This JavaScript code snippet helps you to show a tooltip on the mouse position on a webpage. It uses clientX and clientY properties of the mouse event to get the x and y coordinates of the cursor then sets the top and left CSS properties of the tooltip element.
The tooltip comes with a simple box that appears when the user hovers over an element. You can integrate this snippet to display additional information about the element, such as a description or a link. Moreover, you can customize the tooltip element with additional CSS according to your needs.
How to Show Tooltip On Mouse Position in JavaScript
1. First, create a container for your image and tooltip. Use the following HTML structure as a starting point:
- <div class="container">
- <div class="img-block shadow-effect">
- <img class="image" src="https://www.telegraph.co.uk/content/dam/food-and-drink/2017/01/04/coffeeS006G8_wwwAlamycom_Heart-shaped-coffee-art-on-a-latte_trans_NvBQzQNjv4BqEDjTm7JpzhSGR1_8ApEWQA1vLvhkMtVb21dMmpQBfEs.jpg">
- <span id="tooltip">❤ Coffee</span>
- </div>
- </div>
2. Apply the necessary CSS styles to ensure the proper layout and appearance. You can customize the styles according to your website’s design:
- @import url(https://fonts.googleapis.com/css?family=Open+Sans);
- .container {
- display: flex;
- flex-direction: column;
- justify-content: center;
- align-items: center;
- height: 95vh;
- width: 100%;
- }
- .img-block {
- max-height: 80%;
- width: auto;
- cursor: pointer;
- display: flex;
- justify-content: center;
- position: relative;
- }
- .image {
- max-height: 100%;
- width: auto;
- max-width: 100%
- }
- #tooltip {
- position: fixed;
- height: fit-content;
- width: fit-content;
- background-color: white;
- padding: 5px 12px;
- box-shadow: 0 0 2px rgba(0,0,0,0.2);
- border-radius: 5px;
- font-family: 'Open Sans';
- display: none;
- }
- .img-block:hover #tooltip {
- display: block;
- }
- .shadow-effect {
- position:relative;
- -webkit-box-shadow:0 1px 4px rgba(0, 0, 0, 0.3), 0 0 40px rgba(0, 0, 0, 0.1) inset;
- -moz-box-shadow:0 1px 4px rgba(0, 0, 0, 0.3), 0 0 40px rgba(0, 0, 0, 0.1) inset;
- box-shadow:0 1px 4px rgba(0, 0, 0, 0.3), 0 0 40px rgba(0, 0, 0, 0.1) inset;
- }
- .shadow-effect:before, .shadow-effect:after {
- content:"";
- position:absolute;
- z-index:-1;
- -webkit-box-shadow:0 0 20px rgba(0,0,0,0.8);
- -moz-box-shadow:0 0 20px rgba(0,0,0,0.8);
- box-shadow:0 0 20px rgba(0,0,0,0.8);
- top:5px;
- bottom:0;
- left:10px;
- right:10px;
- -moz-border-radius:100px / 10px;
- border-radius:100px / 10px;
- }
- .shadow-effect:after {
- right:10px;
- left:auto;
- -webkit-transform:skew(8deg) rotate(3deg);
- -moz-transform:skew(8deg) rotate(3deg);
- -ms-transform:skew(8deg) rotate(3deg);
- -o-transform:skew(8deg) rotate(3deg);
- transform:skew(8deg) rotate(3deg);
- }
3. Finally, integrate the following JavaScript code to enable the dynamic positioning of the tooltip based on the mouse cursor:
- let toolTip = document.getElementById('tooltip');
- window.addEventListener('mousemove', toolTipXY);
- function toolTipXY(e) {
- let x = e.clientX,
- y = e.clientY;
- toolTip.style.top = (y + 20) + 'px';
- toolTip.style.left = (x + 20) + 'px';
- };
That’s all! hopefully, you have successfully created a functionality to display the tooltip on the cursor position using JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
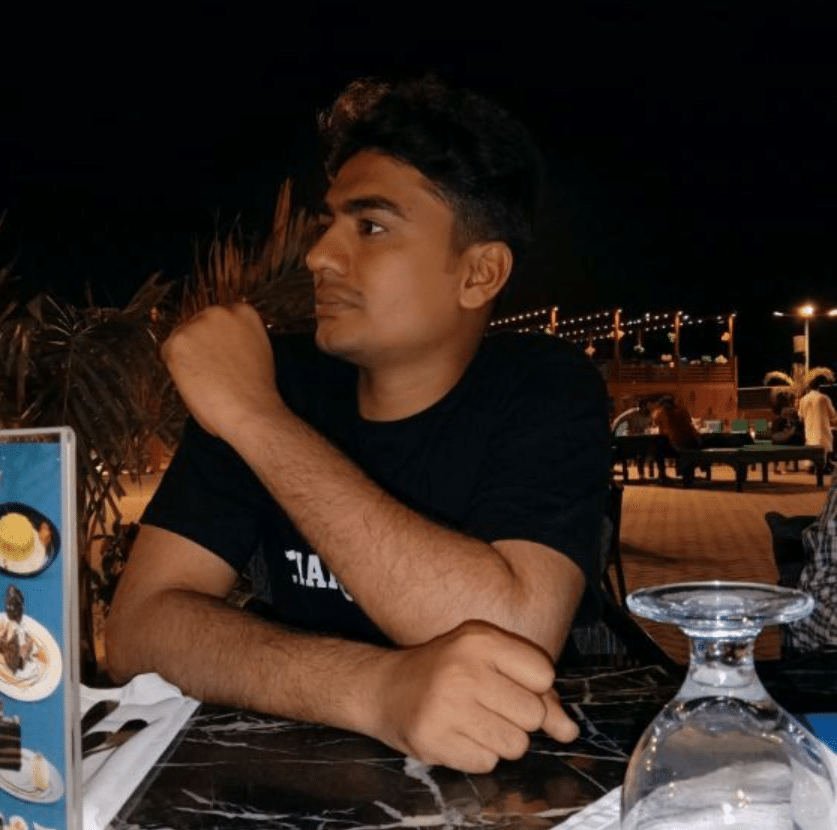
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.