This Vanilla JS code snippet helps you to create a smooth scroll to sections. It comes with a smooth scrolling list in which list items can be clicked and it will smoothly scroll up. You can integrate this code snippet to make a smooth scrolling list.
How to Create Smooth Scroll in Vanilla JS
1. First of all, create the HTML structure as follows:
- <ul>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- <li>CLICK TO SCROLL HERE</li>
- </ul>
2. After that, add the following CSS styles to your project:
- * {
- margin: 0;
- padding: 0;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale;
- -webkit-overflow-scrolling: touch;
- -webkit-tap-highlight-color: rgba(0, 0, 0, 0);
- -moz-tap-highlight-color: rgba(0, 0, 0, 0);
- tap-highlight-color: rgba(0, 0, 0, 0);
- }
- ul li:nth-child(1) {
- color: rgba(60, 60, 80, 0.8);
- background: rgba(0, 0, 255, 0.0333333333);
- }
- ul li:nth-child(2) {
- color: rgba(120, 120, 160, 0.8);
- background: rgba(0, 0, 255, 0.0666666667);
- }
- ul li:nth-child(3) {
- color: rgba(180, 180, 240, 0.8);
- background: rgba(0, 0, 255, 0.1);
- }
- ul li:nth-child(4) {
- color: rgba(240, 240, 255, 0.8);
- background: rgba(0, 0, 255, 0.1333333333);
- }
- ul li:nth-child(5) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.1666666667);
- }
- ul li:nth-child(6) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.2);
- }
- ul li:nth-child(7) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.2333333333);
- }
- ul li:nth-child(8) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.2666666667);
- }
- ul li:nth-child(9) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.3);
- }
- ul li:nth-child(10) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.3333333333);
- }
- ul li:nth-child(11) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.3666666667);
- }
- ul li:nth-child(12) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.4);
- }
- ul li:nth-child(13) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.4333333333);
- }
- ul li:nth-child(14) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.4666666667);
- }
- ul li:nth-child(15) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.5);
- }
- ul li:nth-child(16) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.5333333333);
- }
- ul li:nth-child(17) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.5666666667);
- }
- ul li:nth-child(18) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.6);
- }
- ul li:nth-child(19) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.6333333333);
- }
- ul li:nth-child(20) {
- color: rgba(255, 255, 255, 0.8);
- background: rgba(0, 0, 255, 0.6666666667);
- }
- ul {
- position: relative;
- top: 0;
- right: 0;
- bottom: 0;
- left: 0;
- width: 90%;
- max-width: 300px;
- height: 640px;
- overflow: auto;
- margin: auto;
- border-radius: 4px;
- box-shadow: 0 3px 6px rgba(0, 0, 0, 0.16), 0 3px 6px rgba(0, 0, 0, 0.23);
- }
- ul li {
- width: 100%;
- height: 80px;
- font: 300 12px/80px Arial, sans-serif;
- color: rgba(255, 255, 255, 0.5);
- text-align: center;
- list-style: none;
- cursor: pointer;
- }
- ul li.is-selected {
- background: #fbfbfb;
- color: rgba(0, 0, 255, 0.5);
- }
3. Finally, add the following JavaScript code and done.
- document.
- querySelector('ul').
- addEventListener('click', function (e) {
- smoothScrollTo(selected(e.target, this).offsetTop, this);
- });
- function smoothScrollTo(destination, parent, time) {
- let scroll = init();
- requestAnimationFrame(shouldScroll);
- function init() {
- let start = parent.scrollTop;
- let ticks = time || 30;
- let i = 0;
- let positionY = () => (destination - start) * i / ticks + start;
- let isFinished = () => i++ >= ticks;
- return { positionY, isFinished };
- }
- function shouldScroll() {
- if (scroll.isFinished()) return;
- parent.scrollTop = scroll.positionY();
- requestAnimationFrame(shouldScroll);
- }
- }
- function selected(elem, parent) {
- for (let i = 0; i < parent.children.length; i++) {
- parent.children[i].classList.remove('is-selected');
- }
- elem.classList.add('is-selected');
- return elem;
- }
That’s all! hopefully, you have successfully integrated this smooth scroll code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
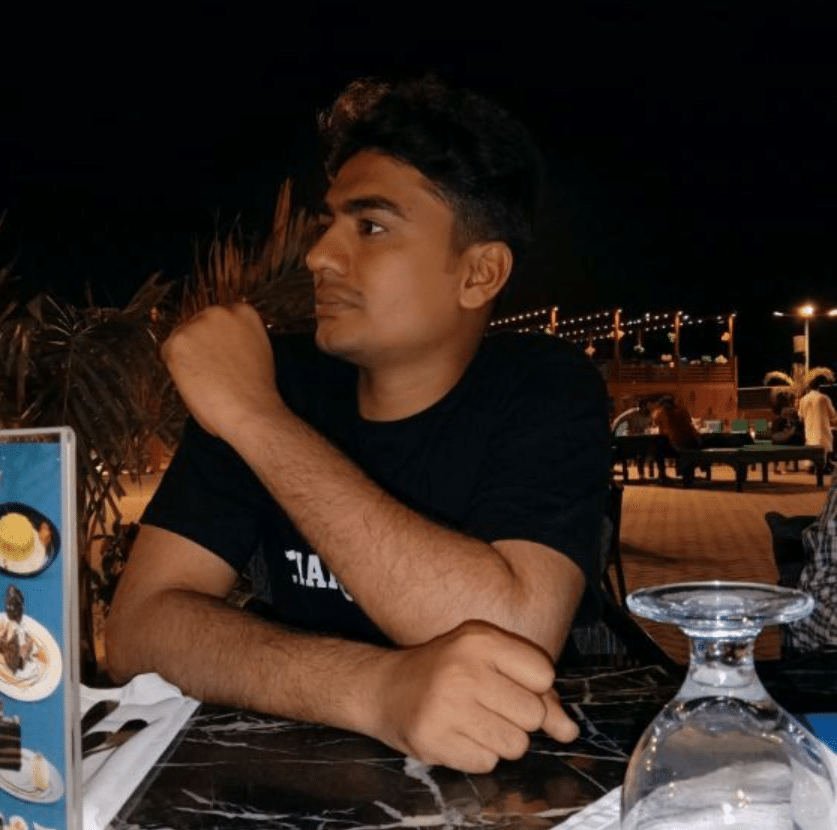
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.