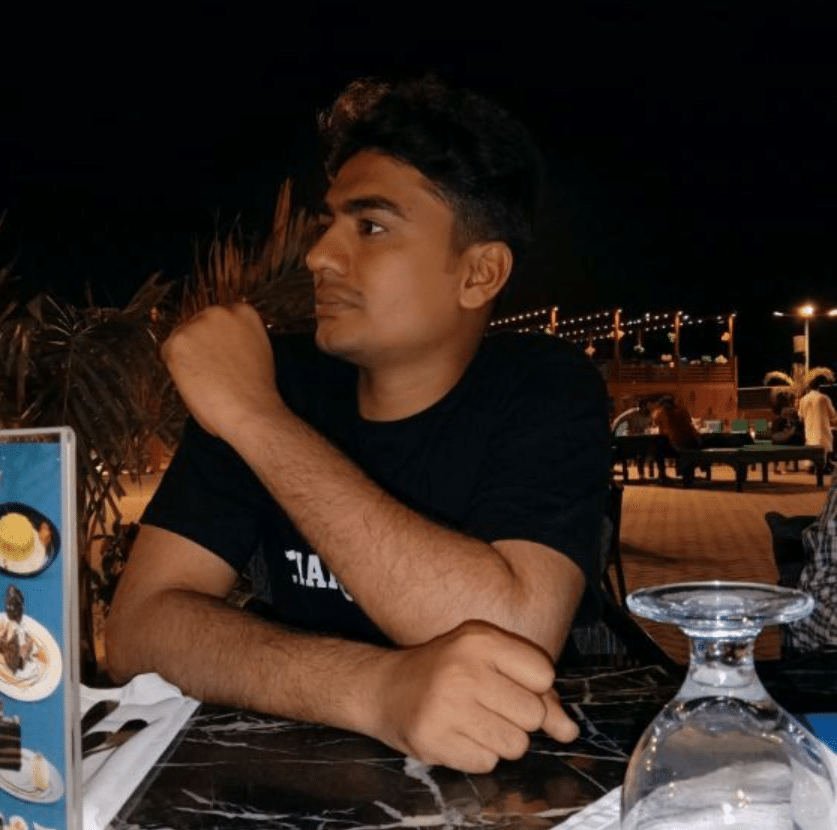
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
This doesn’t work, even in your example. changePage is missing.