The FlexSlider is highly customizable plugin for jQuery to create all kind of carousel/slider on a website. This plugin allows you to create a responsive thumbnail slider, content/text, video slider and image slider with caption, etc. It comes with multiple configuration options to customize each functionality of the slider.
Moreover, the FlexSlider supports touch, keyboard navigation and mousewheel to slide items. Besides this, it also supports CSS3 animations and HTML5 videos in sliding items.
Plugin Overview and Preview
Plugin: | FlexSlider |
Author: | WooCommerce |
Category: | Carousel |
Published: | January 20, 2024 |
File Type: | zip archive (HTML, CSS, Images & JavaScript ) |
Package Size: | File not found! |
Dependencies: | jQuery 1.7.0 or Latest version |
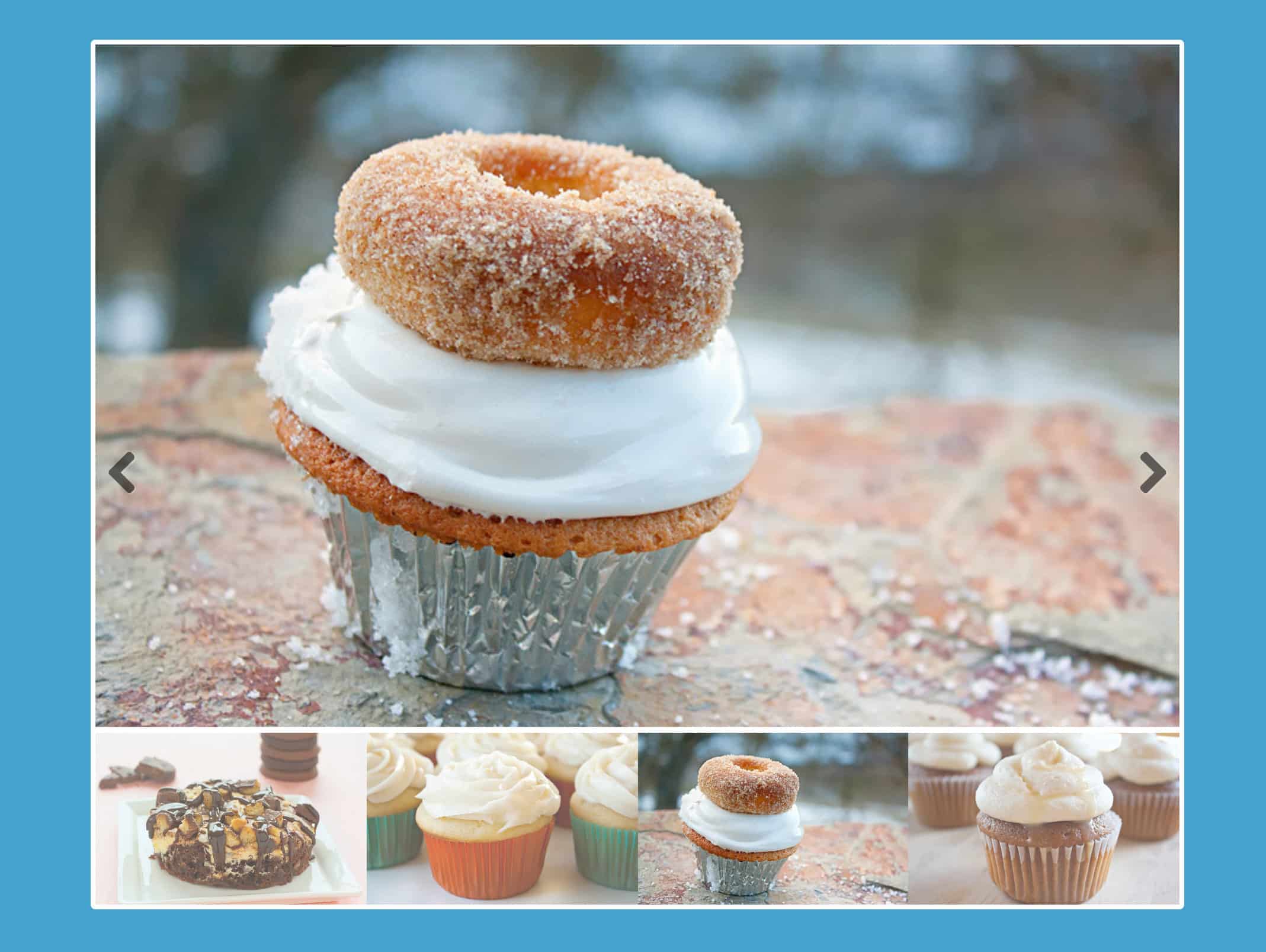
How to Create a Responsive Thumbnail Slider
1. To create “responsive thumbnail slider”, we need to getting started with FlexSlider. So, load the (minefield version of) jQuery into your website.
