This code creates a modal carousel slider using Bootstrap 5, allowing you to showcase a series of items with images.
It works by displaying a set of items in a grid layout. When you click on an item, it opens a modal overlay with navigation buttons (left and right arrows) to view the items as a carousel.
This code is helpful for showcasing multiple items or images in an interactive way, making it easy for users to navigate through them in a modal view.
How to Create Modal Carousel Slider Using Bootstrap 5
1. First of all, load the Bootstrap CSS and Font Awesome CSS by adding the following CDN links into the head tag of your HTML document.
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
- <link rel="stylesheet" href="https://pro.fontawesome.com/releases/v5.10.0/css/all.css" integrity="sha384-AYmEC3Yw5cVb3ZcuHtOA93w35dYTsvhLPVnYs9eStHfGJvOvKxVfELGroGkvsg+p" crossorigin="anonymous" />
- <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.13.0/css/all.min.css" integrity="sha512-L7MWcK7FNPcwNqnLdZq86lTHYLdQqZaz5YcAgE+5cnGmlw8JT03QB2+oxL100UeB6RlzZLUxCGSS4/++mNZdxw==" crossorigin="anonymous" referrerpolicy="no-referrer" />
2. In your HTML file, create the necessary structure for your modal carousel slider. You can place the following code within the <body>
tag of your HTML file.
- <section class="slider my-5">
- <div class="container">
- <div class="row gy-4">
- <div class="col-md-4">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://i1.sndcdn.com/artworks-YxGUGES6DrzzCUGx-Gx32ZQ-t240x240.jpg" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- <div class="col-md-4">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://upload-assets.vice.com/files/2016/03/26/1459014592Basketball.png" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- <div class="col-md-4">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://f4.bcbits.com/img/a2933723780_10.jpg" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- <div class="col-md-4 ">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://inspgr.id/app/uploads/2016/03/3d-grand-chamaco-08.jpg" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- <div class="col-md-4 ">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://images.squarespace-cdn.com/content/v1/53b208ace4b0dc3e7be6ec30/1515709148266-LIUT0GA35774NMFJG036/ke17ZwdGBToddI8pDm48kBtpJ0h6oTA_T7DonTC8zFdZw-zPPgdn4jUwVcJE1ZvWQUxwkmyExglNqGp0IvTJZamWLI2zvYWH8K3-s_4yszcp2ryTI0HqTOaaUohrI8PIWkiAYz5ghgEgSGJuDQ4e1ZKXpRdhEMT7SgthRpD0vyIKMshLAGzx4R3EDFOm1kBS/H20Bounce.jpg" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- <div class="col-md-4 ">
- <div class="item position-relative">
- <div class="img-item">
- <img src="https://www.webdesignertrends.com/wp-content/uploads/2015/01/el-grand-chamacon-24.jpg" alt="item photo" class="w-100">
- </div>
- <div class="content-item text-center p-2 bg-white position-absolute">
- <h4>item title</h4>
- <p>Lorem ipsum, dolor sit amet consectetur adipisicing.</p>
- </div>
- </div>
- </div>
- </div>
- </div>
- <div class="modal-layer position-fixed justify-content-center align-items-center">
- <div class="slide-img w-75 h-75 d-flex align-items-center justify-content-between px-4 position-relative">
- <i id="closeBtn" class="far fa-times-circle fa-2x position-absolute end-0 top-0 m-4"></i>
- <i id="prev" class="fas fa-chevron-left fa-2x"></i>
- <i id="next" class="fas fa-chevron-right fa-2x"></i>
- </div>
- </div>
- </section>
3. You can apply custom CSS styles to the slider and items as needed to match your website’s design. Modify the CSS code to achieve your desired look and feel.
- section .content-item {
- width: 80%;
- left: 10%;
- bottom: 10%;
- }
- section .modal-layer {
- inset: 0;
- background: rgba(0, 0, 0, 0.6);
- display: none;
- }
- section .modal-layer .slide-img {
- background-image: url(https://i1.sndcdn.com/artworks-YxGUGES6DrzzCUGx-Gx32ZQ-t240x240.jpg);
- background-size: cover;
- background-position: 50% 50%;
- background-repeat: no-repeat;
- }
4. Load the Bootstrap JS by adding the following script just before closing the body tag:
- <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
5. Finally, add the following JavaScript code to enable the modal carousel slider’s functionality. This code handles item click events, navigation, and keyboard controls.
