Confetti.js is a lightweight JavaScript plugin to create a confetti celebration explosion effect. It draws confetti graphics on the HTML canvas element. The plugin allows you to render confetti effect on any JavaScript event, like click, hover, or resize. So, you can use this animation effect for various purposes on the webpage.
The confetti effect is useful to implement on the welcome, congratulations, or celebration webpage. Anyhow, you can also integrate it for various purposes like the background of the hero section.
Basically, there are no configurable options to customize the working of the plugin. But you can modify the function file to get the desired output. Like you can redefine colors, confetti size, spinning values, and the round of confetti, etc. Moreover, you have full control over the canvas element, you can style it according to your needs.
How to Create Confetti Explosion Effect
1. In the very first step, create HTML canvas element with the class name "confetti"
and define its unique id “canvas”.
- <canvas class="confetti" id="canvas"></canvas>
2. After that, style the confetti container using CSS. Select the "confetti"
class and define its max-width, display, margin, and border property as follows. Use the CSS user-select property to forbade text selection while clicking for confetti explosion.
- .confetti{
- max-width: 640px;
- display: block;
- margin: 0 auto;
- border: 1px solid #ddd;
- user-select: none;
- }
3. Add the “confetti.js” function between the <script> and </script> tag before the closing of body tag and done.
- //-----------Var Inits--------------
- canvas = document.getElementById("canvas");
- ctx = canvas.getContext("2d");
- canvas.width = window.innerWidth;
- canvas.height = window.innerHeight;
- cx = ctx.canvas.width / 2;
- cy = ctx.canvas.height / 2;
- let confetti = [];
- const confettiCount = 300;
- const gravity = 0.5;
- const terminalVelocity = 5;
- const drag = 0.075;
- const colors = [
- { front: 'red', back: 'darkred' },
- { front: 'green', back: 'darkgreen' },
- { front: 'blue', back: 'darkblue' },
- { front: 'yellow', back: 'darkyellow' },
- { front: 'orange', back: 'darkorange' },
- { front: 'pink', back: 'darkpink' },
- { front: 'purple', back: 'darkpurple' },
- { front: 'turquoise', back: 'darkturquoise' }];
- //-----------Functions--------------
- resizeCanvas = () => {
- canvas.width = window.innerWidth;
- canvas.height = window.innerHeight;
- cx = ctx.canvas.width / 2;
- cy = ctx.canvas.height / 2;
- };
- randomRange = (min, max) => Math.random() * (max - min) + min;
- initConfetti = () => {
- for (let i = 0; i < confettiCount; i++) {
- confetti.push({
- color: colors[Math.floor(randomRange(0, colors.length))],
- dimensions: {
- x: randomRange(10, 20),
- y: randomRange(10, 30) },
- position: {
- x: randomRange(0, canvas.width),
- y: canvas.height - 1 },
- rotation: randomRange(0, 2 * Math.PI),
- scale: {
- x: 1,
- y: 1 },
- velocity: {
- x: randomRange(-25, 25),
- y: randomRange(0, -50) } });
- }
- };
- //---------Render-----------
- render = () => {
- ctx.clearRect(0, 0, canvas.width, canvas.height);
- confetti.forEach((confetto, index) => {
- let width = confetto.dimensions.x * confetto.scale.x;
- let height = confetto.dimensions.y * confetto.scale.y;
- // Move canvas to position and rotate
- ctx.translate(confetto.position.x, confetto.position.y);
- ctx.rotate(confetto.rotation);
- // Apply forces to velocity
- confetto.velocity.x -= confetto.velocity.x * drag;
- confetto.velocity.y = Math.min(confetto.velocity.y + gravity, terminalVelocity);
- confetto.velocity.x += Math.random() > 0.5 ? Math.random() : -Math.random();
- // Set position
- confetto.position.x += confetto.velocity.x;
- confetto.position.y += confetto.velocity.y;
- // Delete confetti when out of frame
- if (confetto.position.y >= canvas.height) confetti.splice(index, 1);
- // Loop confetto x position
- if (confetto.position.x > canvas.width) confetto.position.x = 0;
- if (confetto.position.x < 0) confetto.position.x = canvas.width;
- // Spin confetto by scaling y
- confetto.scale.y = Math.cos(confetto.position.y * 0.1);
- ctx.fillStyle = confetto.scale.y > 0 ? confetto.color.front : confetto.color.back;
- // Draw confetti
- ctx.fillRect(-width / 2, -height / 2, width, height);
- // Reset transform matrix
- ctx.setTransform(1, 0, 0, 1, 0, 0);
- });
- // Fire off another round of confetti
- if (confetti.length <= 10) initConfetti();
- window.requestAnimationFrame(render);
- };
- //---------Execution--------
- initConfetti();
- render();
- //----------Resize----------
- window.addEventListener('resize', function () {
- resizeCanvas();
- });
- //------------Click------------
- window.addEventListener('click', function () {
- initConfetti();
- });
That’s all! hopefully, you have successfully created the confetti explosion effect using this JavaScript plugin. If you have any questions or suggestions, let me know by comment below.
Similar Code Snippets:
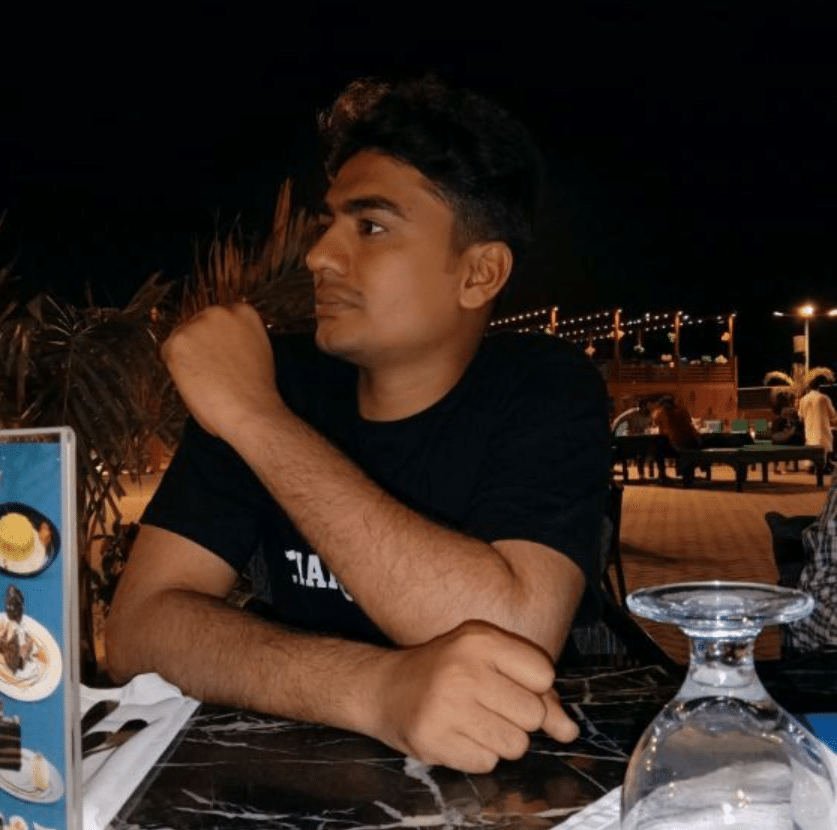
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
How to make confetti appear once and then disappear
Ported this code to React + Typescript:
https://gist.github.com/foucdeg/a6d7b9b0663ede204b0bdff5efc1eae3
Hey,
How do I add this to the whole background of my page instead of a certain place within the html?
I want the Confetti to appear behind all the elements of the page but full screen.
Thank you!