- <!-- jQuery -->
- <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
2. Similarly, include the FlexSlider’s CSS and JavaScript file in your website/app.
- <!-- FlexSlider CSS -->
- <link rel="stylesheet" href="css/flexslider.css">
- <!-- FlexSlider JS -->
- <script src="js/jquery.flexslider.js"></script>
3. Now, create a div
element with id “slider” and place your main images in it. Likewise, create another div
with id “carousel” and place your thumbnails links in it.
- <section class="slider">
- <div id="slider" class="flexslider">
- <ul class="slides">
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- </ul>
- </div>
- <div id="carousel" class="flexslider">
- <ul class="slides">
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_cheesecake_brownie.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_lemon.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_donut.jpg" />
- </li>
- <li>
- <img src="images/kitchen_adventurer_caramel.jpg" />
- </li>
- </ul>
- </div>
- </section>
4. When all was done, initialize the plugin (with following configurations) in jQuery document ready function.
- $(document).ready(function(){
- $('#carousel').flexslider({
- animation: "slide",
- controlNav: false,
- animationLoop: false,
- slideshow: false,
- itemWidth: 210,
- itemMargin: 5,
- asNavFor: '#slider'
- });
- $('#slider').flexslider({
- animation: "slide",
- controlNav: false,
- animationLoop: false,
- slideshow: false,
- sync: "#carousel",
- start: function(slider){
- $('body').removeClass('loading');
- }
- });
- });
FlexSlider Advance Configuration Options
The following are some advanced configuration options to create / customize responsive thumbnail slider.
Option | Default | Type | Description |
---|---|---|---|
slideshow | true | Boolean |
Enable/disable slideshow (autoplay) functionality. Example: $('#slider').flexslider({ slideshow : true, }); |
animation | “fade” | String |
Define an animation for sliding items. Possible options are: “fade” or “slide”. Example: $('#slider').flexslider({ animation : "fade", }); |
direction | “horizontal” | String |
This option define the direction for sliding items. The possible options are: “horizontal” and “vertical”. Example: $('#slider').flexslider({ direction : "horizontal", }); |
reverse | false | Boolean |
Decide whether to reverse animation direction. Example: $('#slider').flexslider({ reverse : false, }); |
animationLoop | true | Boolean |
Enable/disable loop for animations. Example: $('#slider').flexslider({ animationLoop : true, }); |
slideshowSpeed | 7000 | Number |
Define the speed for slideshow in milliseconds. Example: $('#slider').flexslider({ slideshowSpeed : 7000, }); |
animationSpeed | 600 | Number |
It define the speed for animation in milliseconds. Example: $('#slider').flexslider({ animationSpeed : 600, }); |