- // variables
- // items & images
- let itemsImgs = document.querySelectorAll(".img-item img");
- itemsImgsArr = Array.from(itemsImgs);
- // pop slider
- let popSlider = document.querySelector("section .modal-layer");
- let bgSlide = document.querySelector(".modal-layer .slide-img");
- // icons/buttons
- // close
- let closeBtn = document.querySelector("#closeBtn");
- closeBtn.style.cssText = `cursor : pointer;`;
- // left
- let prevBtn = document.querySelector("#prev");
- prevBtn.style.cssText = `cursor : pointer;`;
- // right
- let nextBtn = document.querySelector("#next");
- nextBtn.style.cssText = `cursor : pointer;`;
- // index of item
- let activeIndex;
- for (let i = 0; i < itemsImgs.length; i++) {
- itemsImgs[i].addEventListener("click", function (e) {
- popSlider.style.display = "flex";
- let activeSrc = e.target.src;
- bgSlide.style.backgroundImage = `url(${activeSrc})`;
- activeIndex = itemsImgsArr.indexOf(e.target);
- });
- }
- closeBtn.addEventListener("click", removeSlider);
- function removeSlider() {
- popSlider.style.display = "none";
- }
- nextBtn.addEventListener("click", nextSlider);
- function nextSlider() {
- activeIndex++;
- if (activeIndex == itemsImgs.length) {
- activeIndex = 0;
- }
- let imgSrc = itemsImgsArr[activeIndex].src;
- bgSlide.style.backgroundImage = `url(${imgSrc})`;
- }
- prevBtn.addEventListener("click", prevSlider);
- function prevSlider() {
- activeIndex--;
- if (activeIndex < 0) {
- activeIndex = itemsImgs.length - 1;
- }
- let imgSrc = itemsImgsArr[activeIndex].src;
- bgSlide.style.backgroundImage = `url(${imgSrc})`;
- }
- // keyboard events
- document.addEventListener("keydown", function (e) {
- if (e.key == "Escape") {
- removeSlider();
- } else if (e.key == "ArrowRight") {
- nextSlider();
- } else if (e.key == "ArrowLeft") {
- prevSlider();
- }
- });
That’s all! hopefully, you have successfully created a Modal Carousel Slider using Bootstrap 5. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
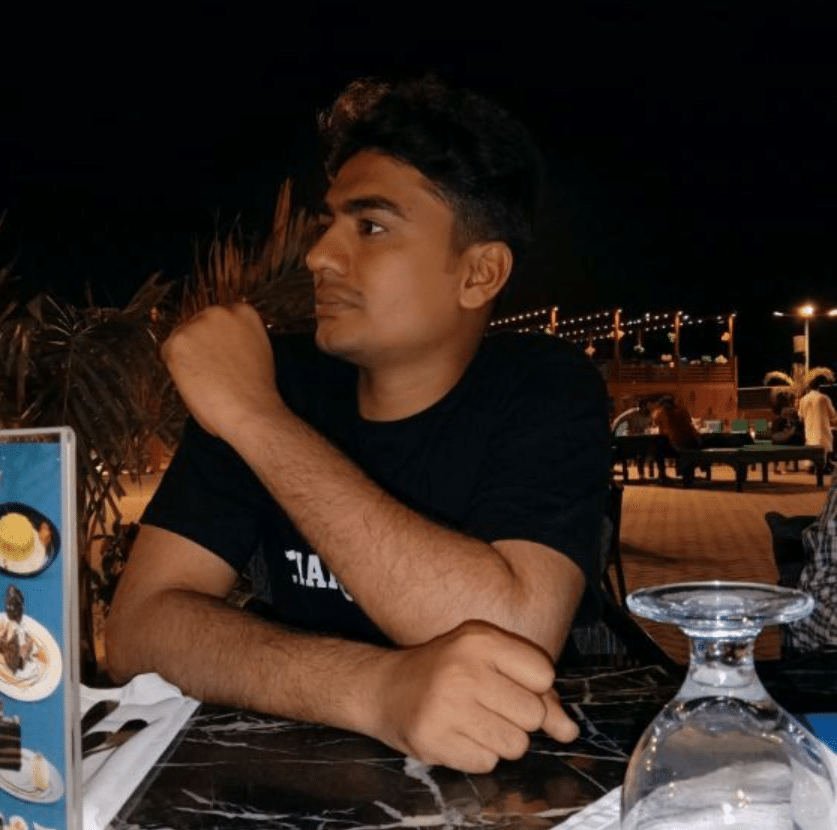
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.