Yet another code snippet to create a simple to do list using Bootstrap 5. It allows users to add and remove and modify tasks lists. You can easily integrate this lightweight code to create to-do lists according to your needs.
How to Create To-Do List Using Bootstrap 5
1. In the very first step, load the Bootstrap 5 CSS into the head tag of your webpage.
- <!-- Bootstrap 5 CSS -->
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-KyZXEAg3QhqLMpG8r+8fhAXLRk2vvoC2f3B09zVXn8CA5QIVfZOJ3BCsw2P0p/We" crossorigin="anonymous">
2. After that, create the HTML structure for the to-do list as follows:
- <div class=" container rounded-3 border border-2 border-dark my-5 bg-white" style="height:auto;">
- <div>
- <h1 class=" h1">To Do List</h1>
- <div class="row">
- <div class=" col-8">
- <input class=" py-3 form-control shadow" placeholder="input your task" type="text" id="inputText">
- </div>
- <div class="col-2">
- <!-- <i onclick="addList()" class=" btn btn-dark rounded-pill fas fa-4x fa-plus-circle "></i> -->
- <button onclick="addList()" class=" mt-2 btn btn-dark"> Add </button>
- </div>
- </div>
- </div>
- <hr>
- <div class="row rounded bg-white">
- <div class=" col-12">
- <ul class=" list-group" id="list"></ul>
- </div>
- </div>
- </div>
3. Finally, add the following to-do list JavaScript program into your project and done.
- let input = document.getElementById("inputText");
- let list= document.getElementById("list");
- let minimalValue = 3;
- let listNum = 0;
- addList=()=>{
- // get
- let inputText = filterList(input.value);
- // set
- if (inputText) {
- list.innerHTML += ` <li class=" my-3 py-3 shadow list-group-item " id="list${listNum}">
- <div class="row">
- <div class="col-1">
- <input class="" type="checkbox" id="check${listNum}" onclick="done(${listNum})">
- </div>
- <div class="col-6">
- <span class=" h4" id="text${listNum}"> ${inputText} </span>
- </div>
- <div class="col-4">
- <button class=" btn btn-dark" onclick="deleteList(${listNum})">Delete</button>
- <button class=" btn btn-dark" onclick="editList(${listNum})">Edit</button>
- </div>
- </div>
- </li> `;
- input.value=" ";
- listNum++;
- }
- }
- done=(listId)=>{
- let checkbox = document.getElementById(`check${listId}`);
- let current = document.getElementById(`text${listId}`);
- let classExit=current.classList.contains("text-decoration-line-through");
- if (classExit == true) {
- current.classList.remove("text-decoration-line-through");
- }else{
- current.classList.add("text-decoration-line-through");
- }
- }
- filterList=(x)=>{
- if (x) {
- if (x.length >= minimalValue) {
- return x;
- }
- else{
- alert("Please enter more than 3 words")
- }
- }
- else{
- return false;
- }
- }
- editList=(listId)=>{
- let currentText = document.getElementById(`text${listId}`);
- let newText = prompt("Wanna Change list?",currentText.innerHTML);
- if (filterList(newText)) {
- currentText.innerHTML = newText;
- }
- }
- deleteList=(listId)=>{
- let current = document.getElementById(`text${listId}`).innerHTML;
- let deleteComfirm = confirm(`Are you sure to delete ${current}`);
- if (deleteComfirm) {
- let p = document.getElementById("list")
- let c = document.getElementById(`list${listId}`);
- p.removeChild(c);
- }
- else{
- console.log("deleted");
- }
- };
Hopefully, you have successfully created a to-do lists for Bootstrap 5 projects. If you have any questions or suggestions, let me know by comment below.
Similar Code Snippets:
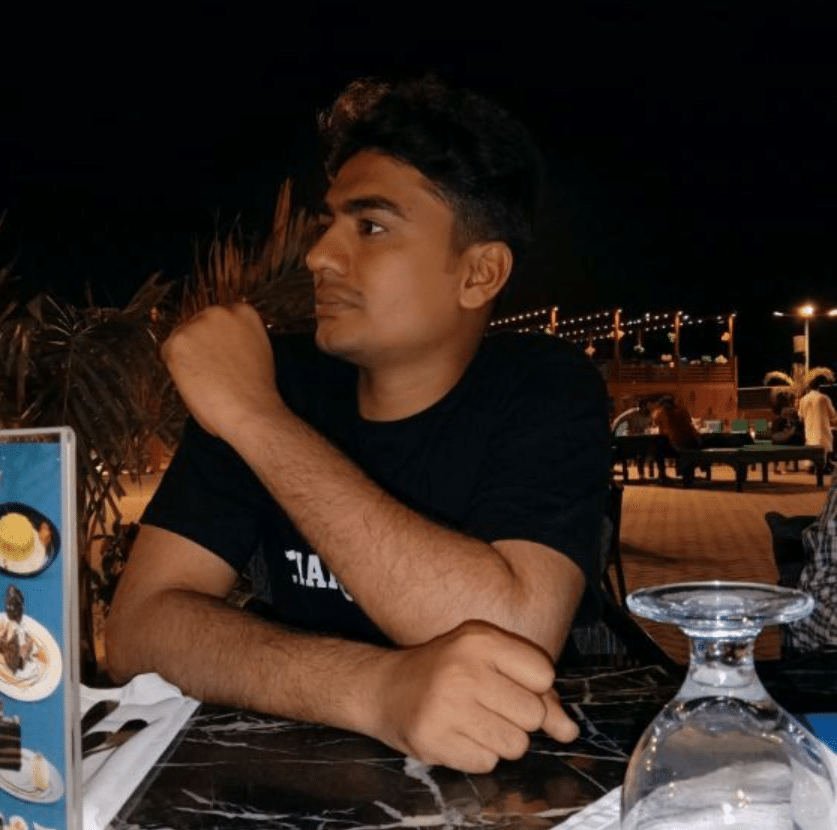
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.