This JavaScript code snippet helps you to create a simple tool to generate random background color with preview. It uses Math.floor() function to get a random value for HTML hex color code and set its value to preview div as background. Similarly, the generated color code display in a text input where users can easily copy the color code to clipboard.
How to Create JavaScript Generate Random Background Color
1. First of all, create the HTML structure as follows:
- <div class="wrapper">
- <button id="gen">Generate</button>
- <button id="clear">Clear</button>
- <input id="randomNum">
- <button id="copy">Copy</button>
- <div id="copyCheck">
- <i class="glyphicon glyphicon-ok"></i>
- <span>Color Code Copied</span>
- </div>
- </div>
2. After that, add the following CSS styles to your project:
- * {box-sizing: border-box;}
- body {
- position: relative;
- background: #F1A9A0;
- }
- .wrapper {
- margin: 200px auto 0;
- width: 420px;
- }
- button {
- width: 200px;
- padding: 10px 20px;
- background: #E74C3C;
- border: none;
- outline: none;
- color: #fff;
- font-size: 16px;
- font-weight: 100;
- transition: all 0.2s ease;
- }
- button:hover {background: #da493a;}
- button:active {background: #f7654b;}
- button:active, button:focus {outline: none;}
- button#copy {width: 80px; height: 50px; position: relative;}
- input#randomNum {
- width: 320px;
- height: 50px;
- outline: none;
- line-height: 50px;
- font-size: 14px;
- border: none;
- text-align: center;
- margin-top: 20px;
- color: #fff;
- box-shadow: inset 0px 0px 200px 10px #446CB3;
- }
- #copyCheck {
- position: fixed;
- top: 10%;
- right: -200px;
- display: inline-block;
- background: #353535;
- padding: 10px 20px;
- border-radius: 5px;
- color: #fff;
- box-shadow: 0px 3px 2px 0px rgba(0,0,0,0.32);
- transition: all 0.3s ease;
- }
- #copyCheck i {
- border-radius: 50%;
- background: #2d8448;
- padding: 10px;
- }
3. Finally, add the following JavaScript code and done.
- // Some Variables
- var genButton = document.getElementById("gen"), // Generate button
- clear = document.getElementById("clear"), // Clear button
- output = document.getElementById("randomNum"), // Output space
- copy = document.getElementById("copy"), // Copy to Clipboard Button
- copyCheck = document.getElementById("copyCheck"); // copy check
- // Get Random Number Function && make it the background of the body xD
- function getRandomNum() {
- "use strict";
- output.value = "#" + Math.floor((1e+5) + Math.random() * (9e+5)); // get random 6-digits number
- var bgClr = output.value;
- document.getElementById("preview").style.backgroundColor = bgClr; // set body bgColor to that random number
- }
- // Clear Random Number Function + make background of the body as default
- function clearNum() {
- "use strict";
- output.value = ""; // clear output space
- document.getElementById("preview").style.backgroundColor = "#F1A9A0"; // make bgColor of the body as default
- }
- // Copy the color code to the clipboard by clicking on "Copy" Button
- function copyToClipBoard() {
- "use strict";
- document.execCommand("copy", false, output.select());
- copyCheck.style.right = "20px";
- showCheck = setTimeout(function() {
- copyCheck.style.right = "-200px";
- }, 3000);
- clearTimeout(showCheck);
- }
- // Adding EventListener to the buttons
- genButton.addEventListener("click", getRandomNum); // Generate Button
- clear.addEventListener("click", clearNum); // Clear Button
- copy.addEventListener("click", copyToClipBoard);
- //---------------- Thanks for being here --------------------- //
That’s all! hopefully, you have successfully integrated this random background color code snippet into your project. If you have any questions or facing any issues, feel free to comment below.
Similar Code Snippets:
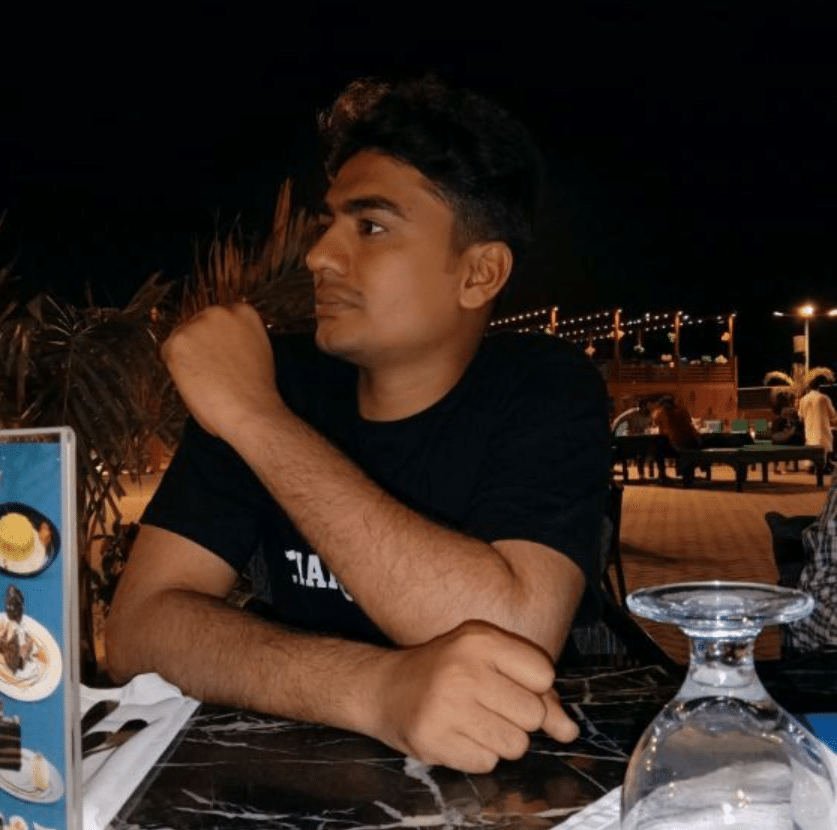
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.