This code demonstrates how to increment and decrement a counter in JavaScript. It works by using two functions, “inc()” and “dec()”, triggered by button clicks. The major functionality is to increase or decrease the displayed number, providing a simple interactive counter for your web page.
It’s helpful for tracking items, votes, or any numeric values, enhancing user engagement. The code provides a user-friendly way to increase or decrease counts with just a click.
How to Create Increment and Decrement Counters in JavaScript
1. First, include the necessary Bootstrap CSS by adding the provided CDN link within your HTML file’s head section.
- <link rel='stylesheet' href='https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css'>
2. Create the HTML structure for your counter using divs, buttons, and an initial count of 0.
- <div class="container">
- <div class="col-md-3">
- <div class="jumbotron text-center">
- <h1 id="counter">0</h1>
- </div>
- <div class="row">
- <div class="col-md-6">
- <button class="btn btn-danger form-control" onclick="inc()">+</button>
- </div>
- <div class="col-md-6">
- <button class="btn btn-primary form-control" onclick="dec()">-</button>
- </div>
- </div>
- </div>
- </div>
3. The CSS code is optional but enhances the counter’s appearance. It adds some padding to the top of the page. You can customize the styling according to your design preferences.
- body{
- padding-top:50px;
- }
4. Finally, let’s create the JavaScript functions to handle the increment and decrement actions. These functions manipulate the displayed counter value and ensure it doesn’t go below zero.
- function inc(){
- counter.innerHTML = parseInt(counter.innerHTML)+1;
- }
- function dec(){
- if(parseInt(counter.innerHTML)>0)
- counter.innerHTML = parseInt(counter.innerHTML)-1;
- }
- var counter = document.getElementById("counter");
That’s all! hopefully, you have successfully created an increment and decrement counter in JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
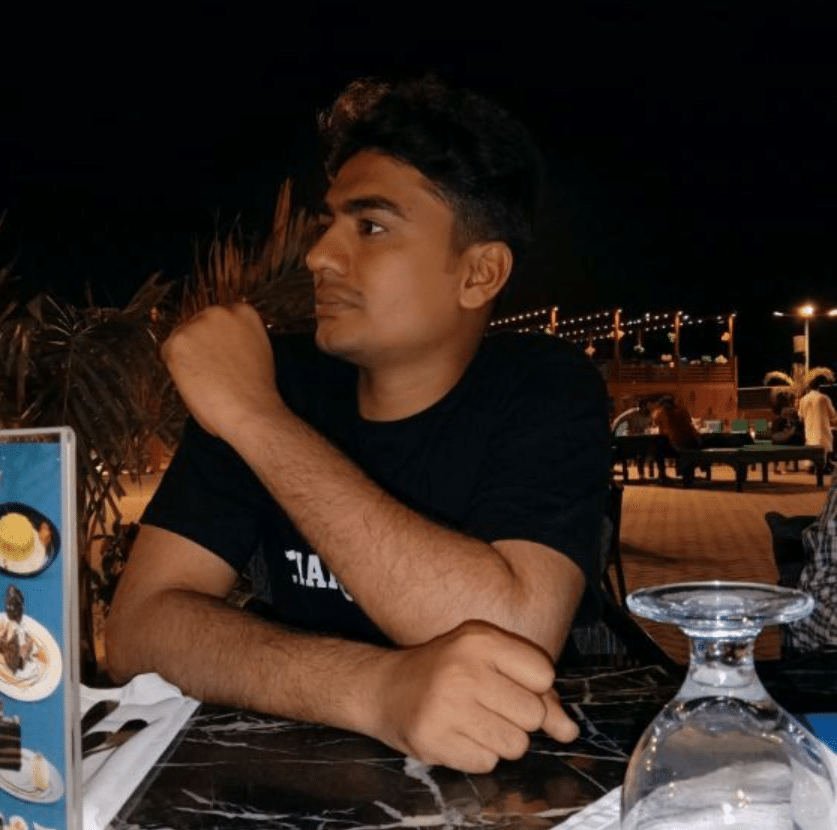
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.