This code implements a 3 Dots Dropdown Menu using HTML, CSS, and JavaScript. It creates a button with three dots, and when clicked, it displays a menu with different options. The JavaScript code handles the visibility of the menu when clicking the button. It’s helpful for adding a user-friendly dropdown menu to a web page.
Furthermore, it provides a neat and compact dropdown menu, saving space and improving the user experience. You can use this code on your website to enhance user interaction.
How to Create 3 Dots Dropdown Menu in HTML CSS
1. First of all, load the Normalize CSS by adding the following CDN link into the head tag of your HTML document.
- <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/5.0.0/normalize.min.css">
2. In your HTML file, create a container div that will hold the dropdown button and menu. Inside this container, add a button element with the class "more-btn"
and three spans with the class "more-dot"
. These dots represent the menu trigger.
- <div class="container">
- <div class="more">
- <button id="more-btn" class="more-btn">
- <span class="more-dot"></span>
- <span class="more-dot"></span>
- <span class="more-dot"></span>
- </button>
- <div class="more-menu">
- <div class="more-menu-caret">
- <div class="more-menu-caret-outer"></div>
- <div class="more-menu-caret-inner"></div>
- </div>
- <ul class="more-menu-items" tabindex="-1" role="menu" aria-labelledby="more-btn" aria-hidden="true">
- <li class="more-menu-item" role="presentation">
- <button type="button" class="more-menu-btn" role="menuitem">Share</button>
- </li>
- <li class="more-menu-item" role="presentation">
- <button type="button" class="more-menu-btn" role="menuitem">Copy</button>
- </li>
- <li class="more-menu-item" role="presentation">
- <button type="button" class="more-menu-btn" role="menuitem">Embed</button>
- </li>
- <li class="more-menu-item" role="presentation">
- <button type="button" class="more-menu-btn" role="menuitem">Block</button>
- </li>
- <li class="more-menu-item" role="presentation">
- <button type="button" class="more-menu-btn" role="menuitem">Report</button>
- </li>
- </ul>
- </div>
- </div>
- </div>
Modify the menu items to suit your specific needs. You can change the text and functionality of each button by editing the HTML within the <ul class="more-menu-items">
element.
3. Now, add the following CSS to your project to customize the appearance of the dots menu. The following CSS code includes styles for the buttons, dots, and dropdown menu.
- /* Page */
- .cd__main{
- position: relative;
- min-height: 640px;
- }
- .container {
- position: absolute;
- top: 50%;
- left: 50%;
- margin-right: -50%;
- transform: translate(-50%, -50%);
- text-align: center;
- }
- .more-menu {
- width: 100px;
- }
- /* More Button / Dropdown Menu */
- .more-btn,
- .more-menu-btn {
- background: none;
- border: 0 none;
- line-height: normal;
- overflow: visible;
- -webkit-user-select: none;
- -moz-user-select: none;
- -ms-user-select: none;
- width: 100%;
- text-align: left;
- outline: none;
- cursor: pointer;
- }
- .more-dot {
- background-color: #aab8c2;
- margin: 0 auto;
- display: inline-block;
- width: 7px;
- height: 7px;
- margin-right: 1px;
- border-radius: 50%;
- transition: background-color 0.3s;
- }
- .more-menu {
- position: absolute;
- top: 100%;
- z-index: 900;
- float: left;
- padding: 10px 0;
- margin-top: 9px;
- background-color: #fff;
- border: 1px solid #ccd8e0;
- border-radius: 4px;
- box-shadow: 1px 1px 3px rgba(0,0,0,0.25);
- opacity: 0;
- transform: translate(0, 15px) scale(.95);
- transition: transform 0.1s ease-out, opacity 0.1s ease-out;
- pointer-events: none;
- }
- .more-menu-caret {
- position: absolute;
- top: -10px;
- left: 12px;
- width: 18px;
- height: 10px;
- float: left;
- overflow: hidden;
- }
- .more-menu-caret-outer,
- .more-menu-caret-inner {
- position: absolute;
- display: inline-block;
- margin-left: -1px;
- font-size: 0;
- line-height: 1;
- }
- .more-menu-caret-outer {
- border-bottom: 10px solid #c1d0da;
- border-left: 10px solid transparent;
- border-right: 10px solid transparent;
- height: auto;
- left: 0;
- top: 0;
- width: auto;
- }
- .more-menu-caret-inner {
- top: 1px;
- left: 1px;
- border-left: 9px solid transparent;
- border-right: 9px solid transparent;
- border-bottom: 9px solid #fff;
- }
- .more-menu-items {
- margin: 0;
- list-style: none;
- padding: 0;
- }
- .more-menu-item {
- display: block;
- }
- .more-menu-btn {
- min-width: 100%;
- color: #66757f;
- cursor: pointer;
- display: block;
- font-size: 13px;
- line-height: 18px;
- padding: 5px 20px;
- position: relative;
- white-space: nowrap;
- }
- .more-menu-item:hover {
- background-color: #489fe5;
- }
- .more-menu-item:hover .more-menu-btn {
- color: #fff;
- }
- .more-btn:hover .more-dot,
- .show-more-menu .more-dot {
- background-color: #516471;
- }
- .show-more-menu .more-menu {
- opacity: 1;
- transform: translate(0, 0) scale(1);
- pointer-events: auto;
- }
4. Finally, include the following JavaScript code to handle the menu’s functionality. This code listens for a click on the button and toggles the menu’s visibility.
