This code snippet provides a simple and effective way to animate progress bars using Bootstrap on page load. It selects each progress bar element on the page and applies an animation to gradually increase the width of the progress bar based on its aria-valuenow
attribute. This creates a smooth visual transition to represent the progress being made.
Moreover, the code includes a text animation feature. It updates the text inside each progress bar with a percentage value, simulating a counting effect. This is achieved by using the animate()
function to increment the value over time until reaching the target percentage.
How to Create Bootstrap Progress Bar Animation on Page Load
1. First of all, load the Bootstrap 5 CSS by adding the following CDN link into the head tag of your HTML document.
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
2. Create the HTML structure for the progress bar as follows:
- <div class="row">
- <div class="col-md-6">
- <h4>Dutch</h4>
- <div class="progress">
- <div class="progress-bar" role="progressbar" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100">100</div>
- </div>
- <h4>French</h4>
- <div class="progress">
- <div class="progress-bar" role="progressbar" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100">100</div>
- </div>
- <h4>English</h4>
- <div class="progress">
- <div class="progress-bar" role="progressbar" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100">100</div>
- </div>
- <h4>German</h4>
- <div class="progress">
- <div class="progress-bar" role="progressbar" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100">50</div>
- </div>
- <h4>Spanish</h4>
- <div class="progress">
- <div class="progress-bar" role="progressbar" aria-valuenow="20" aria-valuemin="0" aria-valuemax="100">20</div>
- </div>
- </div>
- </div>
You can customize the progress bars by modifying the HTML code. Each progress bar is represented by the <div class="progress-bar">
element. Adjust the values of aria-valuenow
, aria-valuemin
, and aria-valuemax
to reflect the desired completion level.
3. Add the following CSS styles to adjust the basic layout for the progress bars. You can also define additional CSS to customize the progress bars according to your needs.
- .row {
- padding: 4em 3em;
- }
- .textbox {
- height: 700px;
- }
- .progress {
- margin-bottom: 10px;
- }
- .progress-bar {
- width: 0;
- }
4. Now, load the jQuery and Bootstrap JS by adding the following CDN links before closing the body tag:
- <script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js'></script>
- <script src='https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/js/bootstrap.min.js'></script>
5. Finally, initialize the progress bar by adding the following JavaScript code to your project.
- var delay = 500;
- $(".progress-bar").each(function(i){
- $(this).delay( delay*i ).animate( { width: $(this).attr('aria-valuenow') + '%' }, delay );
- $(this).prop('Counter',0).animate({
- Counter: $(this).text()
- }, {
- duration: delay,
- easing: 'swing',
- step: function (now) {
- $(this).text(Math.ceil(now)+'%');
- }
- });
- });
That’s all! hopefully, you have successfully created Bootstrap progress bar Animation on page load. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
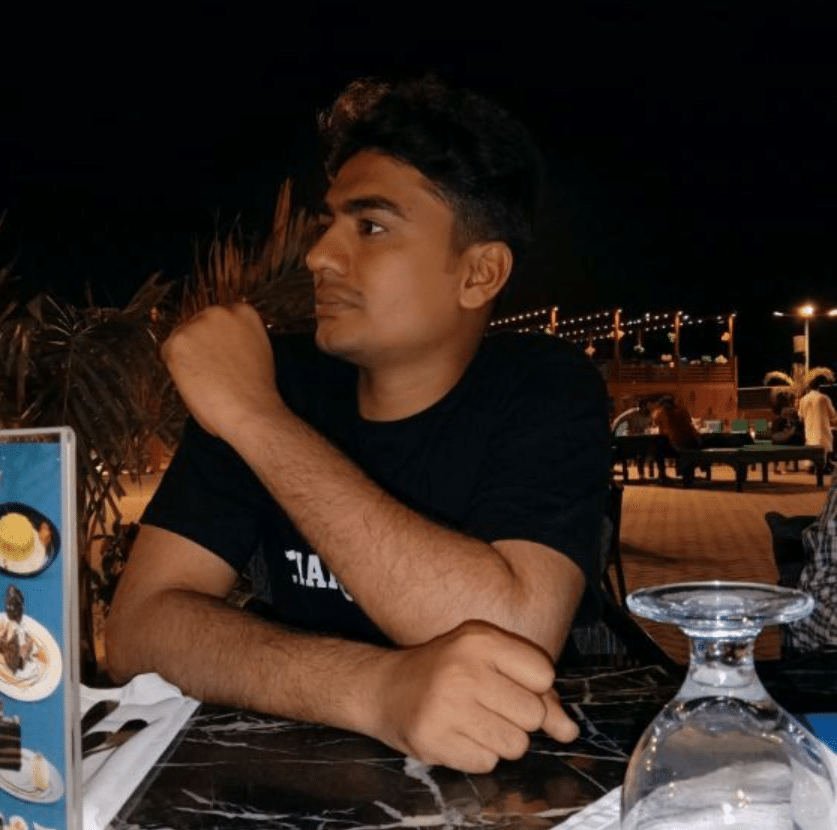
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.