Do you want to create a simple login form using Bootstrap? Yes! here is a step by step guide for creating a basic login form. You can also download this project directly to implement this form into your project.
How to Create a Simple Login Form using Bootstrap
1. First of all, load the Bootstrap framework into your HTML page.
- <!-- Bootstrap CSS -->
- <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
- <!-- Bootstrap JS -->
- <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
2. After that, create an HTML structure for your login form as follows:
- <div class="wrapper">
- <form class="form-signin">
- <h2 class="form-signin-heading">Please login</h2>
- <input type="text" class="form-control" name="username" placeholder="Email Address" required="" autofocus="" />
- <input type="password" class="form-control" name="password" placeholder="Password" required=""/>
- <label class="checkbox">
- <input type="checkbox" value="remember-me" id="rememberMe" name="rememberMe"> Remember me
- </label>
- <button class="btn btn-lg btn-primary btn-block" type="submit">Login</button>
- </form>
- </div>
3. At last, style your login form with the following CSS.
- .wrapper {
- margin-top: 80px;
- margin-bottom: 80px;
- }
- .form-signin {
- max-width: 380px;
- padding: 15px 35px 45px;
- margin: 0 auto;
- background-color: #fff;
- border: 1px solid rgba(0, 0, 0, 0.1);
- }
- .form-signin .form-signin-heading,
- .form-signin .checkbox {
- margin-bottom: 30px;
- }
- .form-signin .checkbox {
- font-weight: normal;
- }
- .form-signin .form-control {
- position: relative;
- font-size: 16px;
- height: auto;
- padding: 10px;
- -webkit-box-sizing: border-box;
- -moz-box-sizing: border-box;
- box-sizing: border-box;
- }
- .form-signin .form-control:focus {
- z-index: 2;
- }
- .form-signin input[type="text"] {
- margin-bottom: -1px;
- border-bottom-left-radius: 0;
- border-bottom-right-radius: 0;
- }
- .form-signin input[type="password"] {
- margin-bottom: 20px;
- border-top-left-radius: 0;
- border-top-right-radius: 0;
- }
You have all done! Feel free to customize this login form according to your needs. If you need any further help, let me know by comment below.
Similar Code Snippets:
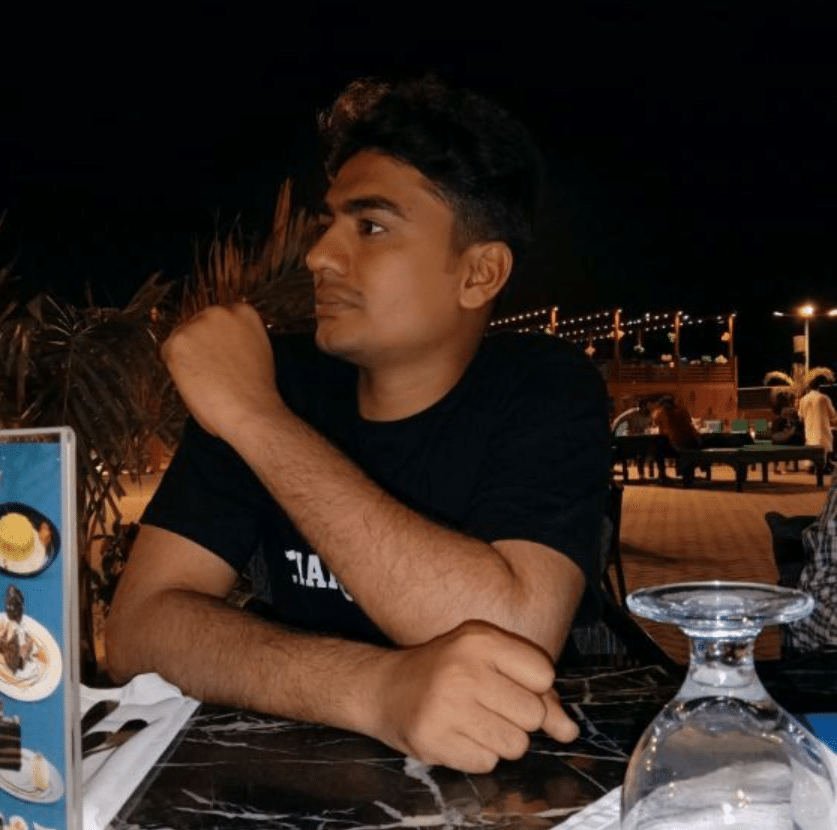
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences.
I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.