- var el = document.querySelector('.more');
- var btn = el.querySelector('.more-btn');
- var menu = el.querySelector('.more-menu');
- var visible = false;
- function showMenu(e) {
- e.preventDefault();
- if (!visible) {
- visible = true;
- el.classList.add('show-more-menu');
- menu.setAttribute('aria-hidden', false);
- document.addEventListener('mousedown', hideMenu, false);
- }
- }
- function hideMenu(e) {
- if (btn.contains(e.target)) {
- return;
- }
- if (visible) {
- visible = false;
- el.classList.remove('show-more-menu');
- menu.setAttribute('aria-hidden', true);
- document.removeEventListener('mousedown', hideMenu);
- }
- }
- btn.addEventListener('click', showMenu, false);
That’s all! hopefully, you have successfully created the 3 Dots Dropdown Menu. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
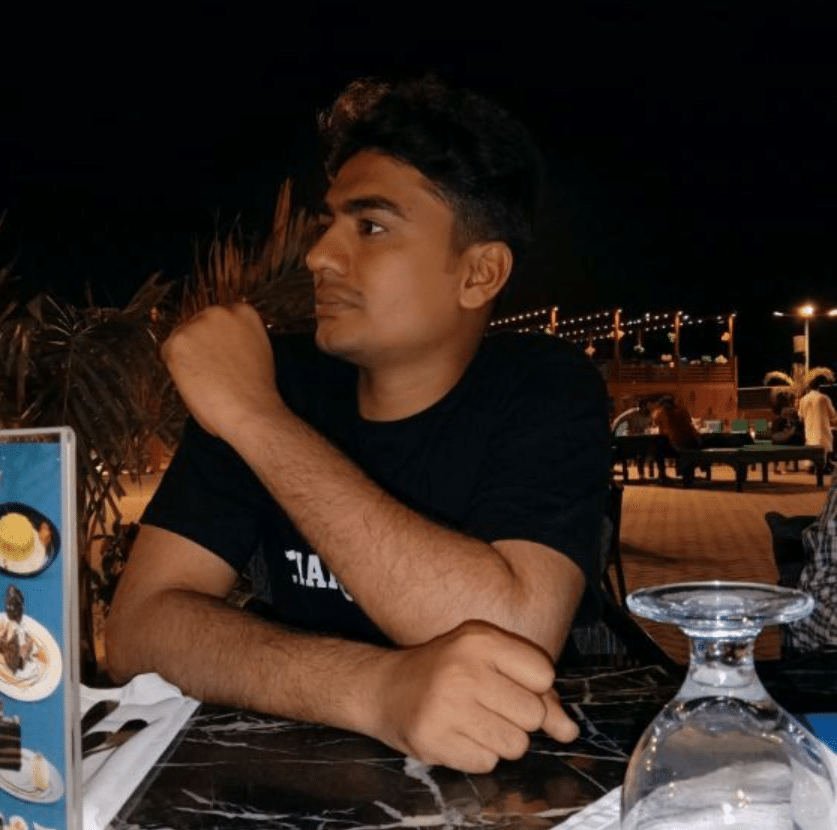
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
añadí esta parte en elcodigo para que funcionen los botones:
function hideMenu(e) {
if (btn.contains(e.target)) {
return;
}
if (menu.contains(e.target)) {
return;
}
if (visible) {
visible = false;
el.classList.remove(‘show-more-menu’);
menu.setAttribute(‘aria-hidden’, true);
document.removeEventListener(‘mousedown’, hideMenu);
}
}