initDelay | 0 | Number |
Generally, this option define the initialization delay. Example: $('#slider').flexslider({ initDelay : 0, }); |
randomize | false | Boolean |
If you want to show your image in random order, then this option is useful. Example: $('#slider').flexslider({ randomize : false, }); |
pauseOnAction | true | Boolean |
This option pause the slider when a user interact with controls. Example: $('#slider').flexslider({ pauseOnAction : true, }); |
pauseOnHover | false | Boolean |
Decide whether to pause slideshow on hover. Example: $('#slider').flexslider({ pauseOnHover : false, }); |
useCSS | true | Boolean |
Lets slider to use CSS3 transitions if available. Example: $('#slider').flexslider({ useCSS : true, }); |
touch | true | Boolean |
Enable/disable touch for slider. Example: $('#slider').flexslider({ touch : true, }); |
video | false | Boolean |
If you placed some videos in the slider, turn this option to true. Example: $('#slider').flexslider({ video : false, }); |
prevText | “Previous” | String |
Define the text for previous slide button. Example: $('#slider').flexslider({ prevText : "Previous", }); |
nextText | “Next” | String |
It define the text for next slide button. Example: $('#slider').flexslider({ nextText : "Next", }); |
keyboard | true | Boolean |
Enable/disable keyboard navigation. Example: $('#slider').flexslider({ keyboard : true, }); |
mousewheel | false | Boolean |
If you want to scroll item with mousewheel, turn this option to true. It requires jquery.mousewheel.js plugin. Example: $('#slider').flexslider({ mousewheel : false, }); |
sync | “” | String |
Mirror the action performed on this slider to another. Pass the selector in this option. Example: $('#slider').flexslider({ sync : "", }); |
itemWidth | 0 | Number |
It define the with for carousel item. Example: $('#slider').flexslider({ itemWidth : 0, }); |
itemMargin | 0 | Number |
Set the margin for carousel item. Example: $('#slider').flexslider({ itemMargin : 0, }); |
minItems | 0 | Number |
The minimum number to visible in the carousel. Example: $('#slider').flexslider({ minItems : 0, }); |
maxItems | 0 | Number |
The maximum number of item visible in the carousel. Example: $('#slider').flexslider({ maxItems : 0, }); |
move | 0 | Number |
It define the number of carousel item should move on animation. Example: $('#slider').flexslider({ move : 0, }); |
Flexslider Callback Functions
The following are some options to excute custom callback functions.
- start: function(){},
- before: function(){},
- after: function(){},
- end: function(){},
- added: function(){},
- removed: function(){}
Similar Code Snippets:
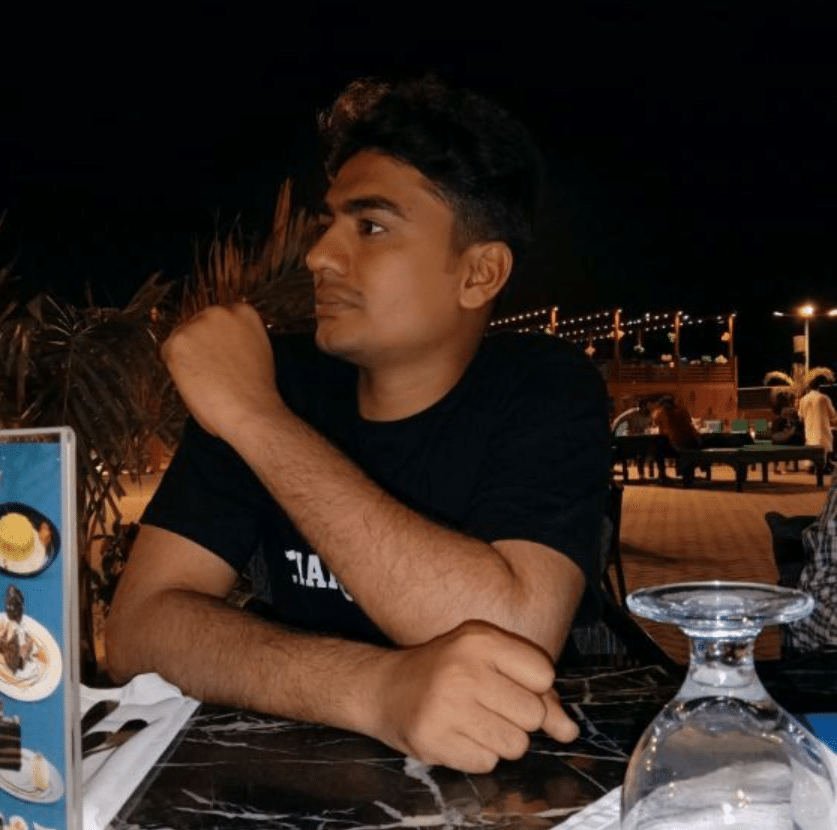
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.